Common JVM Issues and How to Resolve Them Quickly
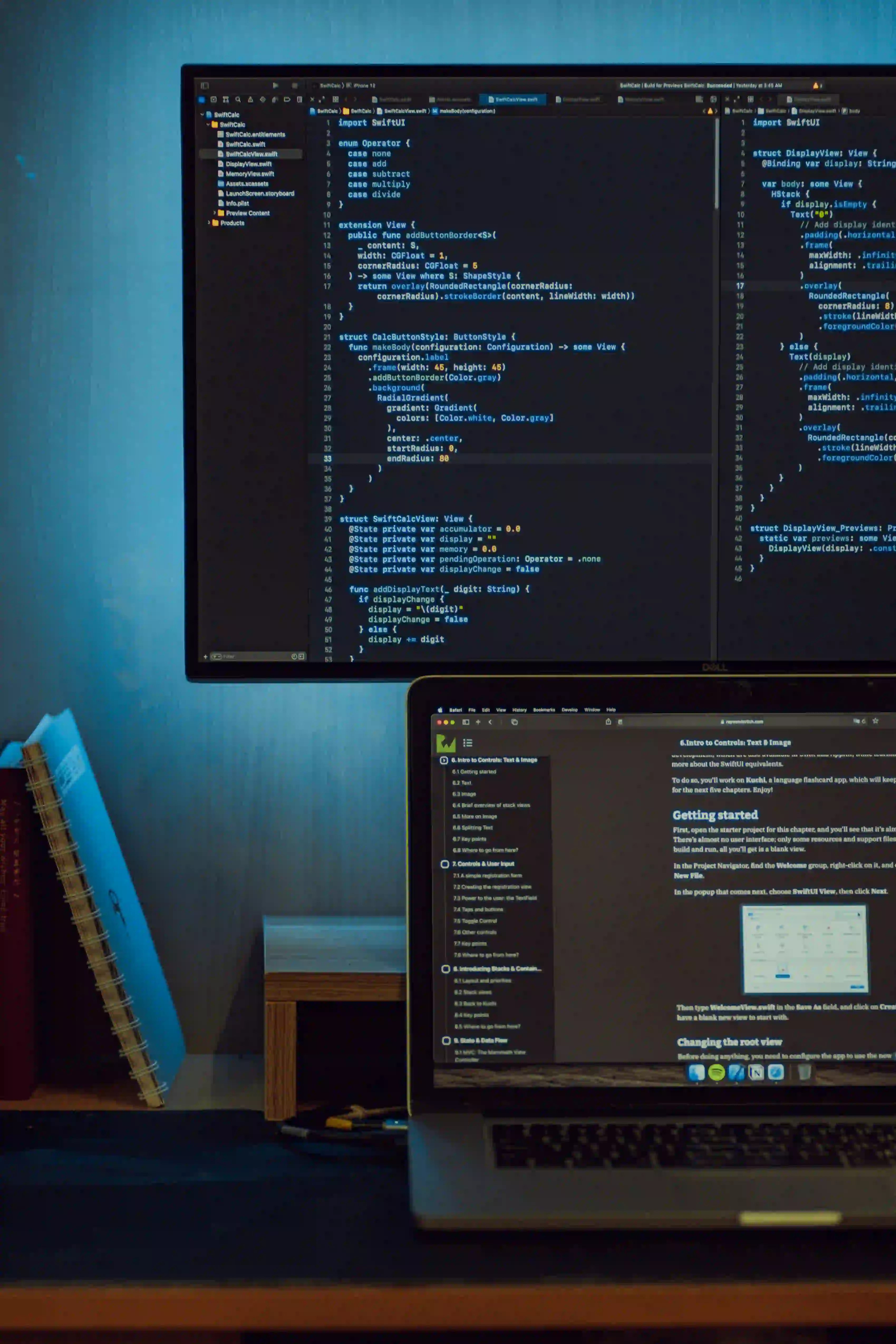
Common JVM Issues and How to Resolve Them Quickly
The Java Virtual Machine (JVM) is an essential, multi-faceted component of the Java programming language. It serves both as a lightweight platform that interprets Java bytecode and as a powerful engine optimizing application performance. While the JVM generally operates smoothly, developers often encounter various issues that can affect the efficiency and reliability of Java applications. In this blog post, we'll discuss some common JVM issues and provide strategies for quickly resolving them.
