Troubleshooting Hot Deployment Issues in IntelliJ IDEA
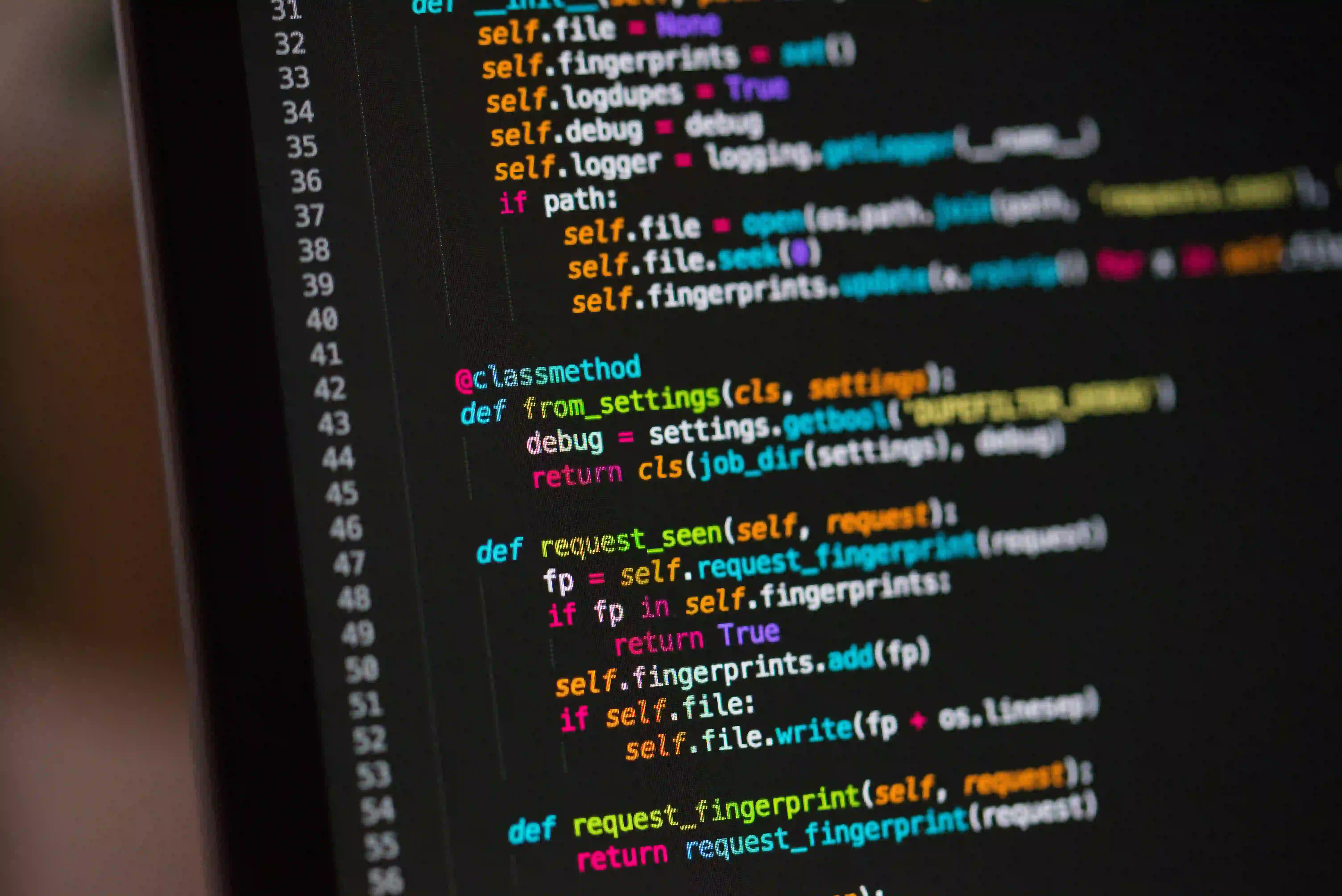
Troubleshooting Hot Deployment Issues in IntelliJ IDEA
Developers today rely heavily on hot deployment features for a more efficient workflow during application development. Hot deployment allows changes to your code to take effect without requiring a complete restart of your application, saving both time and resources. However, it's not infallible and can come with its own set of challenges—especially in integrated development environments (IDEs) like IntelliJ IDEA. In this post, we will explore common hot deployment issues in IntelliJ IDEA and provide you with troubleshooting tips to streamline your development process.
