Static vs Dynamic Analysis: Choosing the Right Approach
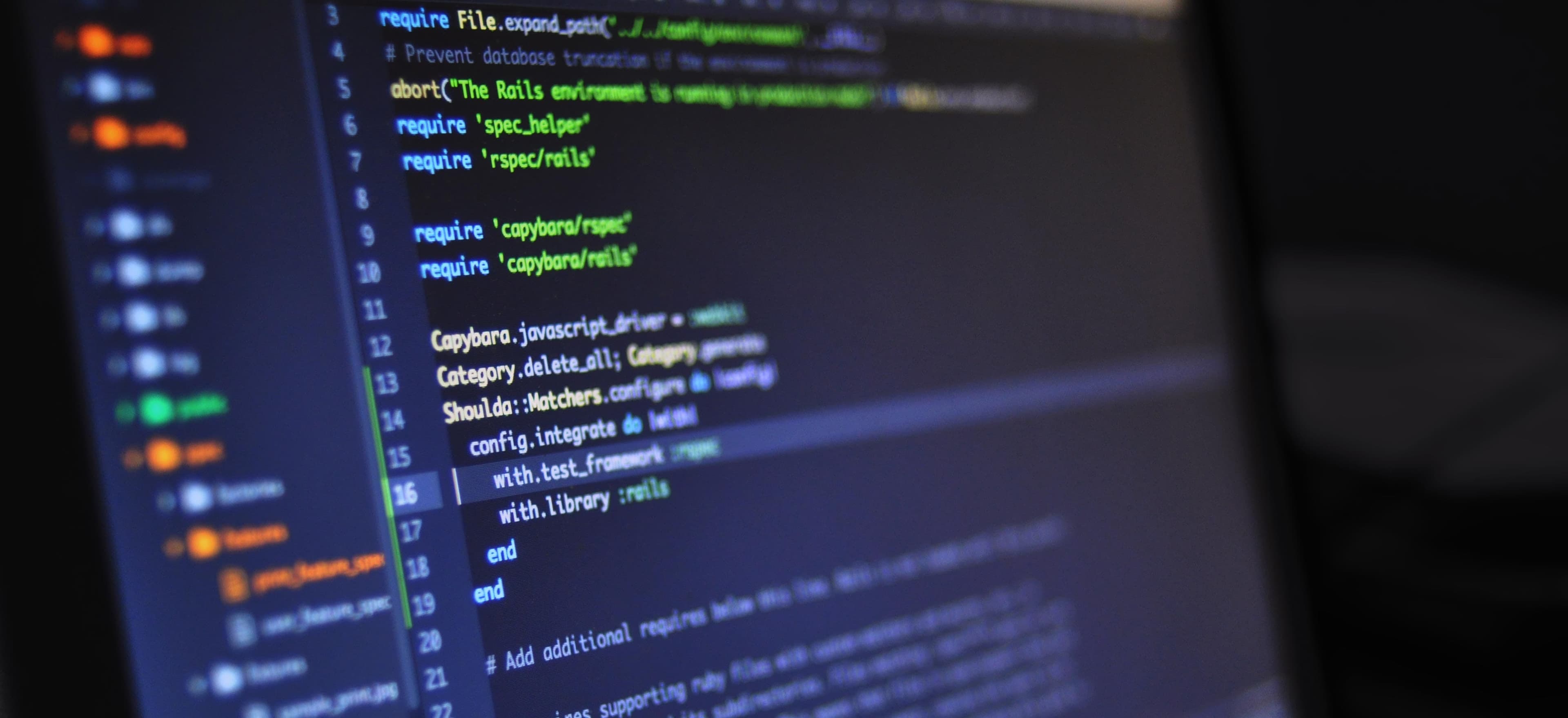
- Published on
Static vs Dynamic Analysis: Choosing the Right Approach
In today's software development landscape, ensuring the quality and correctness of code is paramount. Among the tools and techniques that developers employ, static and dynamic analysis stand out. Both methodologies serve as key components in a developer's toolkit, and understanding the differences, advantages, and use cases for each can enhance software quality. This blog post will delve into static and dynamic analysis, examining their forms, benefits, and how to effectively integrate them into your workflow.
What is Static Analysis?
