Securing JWT in Social Authentication: Common Pitfalls
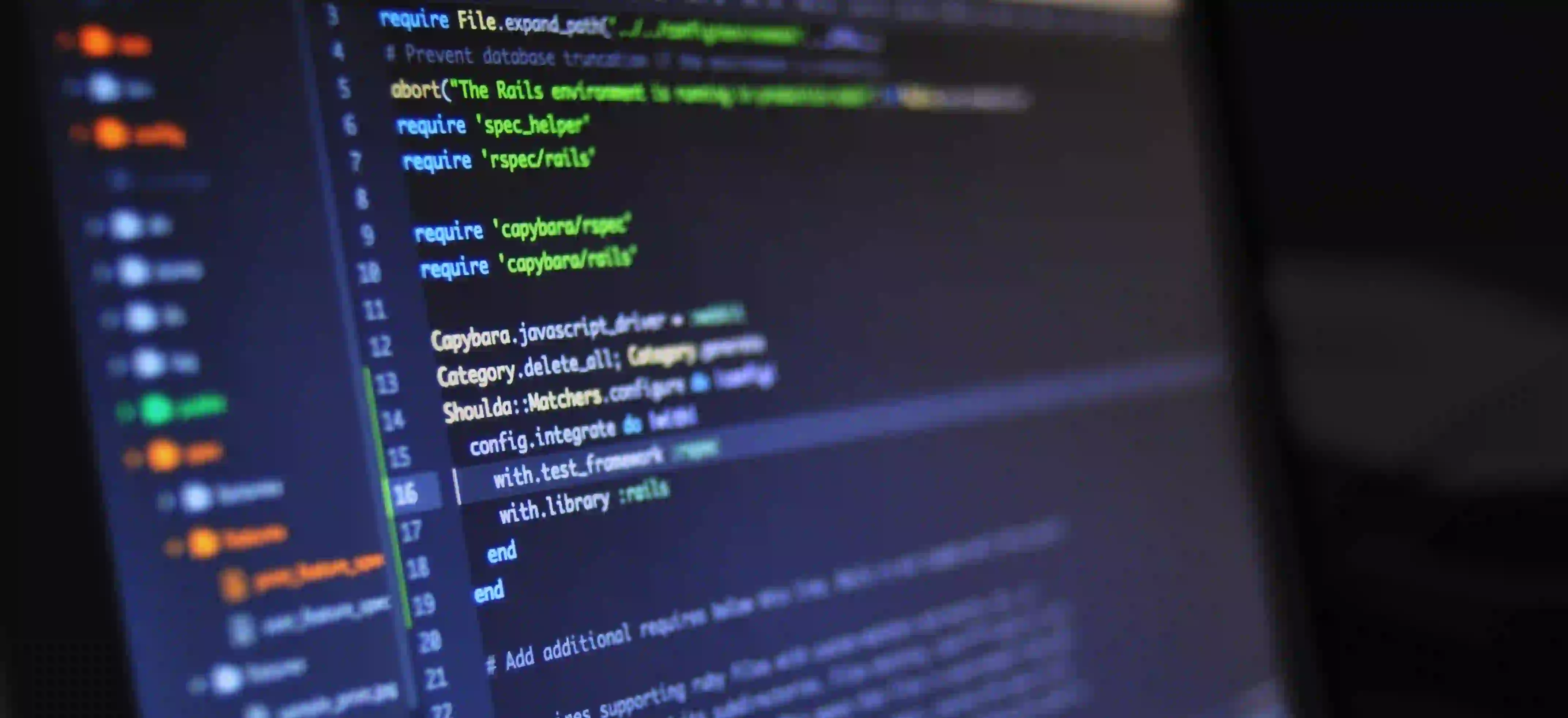
Securing JWT in Social Authentication: Common Pitfalls
In recent years, using JSON Web Tokens (JWT) for authentication has become increasingly popular, particularly for implementing social authentication. This method allows users to log in to an application through third-party services such as Google, Facebook, and Twitter. While JWT provides a stateless and scalable way to manage user sessions, it comes with its own set of challenges and potential security pitfalls.
In this blog post, we will explore the common mistakes developers make when securing JWT in social authentication. We will also provide strategies to avoid these pitfalls and implement a secure authentication flow.
What is JWT?
Before diving into security concerns, let's briefly clarify what JWT is. A JSON Web Token is a compact, URL-safe means of representing claims to be transferred between two parties. The claims in a JWT are encoded as a JSON object that's used as the payload of a JSON Web Signature (JWS) structure or as the plain text of a JSON Web Encryption (JWE) structure.
