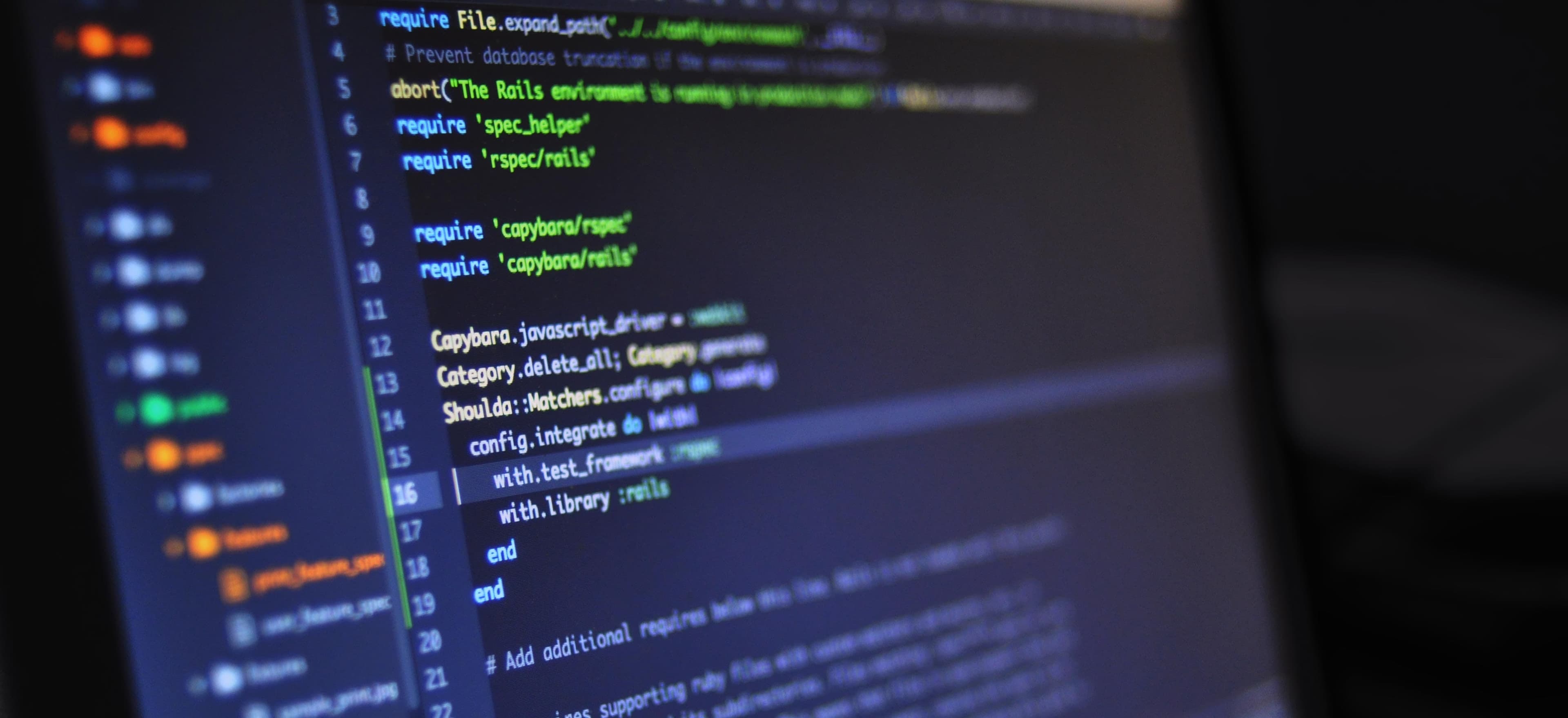
- Published on
Enhancing JavaFX UI: Mastering Callback Interfaces
As Java continues to grow as one of the most popular programming languages, its capabilities in creating user-friendly interfaces have also advanced tremendously. JavaFX is a robust framework for creating rich internet applications, enhancing the Java UI experience. In this blog post, we will delve deep into callback interfaces in JavaFX, demonstrating their importance and how to implement them effectively.
What Are Callback Interfaces?
A callback interface in Java is essentially a method that is passed as an argument to a higher-order function or a thread. This allows you to write more flexible, reusable code. In JavaFX, callback interfaces are especially critical for handling user events, such as clicks or keyboard input.
The Importance of Callback Interfaces
When designing an UI, user actions need to be handled effectively without complicating the code structure. Callback interfaces can:
- Decouple components: Separate the code that triggers events from the code that handles them.
- Improve code readability: By using concise method calls and naming conventions, code becomes easier to read and maintain.
