Resolving the Invalid Target Release Error in Maven
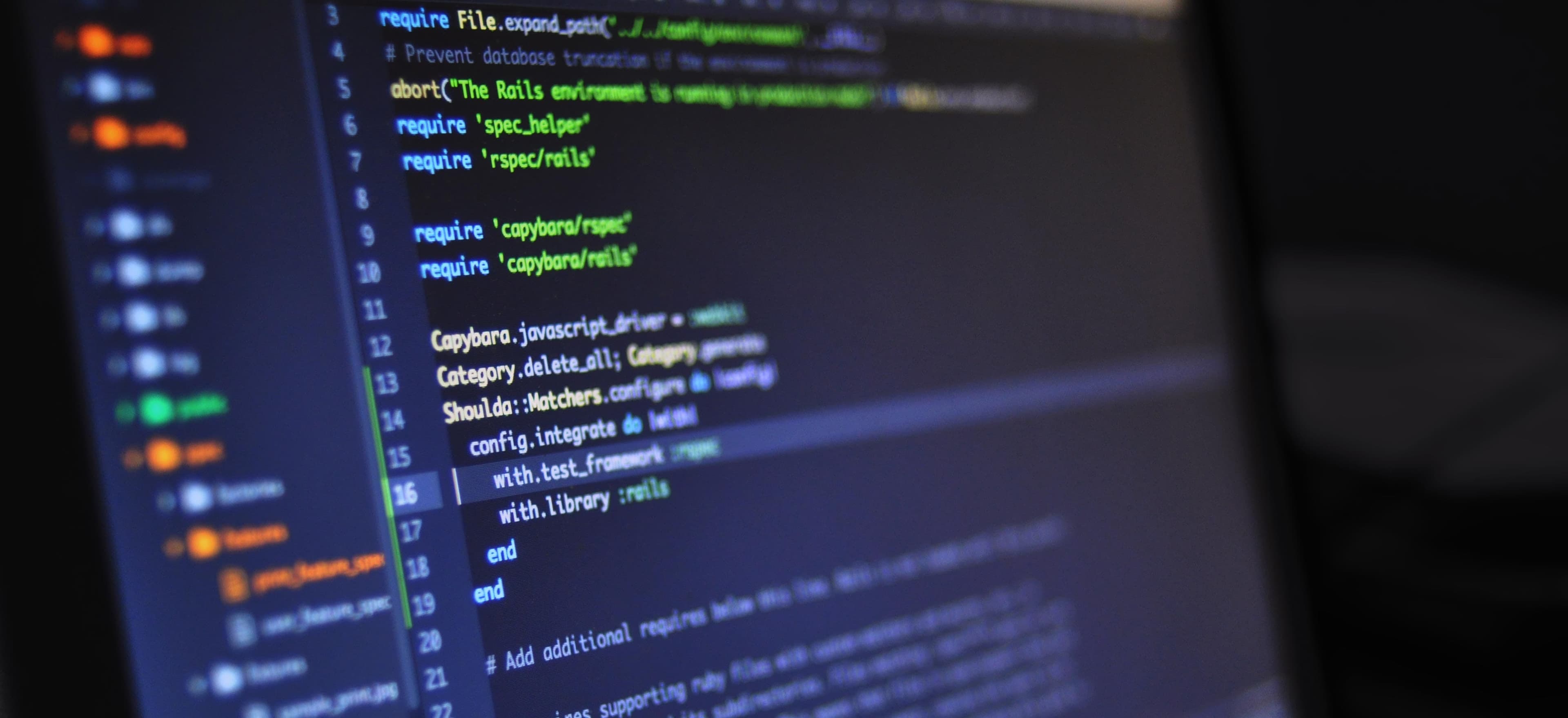
- Published on
Resolving the Invalid Target Release Error in Maven
In the realm of Java project development, Maven serves as a powerful tool for managing project dependencies, builds, and configurations. However, developers often run into a frustrating issue known as the "Invalid Target Release Error." In this blog post, we'll explore the causes of this error, provide detailed instructions on how to resolve it, and ensure your projects maintain seamless functionality.
Understanding the Invalid Target Release Error
The "Invalid Target Release Error" typically occurs when the Java Compiler cannot recognize the specified source
or target
version in the Maven configuration. This may happen due to:
- Installing an unsupported version of Java.
- Incorrectly setting the Maven Compiler Plugin configuration.
- Incompatibility between the project's Java version and the installed Java version.
Common Symptoms of the Error
Here are a few common indicators that you may encounter:
- Error messages like "Invalid target release: 11" or "Invalid target release: 1.8".
- Maven build failures when executing commands like
mvn clean install
. - Warnings during compilation indicating an inability to find the specified Java version.
Example Maven Project Configuration
Before diving into solutions, let's look at a sample pom.xml
file. This file serves as the central configuration point for a Maven project.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>17</maven.compiler.source>
<maven.compiler.target>17</maven.compiler.target>
</properties>
</project>
This example specifies the compiler version with <maven.compiler.source>
and <maven.compiler.target>
. In this case, we’re using Java 17 as the target.
Steps to Resolve the Invalid Target Release Error
Step 1: Verify Installed Java Version
Use the following command in your terminal or command prompt to check your current JVM version:
java -version
The output will look something like:
java version "17.0.1" 2021-10-19
Java(TM) SE Runtime Environment (build 17.0.1+12-39)
Java HotSpot(TM) 64-Bit Server VM (build 17.0.1+12-39, mixed mode, sharing)
Step 2: Align Java Version with Maven Compiler Plugin
Ensure that the version defined in your pom.xml
aligns with your Java installation. If you encounter an error like "Invalid target release: 17," you likely have a different version installed.
To resolve this, update the properties in your pom.xml
to match the version returned by java -version
.
For example, if the installed version is 11, adjust your properties accordingly:
<properties>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
Step 3: Update the Maven Compiler Plugin
If you are still experiencing issues, explicitly define the Maven Compiler Plugin in your pom.xml
as shown below:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>${maven.compiler.source}</source>
<target>${maven.compiler.target}</target>
</configuration>
</plugin>
</plugins>
</build>
In this snippet, we specify the plugin version (3.8.1 in this case) and use the properties set earlier for source
and target
.
Step 4: Clean and Rebuild the Project
After making these changes, ensure you perform a clean and rebuild:
mvn clean install
This command clears previous build artifacts and recompiles all source files.
Step 5: Check for Multiple JDK Installations
If the problem persists, it's worth checking if there are multiple JDK installations on your system. You can list all installed JDKs with the javac -version
command. To manage your Java versions, consider using Java version managers like SDKMAN! or JEnv.
Step 6: Environment Variables
Lastly, ensure that the JAVA_HOME
environment variable is set appropriately, pointing to a valid JDK installation. Here’s how to check your JAVA_HOME
setting:
On Windows:
echo %JAVA_HOME%
On macOS/Linux:
echo $JAVA_HOME
Ensure that this version matches the version you have in your pom.xml
.
Final Thoughts
The "Invalid Target Release Error" in Maven can be a significant hurdle in Java development, but it is certainly resolvable. By ensuring that your project configuration aligns with your Java installation, you can smooth over these bumps in the road.
By following the steps outlined in this guide, developers can get back to focusing on what they do best: writing code. If you wish to learn more about Maven and Java configurations, you can access the official Maven documentation.
Feel free to leave your comments, experiences, or questions below, and we will be glad to assist you! Happy coding!