Static analysis refers to the examination of code without executing it. This type of analysis typically comprises tools that analyze code for bugs, style violations, and other potential issues before the program runs. Static analysis can occur at multiple levels, including:
- Syntax Analysis: Checking for language syntax errors.
- Semantic Analysis: Evaluating logical errors and ensuring the code adheres to language rules.
- Code Quality: Measuring complexity, formatting standards, and code smells.
Example of Static Analysis
Consider this sample Java code snippet:
public int addNumbers(int a, int b) {
return a + b;
}
A static analysis tool may check for potential improvements, such as:
- Unused Variables: Ensure all declared variables are utilized.
- Naming Conventions: Confirm that method names follow Java conventions (e.g., camelCase).
Why Use Static Analysis?
-
Early Bug Detection: Bugs can be identified before the code hits production. The earlier the issue is caught, the cheaper it is to fix.
-
Consistent Coding Standards: Static analysis helps ensure a uniform code style across the team, increasing readability.
-
Automation: Many static analysis tools can be integrated with your build process, automating the evaluation of code.
For a deeper dive into static analysis tools, check out this overview on popular static analysis tools.
What is Dynamic Analysis?
Dynamic analysis, in contrast, involves executing the program with specific inputs to evaluate its behavior and performance in real-time. This form of analysis is designed to discover run-time issues that static analysis might miss, including:
- Memory Leaks: Identifying sections of code that allocate memory but fail to release it.
- Concurrency Issues: Assessing how the application behaves under concurrent processing conditions.
- Integration Errors: Ensuring that different components of the application work together seamlessly.
Example of Dynamic Analysis
Using the same Java code snippet:
public int divideNumbers(int a, int b) {
return a / b; // Potential divide by zero issue
}
A dynamic analysis tool could execute this function with different values of b
, specifically testing for edge cases such as b = 0
, which would cause a runtime exception.
Why Use Dynamic Analysis?
-
Real-time Evaluation: Dynamic analysis provides insights into software behavior during execution, highlighting issues that would go undetected in static analysis.
-
Testing for Edge Cases: Dynamic tools can simulate various input scenarios, ensuring robustness against unpredictable conditions.
-
Performance Metrics: It offers detailed performance metrics, allowing for tuning and optimization.
To explore more about dynamic analysis techniques, refer to this guide on dynamic analysis methodologies.
When to Use Static vs Dynamic Analysis
Choosing between static and dynamic analysis often depends on your development stage, project requirements, and desired outcomes. Consider the following:
-
Early Development Stage: In the early stages, static analysis is your best friend. It helps shape the code by catching errors before they proliferate.
-
Feature Completion: Once your code is nearing completion, dynamic analysis comes into play. Use it to integrate and streamline components while ensuring run-time stability.
-
Code Refactoring: During significant refactorings, utilize static analysis to flag potential issues and maintain coding standards.
Integrating Static and Dynamic Analysis
Both approaches can be combined efficiently in a continuous integration/continuous deployment (CI/CD) pipeline. Below are some strategies for integration:
CI/CD Pipeline Integration
-
Pre-Commit Hooks: Implement static analysis tools to run before code is committed to the repository. Tools like Checkstyle and PMD can ensure coding standards.
-
Automated Builds: Incorporate dynamic analysis in your automated build processes. Tools like JUnit for unit testing can run dynamic tests as part of the CI/CD workflow.
-
Regular Reviews: Schedule regular reviews of static and dynamic reports to track progress and adjust tools as needed.
Tools to Consider
-
Static Analysis Tools:
- SonarQube
- Checkstyle
- PMD
-
Dynamic Analysis Tools:
- JUnit
- JProfiler
- FindBugs
Bringing It All Together
Understanding static and dynamic analysis offers invaluable insights into software development. Static analysis serves as a robust first line of defense against bugs, ensuring code quality before execution. Dynamic analysis complements this by providing real-time insights during execution, identifying runtime issues that could lead to failures.
Remember, the best approach to software quality combines both methodologies. By integrating static and dynamic analysis into your workflow, you not only enhance code quality but also create a more resilient and maintainable software product.
Utilize the resources linked above, and consider making these tools and techniques a part of your standard operating procedures. Happy coding!
Checkout our other articles