Common Mistakes When Finding Transpose of a Matrix in Java
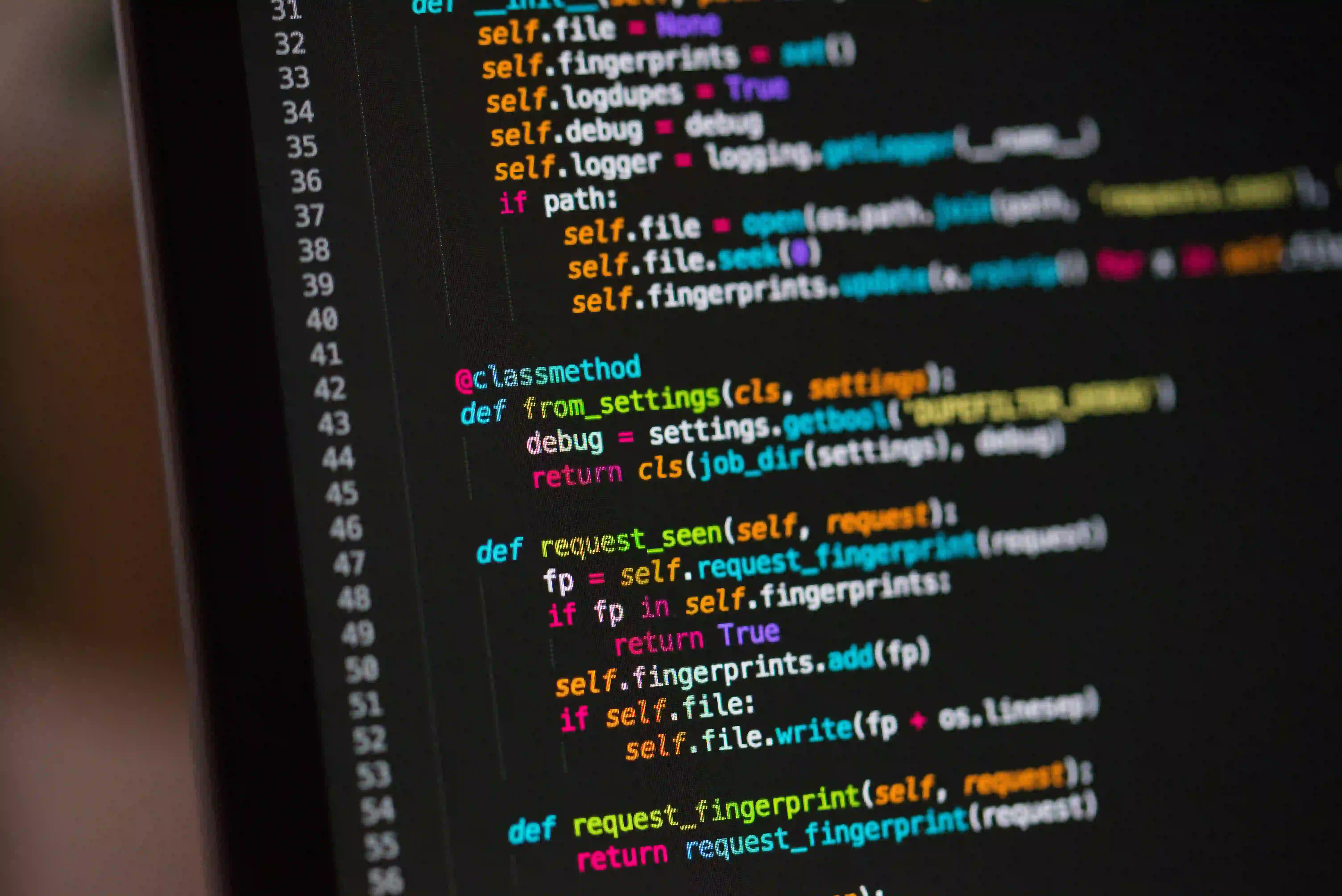
Common Mistakes When Finding the Transpose of a Matrix in Java
Matrix operations are fundamental in various fields like mathematics, data science, and computer science. Among these operations, the transpose of a matrix is one of the most commonly encountered tasks. A transpose of a matrix is formed by swapping rows and columns. Despite its simplicity, many developers make mistakes while implementing this operation in Java. In this blog post, we'll discuss these common pitfalls and provide clear code examples to illustrate the correct approach to finding the transpose of a matrix in Java.
What is a Matrix Transpose?
The transpose of a matrix flips its dimensions. For example, if you have a matrix:
1 2 3
4 5 6
Its transpose would be:
1 4
2 5
3 6
Here, the first row of the original matrix becomes the first column of the transposed matrix, and so on.
Common Mistakes
1. Incorrect Matrix Dimensions
It's essential to ensure that the dimensions of the resultant transposed matrix are defined correctly. Developers often forget to initialize the transposed matrix with the correct dimensions.
