ArrayList vs LinkedList: Which One Should You Choose?
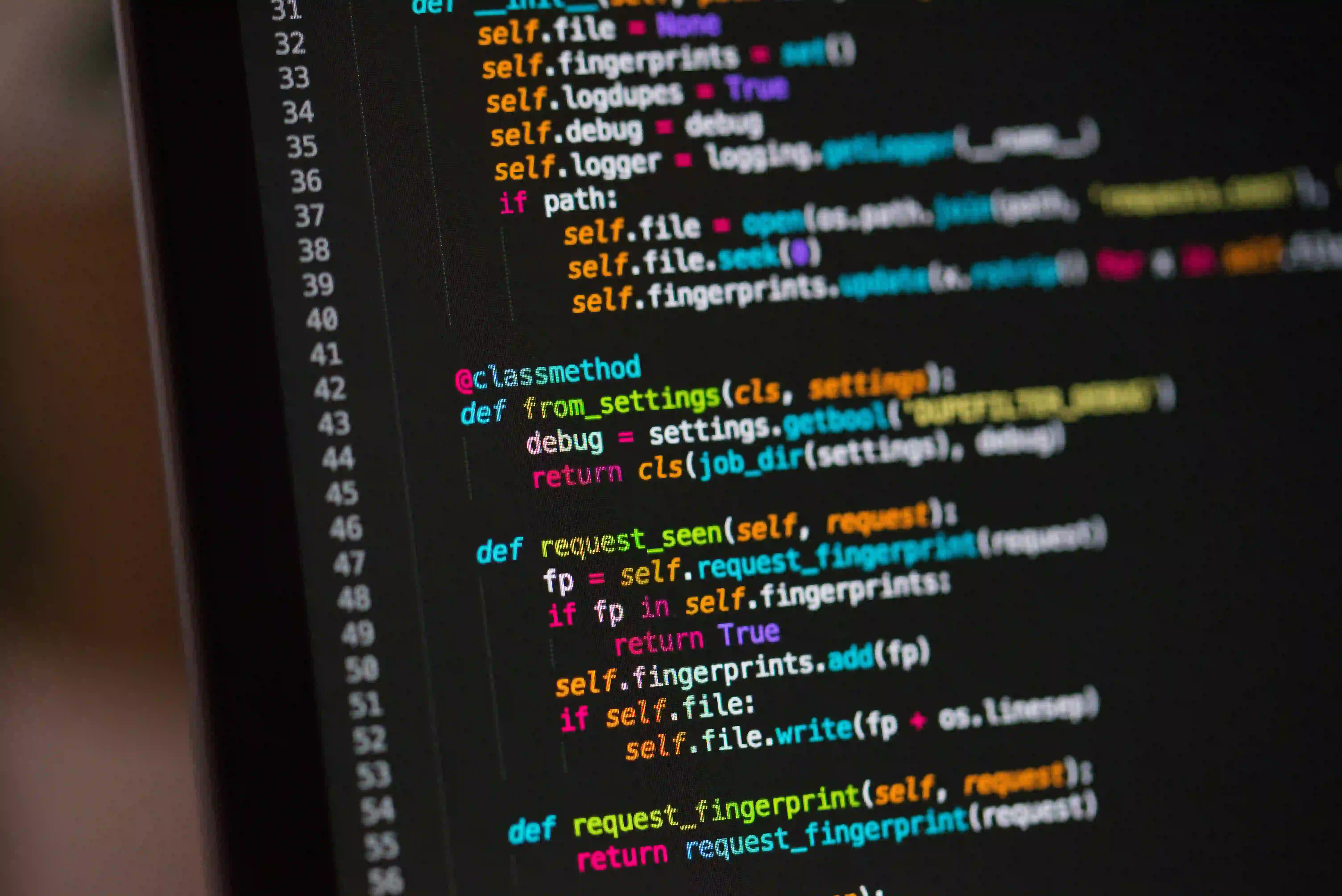
ArrayList vs LinkedList: Which One Should You Choose?
Data structures are fundamental to programming, and choosing the right one can significantly impact the performance and efficiency of your application. In this article, we delve into two of Java's most well-known collections: ArrayList
and LinkedList
. We will explore their differences, use cases, and performance considerations, providing you with a comprehensive understanding to help inform your choice.
