Streamline Java POJO Creation with Eclipse Hibernate Tool
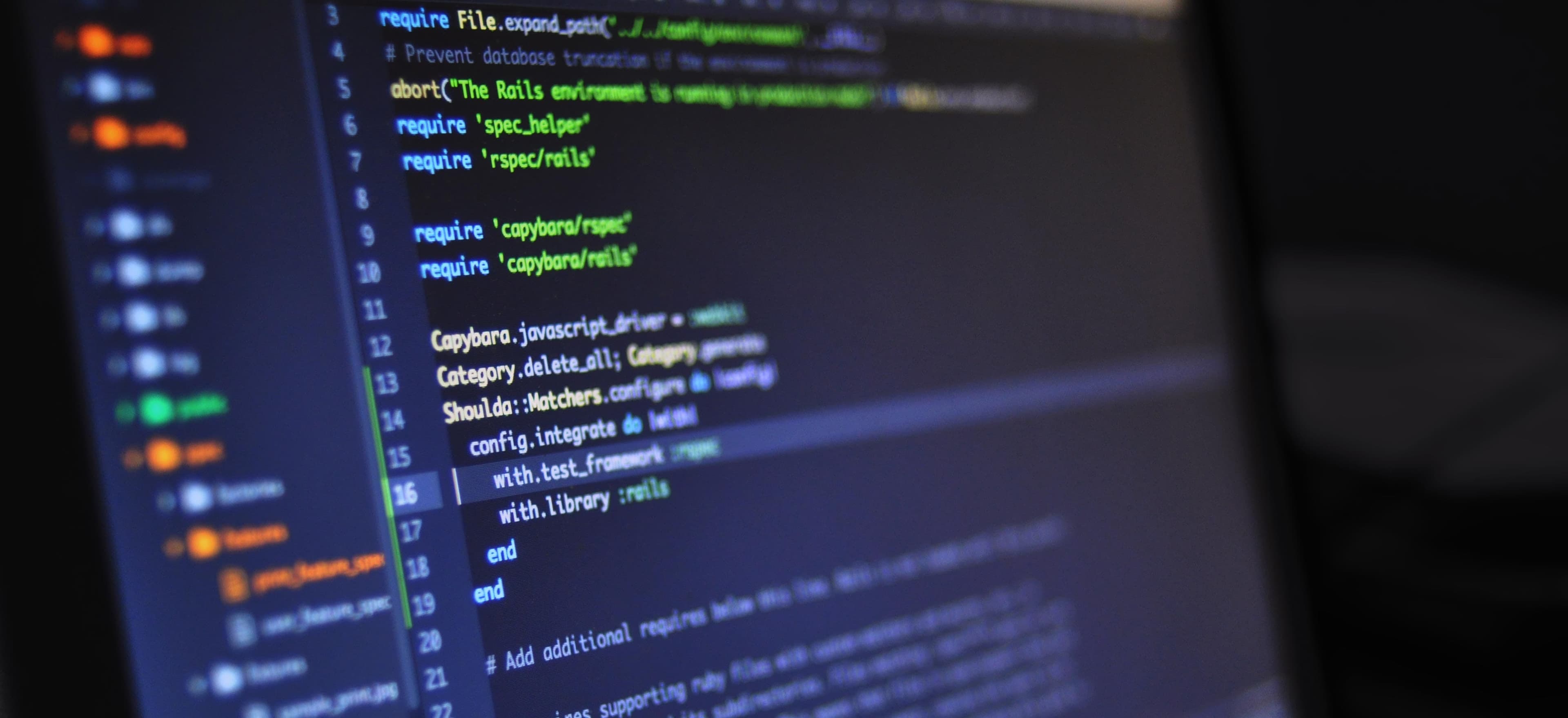
- Published on
Streamline Java POJO Creation with Eclipse Hibernate Tool
In the realm of Java development, creating Plain Old Java Objects (POJOs) is an essential task. These simple objects are primarily used to represent data, facilitating the data manipulation processes of Java applications. However, creating POJOs manually can be a repetitive and time-consuming process. Thankfully, tools such as the Eclipse Hibernate Plugin come to the rescue, allowing for an efficient and streamlined workflow.
This blog post delves into how you can leverage the Eclipse Hibernate Tool to create and manage Java POJOs with ease. By the end of this guide, you should be able to enhance your productivity, and seamlessly integrate Hibernate into your Java applications.