- Allow for reusability: Implementing generic callback interfaces allows different components to use the same logic.
Basic Implementation of Callback Interfaces
Let's start with a straightforward callback interface in JavaFX. In this example, we will create a simple dialog that accepts user input and triggers a callback when a button is clicked.
Step 1: Creating a Callback Interface
First, define an interface. This interface will contain a single method named onInputReceived
. The method takes a String
as a parameter to process user input.
public interface InputCallback {
void onInputReceived(String input);
}
Step 2: Implementing the Callback
Next, create a class where we will implement this interface.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextInputDialog;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class CallbackExample extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage stage) {
Button button = new Button("Enter something");
// Call to action using the callback
button.setOnAction(e -> showInputDialog(input -> {
System.out.println("User input: " + input);
}));
VBox vbox = new VBox(button);
Scene scene = new Scene(vbox, 300, 200);
stage.setScene(scene);
stage.setTitle("Callback Example");
stage.show();
}
private void showInputDialog(InputCallback callback) {
TextInputDialog dialog = new TextInputDialog();
dialog.setTitle("Input Dialog");
dialog.setHeaderText("Please enter your input:");
// Use the optional dialog result to invoke the callback
dialog.showAndWait().ifPresent(input -> callback.onInputReceived(input));
}
}
Explanation of the Code
-
Interface Definition: The
InputCallback
interface defines the methodonInputReceived
, which can be implemented by classes that handle user input. -
Main Application Class: The
CallbackExample
class extendsApplication
, making it the main entry point of the JavaFX application. -
Button Setup: When the button is clicked, it opens a text input dialog box via the
showInputDialog
method. -
Dialog Handling: The
showInputDialog
method accepts anInputCallback
implementation. When the user provides input and closes the dialog, the callback method is invoked.
Enhancing User Interface Responsiveness
Using callback interfaces enhances the user experience significantly. For instance, we can add more functionality to the callback mechanism by allowing multiple callbacks.
public interface MultiInputCallback {
void onInputReceived(String input1, String input2);
}
// Inside your CallbackExample class
private void showMultiInputDialog(MultiInputCallback callback) {
TextInputDialog firstDialog = new TextInputDialog();
firstDialog.setTitle("Input Dialog");
firstDialog.setHeaderText("Please enter first input:");
firstDialog.showAndWait().ifPresent(input1 -> {
TextInputDialog secondDialog = new TextInputDialog();
secondDialog.setTitle("Second Input");
secondDialog.setHeaderText("Please enter second input:");
secondDialog.showAndWait().ifPresent(input2 -> callback.onInputReceived(input1, input2));
});
}
Usage Example
To use the showMultiInputDialog
method, simply call it within the button action and provide your callback implementation:
button.setOnAction(e -> showMultiInputDialog((input1, input2) -> {
System.out.println("User inputs: " + input1 + ", " + input2);
}));
Advanced UI Interaction with Callbacks
JavaFX supports various UI components, such as sliders, combo boxes, and tables. Callbacks play a significant role in managing these components interactively.
Example: Slider Callback
Let’s create a slider that modifies a text label based on the selected value.
import javafx.scene.control.Label;
import javafx.scene.control.Slider;
// Inside your CallbackExample class
private void initializeSlider(VBox layout) {
Slider slider = new Slider(0, 100, 50);
Label sliderLabel = new Label("Value: " + slider.getValue());
// Bind the label text to the slider value
slider.valueProperty().addListener((observable, oldValue, newValue) -> {
sliderLabel.setText("Value: " + newValue.intValue());
});
layout.getChildren().addAll(slider, sliderLabel);
}
// Call initializeSlider(layout) in your start method.
Benefits of Using Callbacks in JavaFX
-
Decoupling Logic: Since callbacks are separate from the UI logic, you can reuse them in different contexts without changing the main code structure.
-
Asynchronous Operations: Callbacks can handle operations happening after long processes, keeping the UI responsive. This is essential for tasks like file loading or network requests.
-
Enhanced Testing: With callback interfaces, you can easily mock dependencies in unit testing, allowing for better isolation of test cases.
My Closing Thoughts on the Matter
Incorporating callback interfaces within your JavaFX applications can significantly improve the maintainability and readability of your code. They serve as a powerful tool to handle user events efficiently and allow for more engaging and interactive experiences.
For further understanding, you might want to check this detailed JavaFX guide or explore the JavaFX documentation.
By mastering callback interfaces, you can take your JavaFX UI design to the next level, ultimately enhancing not only the functionality but also the user experience of your applications. Happy coding!