Example Mistake:
int[][] original = {
{1, 2, 3},
{4, 5, 6}
};
// Incorrect dimension for transposed matrix
int[][] transposed = new int[2][3]; // This will lead to ArrayIndexOutOfBounds
Correction:
// Correct dimensions for transposing
int[][] transposed = new int[original[0].length][original.length];
// Perform transposition
for (int i = 0; i < original.length; i++) {
for (int j = 0; j < original[0].length; j++) {
transposed[j][i] = original[i][j]; // Swapping
}
}
// transposed is now correctly populated
2. Misunderstanding Row and Column Indices
Mismanaging row and column indices is another common mistake. Developers sometimes confuse the two when filling out the new transposed matrix, leading to logical errors.
Example Mistake:
for (int i = 0; i < original.length; i++) {
for (int j = 0; j < original[0].length; j++) {
// Incorrectly transposing
transposed[i][j] = original[j][i]; // This will cause an error
}
}
Correction:
Make sure you remember that transposed[j][i] = original[i][j];
is the correct way to perform the swap.
3. Using Wrong Loop Conditions
Another frequent mistake is using incorrect boundaries for loops. Depending on the matrix's dimensionality, accidentally iterating beyond the matrix limits can lead to runtime exceptions.
Example Mistake:
for (int i = 0; i <= original.length; i++) { // Using <= instead of <
for (int j = 0; j <= original[0].length; j++) { // Same here
transposed[j][i] = original[i][j]; // Error
}
}
Correction:
Loops should strictly use <
for their conditions:
for (int i = 0; i < original.length; i++) {
for (int j = 0; j < original[0].length; j++) {
transposed[j][i] = original[i][j]; // Correct assignment
}
}
4. Ignoring Irregular Matrices
Matrix transposition typically involves rectangular matrices. If you attempt to transpose an irregular matrix (ragged arrays), you might run into issues since the lengths of the rows can vary.
Example Mistake:
int[][] irregularMatrix = {
{1, 2},
{3, 4, 5}
};
// This approach will lead to runtime exceptions.
Correction:
When working with irregular matrices, explicitly handle each row's length during transposition.
int[][] irregularMatrix = {
{1, 2},
{3, 4, 5}
};
// Create a temporary transposed array
int maxLength = 0;
for (int[] row : irregularMatrix) {
if (row.length > maxLength) {
maxLength = row.length;
}
}
int[][] transposed = new int[maxLength][irregularMatrix.length];
// Perform transposition
for (int i = 0; i < irregularMatrix.length; i++) {
for (int j = 0; j < irregularMatrix[i].length; j++) {
transposed[j][i] = irregularMatrix[i][j];
}
}
5. Not Accounting for Edge Cases
When transposing a matrix, it is crucial to handle special cases, such as empty matrices or 1x1 matrices.
Example Mistake:
int[][] emptyMatrix = {};
// Attempting to transpose without checks
int[][] transposed = new int[emptyMatrix[0].length][emptyMatrix.length];
Correction:
Always check for edge cases before performing operations:
if (original.length == 0 || original[0].length == 0) {
System.out.println("Cannot transpose an empty matrix.");
} else {
int[][] transposed = new int[original[0].length][original.length];
// Proceed with transpose logic
}
Complete Transpose Method Example
Let's put everything together into a complete, reusable Java method to demonstrate the correct implementation:
public class MatrixTranspose {
public static int[][] transpose(int[][] original) {
// Handle edge case for empty matrix
if (original.length == 0 || original[0].length == 0) {
System.out.println("Cannot transpose an empty matrix.");
return new int[0][0];
}
// Initialize transposed matrix
int[][] transposed = new int[original[0].length][original.length];
// Perform transposition
for (int i = 0; i < original.length; i++) {
for (int j = 0; j < original[i].length; j++) {
transposed[j][i] = original[i][j];
}
}
return transposed;
}
public static void main(String[] args) {
int[][] matrix = {
{1, 2, 3},
{4, 5, 6}
};
int[][] transposedMatrix = transpose(matrix);
// Output transposed matrix
for (int[] row : transposedMatrix) {
for (int val : row) {
System.out.print(val + " ");
}
System.out.println();
}
}
}
Why This Matters
Understanding how to properly transpose a matrix in Java—not just the code but also the logic behind it—is critical for preventing bugs and ensuring data is manipulated correctly. Mistakes in matrix operations can lead to cascading errors in applications, especially in data-driven fields like machine learning and computational mathematics.
For more intricate details on working with matrices, consider visiting GeeksforGeeks for additional resources.
Closing Remarks
Transposing a matrix in Java is straightforward, but it comes with its share of common pitfalls. By understanding these frequent mistakes and monitoring each step, developers can efficiently and accurately work with matrices. By adhering to the corrected examples and best practices outlined in this post, you can avoid the common traps that many encounter when dealing with this fundamental data structure.
Remember, whether you are tackling simple transpositions or complex algorithms in high-performance applications, a strong foundation in these basic operations makes a world of difference. Happy coding!