Table of Contents
- Memory Leaks
- OutOfMemoryError
- Garbage Collection Issues
- Threading Problems
- Class Loading Issues
- Native Memory Issues
- Conclusion
- Additional Resources
1. Memory Leaks
What are Memory Leaks?
A memory leak occurs when objects in memory are unintentionally referenced by other objects, preventing them from being garbage-collected. Over time, memory usage increases, which may lead to performance degradation.
How to Resolve
Use Profiling Tools: Use tools like VisualVM or Eclipse Memory Analyzer (MAT) to identify memory leaks.
Example: Below is an example of how to analyze memory usage:
// Sample Java code demonstrating a potential memory leak
import java.util.HashMap;
import java.util.Map;
public class MemoryLeakDemo {
private static Map<String, String> cache = new HashMap<>();
public static void main(String[] args) {
while (true) {
cache.put(String.valueOf(System.currentTimeMillis()), "Value");
}
}
}
Why?: In this code, the cache
map keeps growing indefinitely, as new entries are added without ever being removed. A profiling tool will make it easier to spot that cache
holds onto more data than it should.
2. OutOfMemoryError
What is OutOfMemoryError?
java.lang.OutOfMemoryError
occurs when the JVM cannot allocate an object due to lack of memory. This is often a direct consequence of memory leaks and poor allocation settings.
How to Resolve
Adjust Heap Size: Modify the JVM options to allocate more heap space using -Xms
(initial size) and -Xmx
(maximum size).
java -Xms512m -Xmx2048m -jar YourApplication.jar
Why?: Increasing heap size allows your application to consume more memory, reducing the likelihood of encountering OutOfMemoryError
.
3. Garbage Collection Issues
What are Garbage Collection Issues?
Garbage Collection (GC) issues arise when the garbage collector fails to reclaim memory efficiently, leading to long pause times or high CPU usage.
How to Resolve
Use Different GC Algorithms: Switching to a more efficient GC algorithm can improve performance. For Java 8 and later, use:
java -XX:+UseG1GC -jar YourApplication.jar
Why?: The G1 garbage collector is designed to efficiently manage heap space with minimal pause times, thus enhancing application responsiveness.
4. Threading Problems
What are Threading Problems?
Threading issues can cause performance bottlenecks or application crashes. Common threading problems include deadlocks, race conditions, and thread starvation.
How to Resolve
Use Monitoring Tools: Utilize the Java Thread Dump for profiling threads.
jstack [pid] > thread_dump.txt
Example: Code snippet for thread creation.
public class ThreadExample implements Runnable {
public void run() {
// Code that may lead to race conditions or deadlocks
}
public static void main(String[] args) {
Thread thread = new Thread(new ThreadExample());
thread.start();
}
}
Why?: Monitoring threads helps identify bottlenecks or deadlocks. Analyze the thread dump for potential issues.
5. Class Loading Issues
What are Class Loading Issues?
Class loading issues arise when the JVM cannot locate or load classes, often leading to ClassNotFoundException
.
How to Resolve
Check Classpath Settings: Ensure that the necessary JAR files are included in the classpath.
Example: Setting the classpath in a command:
java -cp .:lib/* YourMainClass
Why?: The -cp
option allows you to specify where the classes and libraries are located, ensuring the JVM can load them correctly.
6. Native Memory Issues
What are Native Memory Issues?
Native memory issues stem from memory allocation outside the JVM heap, often in native libraries, leading to excessive memory consumption.
How to Resolve
Use Native Memory Tracking (NMT): Enable NMT to troubleshoot native memory leaks.
java -XX:NativeMemoryTracking=summary -jar YourApplication.jar
Why?: NMT helps developers understand how native resources are allocated and can identify memory leaks that cannot be tracked with standard garbage collection.
7. Conclusion
In summary, the Java Virtual Machine is a robust and versatile environment for running Java applications, but it comes with its own set of challenges. From memory leaks to threading disorders, developers can face numerous issues that can slow down applications or lead to crashes. By utilizing proper diagnostic tools and strategies, JVM issues can be resolved quickly and effectively.
Additional Resources
- Java Performance: The Definitive Guide
- JVM Tuning Guide
- Understanding Garbage Collection in Java
By staying informed and anticipating potential JVM issues, Java developers can enhance their applications' performance and reliability. Feel free to explore the provided links for deeper insights and techniques tailored to JVM management.