What is Hot Deployment?
Hot deployment (or hot swapping) refers to the ability to deploy changes to a live system without stopping the system. This is particularly useful in development settings where programmers need to test small changes frequently. It typically involves reloading classes or components of your application so that the developer can see the effect of recent changes immediately.
Benefits of Hot Deployment
- Increased Productivity: Saving time by not having to restart the server.
- Immediate Feedback: Quickly see the effects of your changes.
- Testing: Test changes in real-time without altering the server state.
Dependencies on Hot Deployment
Hot deployment generally relies on Java's Class Loading mechanism. When an application is running, new or updated classes can be loaded while the application continues to run. The effectiveness of hot deployment, however, can vary based on several factors, including the application server, framework, and IDE.
Common Issues with Hot Deployment in IntelliJ IDEA
1. Changes Not Reflected
One of the most frustrating issues is when you make a change, save it, and do not see any effect in the running application. This may occur due to various reasons:
Solution: Rebuild Project Configuration
Make sure your project is set to build upon changes. You can configure this in your IntelliJ settings:
- Go to File > Settings (or Preferences on macOS).
- Navigate to Build, Execution, Deployment > Compiler.
- Check the box for "Build project automatically."
This ensures that any changes you make are picked up by the IDE and reflected in your running application.
Code Snippet Example
public class MyService {
// Original method
public String processData(String input) {
return "Data processed: " + input;
}
}
// Modify this method during hot deployment
public String processData(String input) {
return "Processed Data: " + input.toUpperCase(); // Changes not usually reflected without a rebuild
}
2. Class Loader Issues
Java’s class loader is responsible for loading classes at runtime. Sometimes, changes in method signatures or dependencies can lead to class loader conflicts.
Solution: Clean and Build the Project
Often, class loader conflicts occur if the changes are not reflected correctly. You can do the following:
- From the main menu, select Build > Clean Project.
- Then, choose Build > Rebuild Project.
Cleaning helps in eliminating old configurations and ensuring that you have a fresh start.
3. Server Configuration Issues
The server settings can also affect hot deployment. Sometimes, the application server (like Tomcat, WildFly, etc.) needs to be configured properly to facilitate hot deployment.
Solution: Review Server Configuration in IntelliJ
- Navigate to Run > Edit Configurations.
- Ensure that "On frame deactivation" is set to “Update classes and resources”. This setting enables the server to update the changes without requiring a full restart.
4. Framework-Specific Limitations
Different frameworks have different support levels for hot deployment:
- Spring Boot: Often used with DevTools for live reloading.
- Java EE: May have more restrictions compared to Spring.
Solution: Utilize Framework-Specific Tools
For example, in Spring Boot, including the Spring Boot DevTools dependency can enhance your hot deployment experience.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
</dependency>
Including this in your pom.xml
will facilitate better reload capabilities when developing a Spring Boot application.
5. IDE Not Responding to Changes
Sometimes, even after all configurations are correct, the IDE does not seem to register changes made in external files or resources.
Solution: Restart IntelliJ IDEA
While this may sound trivial, sometimes the issue lies with the IDE itself. If you still can't get hot deployment to reflect changes:
- Restart IntelliJ IDEA.
- Ensure you have the latest version, as updates often fix bugs related to hot deployment.
Best Practices for Troubleshooting Hot Deployment Issues
- Use Version Control: Keep track of changes and revert if necessary.
- Log Errors: Check the application's logs to identify class-loading errors.
- Consult Documentation: Every framework and server can have unique configurations. Understand how they handle hot deployment.
- Stay Updated: Regularly update IntelliJ IDEA and plugins for the latest features and fixes.
Final Thoughts
Hot deployment is a powerful feature that can drastically improve your productivity as a developer. However, issues may arise that can hinder this advantage. By ensuring that you're correctly configuring IntelliJ IDEA, understanding how Java class loaders work, and utilizing framework-specific tools, you can troubleshoot and resolve most issues.
For additional insights, you might find these resources helpful:
By keeping these tips in mind, you can achieve a smoother development experience and make the most of the hot deployment features in IntelliJ IDEA. Happy coding!