Troubleshooting Early Look Features in Java 11
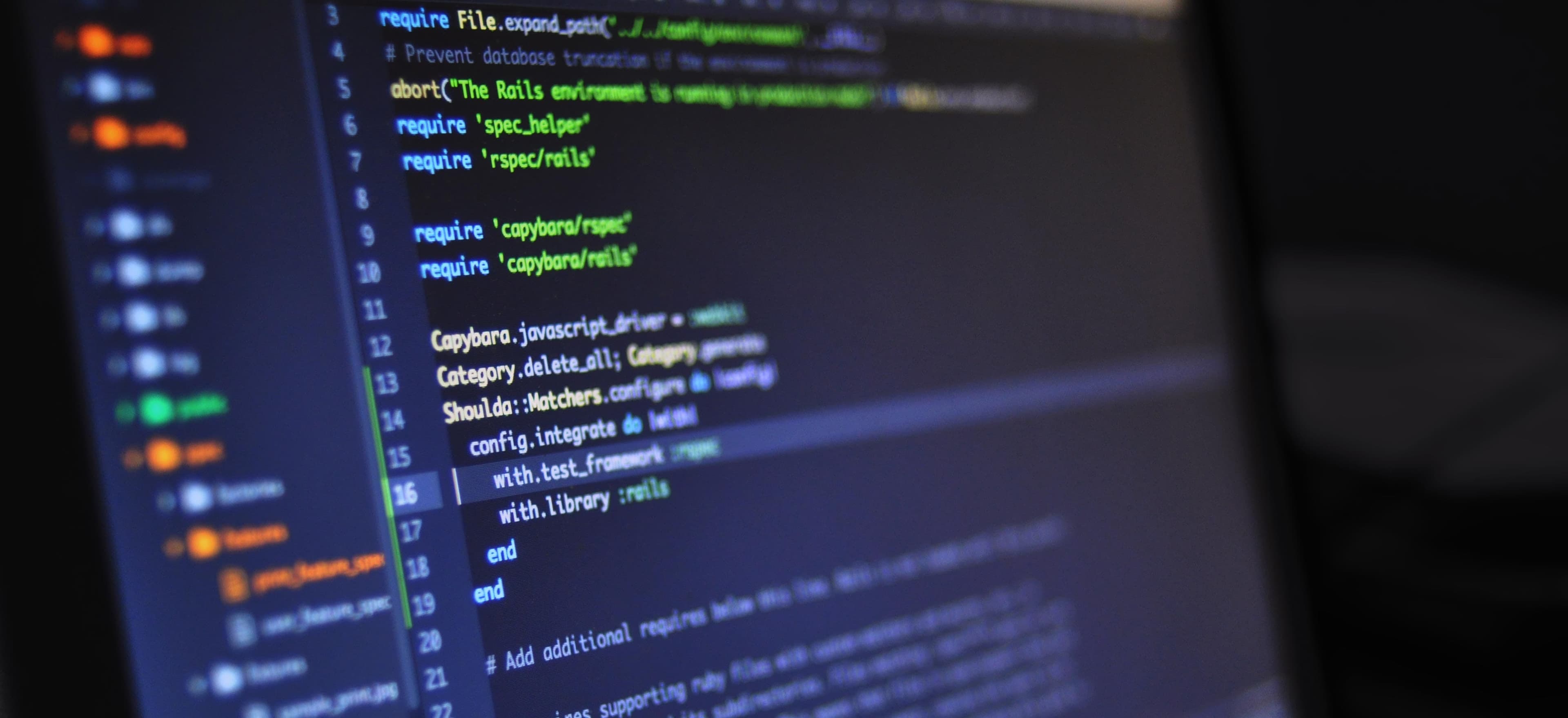
- Published on
Troubleshooting Early Look Features in Java 11
Java 11, a long-term support (LTS) version, brought a treasure trove of features that developers can leverage to create efficient and maintainable applications. However, with such additions come challenges, especially when it comes to early look features. These features, while promising, can also lead to confusion or unexpected behavior during implementation.
In this blog post, we will analyze the most common troubleshooting aspects related to early look features in Java 11. We will delve into examples, explanations, and best practices to help you navigate through any issues you encounter. Let's get started.
