Common Issues When Setting Up a Jersey Client for Students
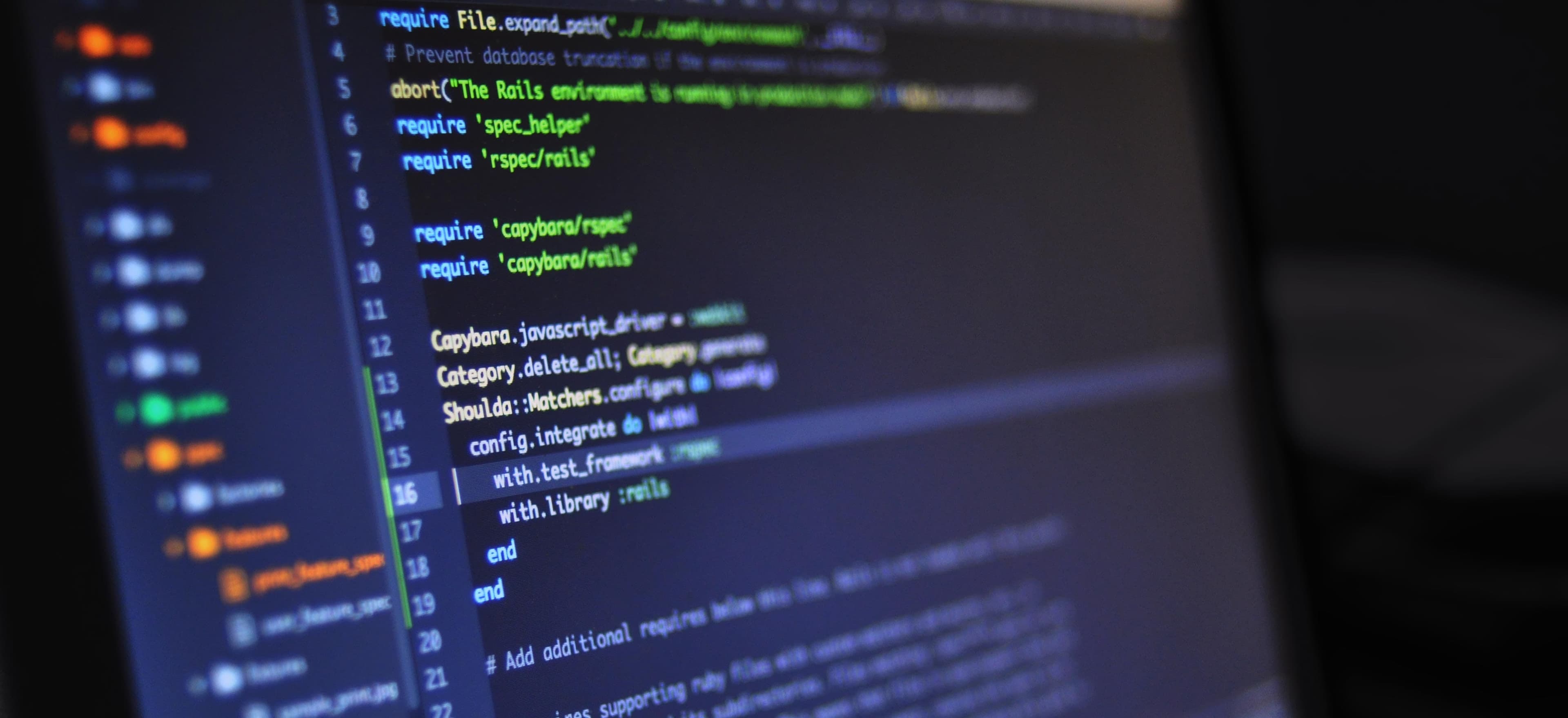
- Published on
Common Issues When Setting Up a Jersey Client for Students
Setting up a Jersey Client can be a challenging endeavor, especially for students who are just starting with RESTful web services in Java. Jersey is an open-source framework that simplifies the creation of RESTful web services in Java and is a reference implementation for JAX-RS.
In this blog post, we will explore some common issues you may encounter when setting up a Jersey Client, providing solutions and insights along the way. We'll break down the problems, share relevant code snippets, and guide you through the setup process. By the time you're done reading, you'll be better equipped to tackle these challenges head-on.