Understanding ArrayList and LinkedList
In Java, both ArrayList
and LinkedList
are part of the Java Collections Framework. They both implement the List
interface, meaning they allow you to store an ordered collection of elements. However, their underlying implementations are quite different.
ArrayList
An ArrayList
is backed by a dynamic array. When you create an ArrayList
, it allocates a specific size for the array. If this size is exceeded during the addition of elements, a new, larger array is allocated, and the old elements are copied over.
Key Characteristics of ArrayList:
- Access Speed: O(1) for accessing elements since it uses a dynamic array.
- Insertion Speed: O(n) if adding elements anywhere except at the end (where it will be O(1) if there is enough capacity).
- Space Efficiency: Utilizes more memory due to underlying array allocation.
- Iteration Speed: Generally faster due to better cache locality, making it more performant in terms of iteration.
LinkedList
A LinkedList
, on the other hand, is comprised of a series of nodes. Each node stores the data and references to the next (and optionally previous) node in the sequence. This structure allows for dynamic resizing without the need for array reallocation.
Key Characteristics of LinkedList:
- Access Speed: O(n) as you may need to traverse the list to find an element.
- Insertion Speed: O(1) for adding or removing elements (especially at the beginning or end).
- Space Efficiency: Each element needs additional memory for pointers (or references), leading to a higher memory overhead.
- Iteration Speed: Slower than
ArrayList
due to the non-contiguous nature of the memory allocation.
When to Use Each
Use ArrayList
When:
- Your primary operation involves indexing and accessing elements.
- The number of elements is stable and does not change frequently.
- You require better performance for iterations.
- You prefer less memory overhead for simple data storage.
Example of Using ArrayList:
import java.util.ArrayList;
public class ArrayListExample {
public static void main(String[] args) {
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
// Accessing an item by index
String myFruit = fruits.get(1); // O(1) Access
System.out.println("Fruit at index 1: " + myFruit); // Outputs: Banana
}
}
In this example, getting the element at index 1 is fast (O(1)), thanks to the underlying array structure of ArrayList
.
Use LinkedList
When:
- You frequently add or remove elements from the beginning or end of the list.
- Your operations often involve inserting or deleting elements at arbitrary positions.
- You require a large number of insertions and deletions.
- The list’s size is highly dynamic and unpredictable.
Example of Using LinkedList:
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
LinkedList<String> animals = new LinkedList<>();
animals.add("Lion");
animals.add("Tiger");
animals.add("Bear");
// Adding an element at the beginning
animals.addFirst("Elephant"); // O(1) Insertion
System.out.println("First animal: " + animals.get(0)); // Outputs: Elephant
}
}
Here, adding an element at the beginning is efficient (O(1)), showcasing the strength of the LinkedList
when managing dynamic data.
Performance Comparison
To better compare ArrayList
and LinkedList
, let’s summarize their performance in terms of time complexity:
| Operation | ArrayList | LinkedList | |-------------------------|----------------|-----------------| | Access | O(1) | O(n) | | Search | O(n) | O(n) | | Insertion at End | O(1) on average| O(1) | | Insertion at Start/Index| O(n) | O(1) | | Deletion at End | O(1) | O(1) | | Deletion at Start/Index | O(n) | O(1) |
Potential Pitfalls
While choosing between ArrayList
and LinkedList
, be aware of their implications on memory usage and performance:
- Memory Overhead: Each node in a
LinkedList
incurs additional memory for storage of pointer/reference. This is particularly significant when dealing with large amounts of data. - Cache Performance: Due to contiguous memory allocation,
ArrayList
can often provide better cache performance, making it more efficient for iteration operations.
Wrapping Up
In conclusion, the choice between ArrayList
and LinkedList
fundamentally hinges on the intended use case. If you require fast access to elements with infrequent insertions and deletions, ArrayList
is your best bet. Conversely, if you need to perform many insertions and deletions, especially at both the beginning and end of your list, consider using LinkedList
.
Ultimately, there is no one-size-fits-all answer; the right option depends on the specific context of your application.
For further reading, consider checking out the official Java Documentation on ArrayList
and LinkedList for deeper insights into their methods and behaviors.
By understanding the differences and implications of each, you can make an informed decision and optimize your Java applications effectively. Happy coding!