The structure generally consists of three parts:
- Header
- Payload
- Signature
Here's a simple example of creating a JWT in Java:
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import java.util.Date;
public class TokenGenerator {
private static final String SECRET_KEY = "mySecretKey"; // Use a strong secret
public static String generateToken(String userId) {
return Jwts.builder()
.setSubject(userId)
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + 86400000)) // Token valid for 1 day
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
}
Here, we create a JWT that contains the user ID as the subject, an issued date, and an expiration date. The token is signed with a secret key using the HMAC SHA-256 algorithm.
Now that we've established a basic understanding of JWT, let’s examine the common pitfalls when integrating JWT for social authentication.
Common Pitfalls When Using JWT in Social Authentication
1. Weak Secret Keys
One of the most critical vulnerabilities arises from weak secret keys. If your secret key can be easily guessed or compromised, an attacker can generate valid tokens at will.
Solution: Use a strong, random secret key and frequently rotate it. A random string of characters long enough to resist brute-force attacks is essential.
2. Lack of Token Expiration
Tokens that do not expire can lead to serious security issues. If an attacker obtains a JWT without an expiration time, they can utilize it indefinitely.
Improved Code:
.setExpiration(new Date(System.currentTimeMillis() + 86400000)) // Token valid for 1 day
In this example, we set the expiration to 1 day. This limits the lifespan of the token, reducing the risk associated with token theft.
3. Not Invalidating Tokens
Another common mistake is failing to invalidate tokens when a user logs out. If a token persists after a user has logged out, anyone who has it can still access protected resources.
Solution: Implement a token revocation mechanism. Store invalidated tokens in a blacklist database and check incoming tokens against this list.
4. Overlooking Refresh Tokens
Many developers forget to implement refresh tokens alongside access tokens. Access tokens typically have a short lifespan, while refresh tokens can last longer and are used to request new access tokens.
Implementation:
Here’s a simple way to implement refresh tokens in Java:
// Assuming we have a database to store refresh tokens
public String createRefreshToken(String userId) {
String refreshToken = Jwts.builder()
.setSubject(userId)
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + 604800000)) // 7 days
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
// Save refresh token to the database (not shown)
return refreshToken;
}
In this method, we create a refresh token valid for seven days, enabling the application to maintain user sessions securely.
5. Ignoring Algorithm Mismatch
JWT allows the use of different algorithms to sign tokens (e.g., HMAC SHA256, RS256). However, not validating the algorithm used can open security channels for attackers.
Solution: Always validate the algorithm specified in the header. Avoid using the "none" algorithm, which permits unsigned tokens.
6. Insufficient Claims Validation
When using social authentication, it's vital to validate claims from the JWT payload. For example, you should verify the iss
(issuer) claim to ensure the JWT was issued by a trusted provider.
Example:
Claims claims = Jwts.parser()
.setSigningKey(SECRET_KEY)
.parseClaimsJws(token)
.getBody();
if (!claims.getIssuer().equals("TrustedProvider")) {
throw new SecurityException("Invalid issuer");
}
By checking the issuer, we ensure that our application only accepts JWTs from authorized providers.
7. Failing to Protect Against Cross-Site Scripting (XSS)
XSS vulnerabilities can expose JWTs stored in local storage, allowing attackers to hijack user sessions.
Solution: Use HTTP-only cookies to store JWTs. This way, the tokens cannot be accessed via JavaScript. Additionally, ensure proper validation and sanitization of user inputs to mitigate XSS risks.
8. Inadequate Token Storage
Storing JWTs in local storage can be risky. If an attacker gains access to users' browsers, they can extract the tokens.
Recommendation: Use secure, HTTP-only cookies for token storage. This approach limits exposure and aids security.
My Closing Thoughts on the Matter: A Secure Approach to JWT and Social Authentication
When implementing social authentication with JWT, being aware of common security pitfalls is crucial. By adopting best practices and employing robust security measures, you can safeguard user data and maintain secure sessions.
A summary of key points to remember:
- Use strong secret keys and rotate them.
- Implement token expiration and revocation.
- Utilize refresh tokens for better session management.
- Validate algorithm and claims.
- Store tokens in secure, HTTP-only cookies.
For more on securing JWT and understanding social authentication, consider checking JWT.io and OWASP JWT Cheat Sheet.
By following these guidelines, you can greatly enhance the security of your applications leveraging JWT for social authentication. Stay safe and code securely!