What is a Jersey Client?
Before diving into the issues, let's briefly cover what a Jersey Client is. Essentially, a Jersey Client is a Java-based client that communicates with a RESTful API. It allows you to perform HTTP requests (GET, POST, PUT, DELETE) to interact with web services.
Dependencies
To start, you'll need to include the necessary dependencies in your pom.xml
file if you're using Maven. Below is a sample dependency setup for Jersey Client.
<dependency>
<groupId>org.glassfish.jersey.core</groupId>
<artifactId>jersey-client</artifactId>
<version>2.35</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.35</version>
</dependency>
Make sure to check Maven Central for the latest versions, as they are frequently updated.
Common Issues
1. Misconfigured Dependencies
One of the most frequent issues students face is misconfiguring their dependencies. This typically arises from incompatible versions or missing libraries.
Solution:
Ensure that you have all required Jersey dependencies and that they are compatible. Cross-reference versions as shown below:
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
<version>2.35</version>
</dependency>
If you miss the dependency needed for handling JSON, your application may fail to parse JSON responses, leading to runtime exceptions.
2. Incorrect URL Endpoints
Another common pitfall is not correctly specifying the endpoint URL, which often turns out to be frustrating.
Solution:
Double-check the URL and ensure it's complete and correct. If you're testing an API, try outputting the URL using System.out.println(url);
just before making the request. This way, you can easily identify if there’s a typo.
String url = "http://your-api-endpoint.com/resource";
System.out.println("Making request to: " + url);
WebTarget target = client.target(url);
3. Handling Different HTTP Methods
Students often err in using the correct HTTP method for their requests. For example, mixing POST and GET requests can lead to unexpected behavior.
Solution:
Understand the use of each HTTP method – GET is used to retrieve data, while POST is often used for creating new resources. Here’s an example of making both GET and POST requests:
// Make a GET request
Response response = target.request(MediaType.APPLICATION_JSON).get();
if (response.getStatus() == 200) {
String jsonResponse = response.readEntity(String.class);
System.out.println("GET Response: " + jsonResponse);
}
// Make a POST request
String jsonPayload = "{\"name\":\"John Doe\"}";
Response postResponse = target.request(MediaType.APPLICATION_JSON)
.post(Entity.entity(jsonPayload, MediaType.APPLICATION_JSON));
if (postResponse.getStatus() == 201) {
System.out.println("Resource created successfully!");
}
4. Error Handling
Ignoring error handling can lead students to miss critical issues that occur during execution. It's essential to check the response status and handle error codes appropriately.
Solution:
Implement error handling by checking the response code. Here’s an enhanced example:
if (response.getStatus() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + response.getStatus());
}
This snippet ensures you're alerted of any client or server errors immediately.
5. Authentication Challenges
Sometimes, APIs might require authentication, leading to complications when you attempt to access them.
Solution:
Ensure you are providing the right authentication headers. Here's an example of how to set a Basic Authentication header:
String username = "user";
String password = "pass";
String auth = username + ":" + password;
String encodedAuth = Base64.getEncoder().encodeToString(auth.getBytes(StandardCharsets.UTF_8));
Response authResponse = target.request()
.header("Authorization", "Basic " + encodedAuth)
.get();
6. Resource Cleanup
Failing to properly close resources can lead to memory leaks and other unwanted issues, particularly when your application scales.
Solution:
Always close your Client
instance after use:
client.close();
Utilizing a try-with-resources statement can simplify this process, ensuring that resources are cleaned up automatically.
try (Client client = ClientBuilder.newClient()) {
// your code here
}
Closing the Chapter
Working with Jersey Client can be incredibly rewarding as you build RESTful applications in Java. However, it's essential to navigate the common issues that might arise during the setup process. By understanding the common pitfalls like misconfigured dependencies, incorrect URL endpoints, HTTP method usage, error handling, authentication challenges, and resource cleanup, you can build more robust applications.
For further reading on Jersey Client setup and error handling, you might find these resources helpful:
Keep experimenting, and don’t hesitate to revisit this guide whenever you find yourself stuck. Happy coding!