What are POJOs?
POJOs are the backbone of any Java application, serving as the primary means of representing data. They are simple Java objects not bound by any specific framework constraints, and can include fields, constructors, and getter/setter methods. Here’s a quick example of a simple POJO:
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
While manually creating such classes is straightforward, there are common pitfalls and repetitive tasks that can drain valuable time. This is where automation through tools like Eclipse Hibernate becomes invaluable.
Why Use Hibernate?
Hibernate is an object-relational mapping (ORM) framework for Java. It helps developers interact with a database in a more object-oriented manner, abstracting the interactions with SQL. Declaring relationships between objects and tables is simplified, making it easier to persist and retrieve data.
Advantages of Hibernate:
-
Reduced Boilerplate Code: Write less code by using APIs that handle repetitive tasks.
-
Caching: Hibernate provides built-in caching mechanisms that boost performance.
-
Automatic Schema Generation: You can auto-generate database schemas from your POJOs.
-
Database Independence: Write database-agnostic code.
With these advantages in mind, it becomes clear why integrating Hibernate into your development phase is a smart choice.
Setting Up Eclipse with Hibernate
Before diving into automating POJO creation, ensure you have the following:
-
Eclipse IDE: Ensure you have the latest version of Eclipse installed.
-
Hibernate: Download the Hibernate library and add it to your project.
-
Database Connector: Make sure to include the necessary database JDBC driver in your project.
Installing the Hibernate Tool
-
Open Eclipse.
-
Navigate to Help -> Eclipse Marketplace.
-
Search for "Hibernate" and install the Hibernate Tools plugin.
This tool will enhance your IDE by providing you the necessary functionalities to generate POJOs from your database tables.
How to Create POJOs Using Eclipse Hibernate Tool
Now that our environment is ready, let’s walk through the steps to create POJOs efficiently.
Step 1: Create a Database Connection
- Open the "Data Source Explorer" in Eclipse.
- Right-click on Database Connections -> New to create a new connection.
- Follow the prompts to connect to your database by specifying the database type, name, URL, username, and password.
Step 2: Generate POJOs from Database Tables
Once you’ve established a database connection, it’s time to generate POJOs:
- Navigate to File -> New -> Other.
- In the dialog box, search for JPA -> JPA Project, and click Next.
- Provide a project name and select a target runtime.
- On the next screen, choose your Data Source and JPA Facet version.
After you finalize the setup:
- Right-click your project in the Package Explorer.
- Select JPA Tools -> Generate Entities from Database.
- Follow the steps to specify which tables to generate your POJOs from.
Code Example: Generated POJO
Here’s an example POJO that might be generated from a user table:
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name", nullable = false)
private String name;
@Column(name = "age")
private Integer age;
// Constructors, Getters and Setters
public User() {
}
public User(String name, Integer age) {
this.name = name;
this.age = age;
}
public Long getId() {
return id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
}
Why Use Annotations?
In the above code, we leverage JPA annotations:
- @Entity: Tells Hibernate to treat this class as an entity.
- @Table: Maps the class to a specific database table.
- @Id: Denotes the primary key of the entity.
- @GeneratedValue: Specifies the strategy for primary key generation.
Using these annotations makes it easier to control aspects of POJO behavior without writing excessive configuration.
Leveraging Generated POJOs
Once POJOs are generated, they can easily be integrated into any CRUD operation. Hibernate will provide data retrieval and persistence methods, allowing you to focus more on business logic.
Example of using the generated User POJO in service layer:
public void createUser(String name, int age) {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
User user = new User(name, age);
session.save(user);
transaction.commit();
} catch (HibernateException e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
} finally {
session.close();
}
}
In this snippet, you’ve encapsulated the logic for creating a new user while allowing Hibernate to manage the database operations seamlessly.
For further reading, you may want to check the official Hibernate documentation and Spring Data JPA projects for advanced ORM concepts.
Final Thoughts
The Eclipse Hibernate Tool not only simplifies POJO creation but also significantly enhances the efficiency of your development process. By streamlining the creation of POJOs and reducing boilerplate code, developers can focus on what truly matters - building their applications.
Whether you are working on small-scale projects or larger enterprise applications, mastering tools like Hibernate will undoubtedly provide a robust foundation. With a few steps of setup, you can generate a plethora of POJOs and elevate your database interactions to new heights.
Utilize the power of Hibernate today and witness the transformation in your Java development experience!