Understanding Early Look Features
Before diving into troubleshooting, it’s crucial to grasp what early look features are. Early look features (also known as preview features) are experimental capabilities introduced in a Java version but not yet finalized. They allow developers to test and provide feedback to the Java community.
In Java 11, the key early look features include:
Local-Variable Syntax for Lambda Parameters
Var Handles
Although these features introduce significant enhancements, developers may face issues due to their experimental nature. At times, a feature might not work as expected or may have limitations. This post will help recognize and fix common problems.
Local-Variable Syntax for Lambda Parameters
One of the most notable features is the ability to use the var
keyword for lambda parameters. This allows for type inference, leading to more concise and clearer code.
Example Code
import java.util.List;
public class LambdaExample {
public static void printNumbers(List<Integer> numbers) {
numbers.forEach((var number) -> {
System.out.println("Number: " + number);
});
}
public static void main(String[] args) {
List<Integer> myNumberList = List.of(1, 2, 3, 4, 5);
printNumbers(myNumberList);
}
}
Key Points
-
Why Use
var
?: Usingvar
enhances readability without compromising type safety. It allows the developer to convey the intent more clearly, as seen in the lambda expression. -
Common Issues: One common mistake is trying to mix
var
with other explicitly declared types in the same lambda. For instance:numbers.forEach((var number, String ignored) -> { System.out.println(number); });
This will lead to a compilation error because
var
cannot be mixed with other explicit types in lambda parameters. -
Solution: Stick to using
var
exclusively for all parameters in that lambda. If you need to specify types, opt for regular declarations.
Var Handles
Another prominent early look feature in Java 11 is Var Handles. These offer a way to access variables, including both static and instance fields, in a way that's more flexible than traditional reflection.
Example Code
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
public class VarHandleExample {
private int counter = 0;
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
VarHandle handle = MethodHandles.lookup().findVarHandle(VarHandleExample.class, "counter", int.class);
VarHandleExample example = new VarHandleExample();
handle.set(example, 42); // Set counter to 42
int newValue = (int) handle.get(example); // Get counter value
System.out.println("Counter: " + newValue);
}
}
Key Points
-
Why Use Var Handles?: Var Handles allow atomic operations on variables. This is especially useful in concurrent programming scenarios.
-
Common Issues: A frequent stumbling block is not using the correct access type or attempting to use Var Handles with types that are incompatible.
-
Solution: Make sure the type specified during Var Handle creation matches the actual variable type. For instance, trying to use an
int
Var Handle to access a field of typedouble
will lead to a runtime error. -
Additional Reading: For an in-depth understanding, refer to the Java VarHandle Documentation.
General Troubleshooting Tips
-
Check Compatibility: Make sure you are using Java 11 correctly. It is imperative to ensure your development environment is set up correctly to avoid issues with these early look features.
-
Read the Documentation: Many issues stem from misunderstandings about how a feature should work. The Java SE 11 Documentation can be invaluable.
-
Debugging: Use a debugger to step through the code. Part of troubleshooting involves observing how variables change as the code executes.
-
Community Resources: Don’t hesitate to turn to forums, such as Stack Overflow or the Java subreddit, to seek help from other developers who've experienced similar issues.
-
Keep Learning: Features evolve, and so does the Java language. Be open to learning, whether through formal classes, tutorials, or community discussions.
Wrapping Up
Utilizing early look features in Java 11 can significantly enhance your code's conciseness and performance. However, it’s vital to approach these features with a mindset attuned to potential issues.
This post highlighted the key troubleshooting aspects of the Local-Variable Syntax for Lambda Parameters
and Var Handles
. By following best practices and utilizing the available resources, you can navigate through and resolve any challenges that may arise.
As with any evolving technology, staying informed will empower you to take advantage of new features while minimizing hurdles. Happy coding!
For more information on Java's evolving features, consider subscribing to the OpenJDK mailing list for insights and updates from the community.
This SEO-optimized blog post is designed to help you not only troubleshoot effectively but also expand your understanding of Java 11’s early look features. If you found this useful, feel free to share or leave a comment!
Checkout our other articles