Unveiling Spring Boot 3.5: Key Features You Can't Miss!
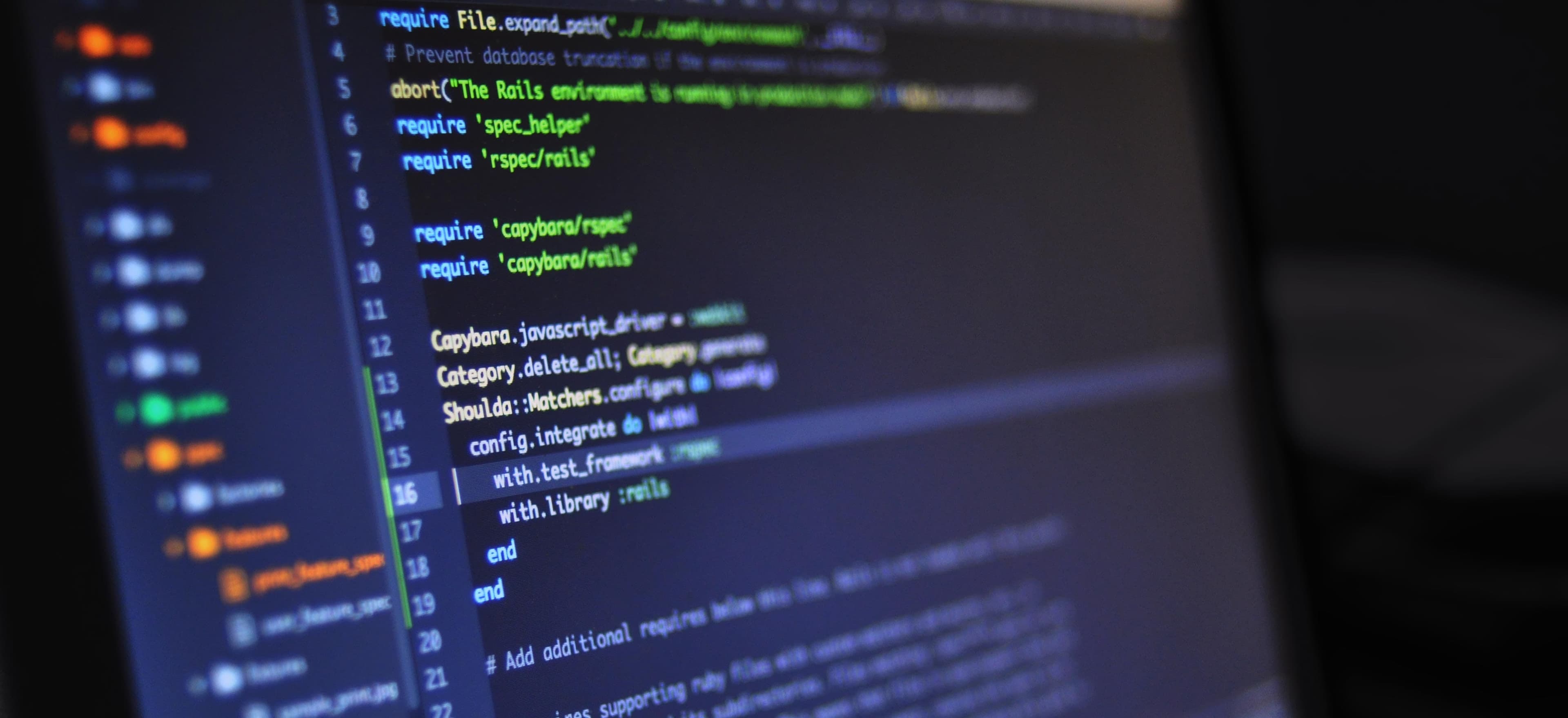
- Published on
Unveiling Spring Boot 3.5: Key Features You Can't Miss!
Spring Boot is an immensely popular framework for building production-ready applications in Java quickly. As of the latest release, Spring Boot 3.5, several new features and enhancements elevate its performance and developer experience. In this blog post, we'll explore the key features of Spring Boot 3.5 that you cannot afford to overlook.
Table of Contents
- What is Spring Boot?
- Key Features of Spring Boot 3.5
- 2.1 Java 21 Support
- 2.2 Enhanced Configuration Properties
- 2.3 Native Image Support
- 2.4 Improved Dependency Injection
- 2.5 Spring Security Enhancements
- Conclusion
What is Spring Boot?
Spring Boot simplifies the process of developing applications with the Spring framework by providing a suite of tools and features that eliminate boilerplate code. It allows developers to focus on writing business logic rather than on complex configurations. Spring Boot's convention-over-configuration principle makes it a top choice for building microservices and cloud-based applications.
Key Features of Spring Boot 3.5
2.1 Java 21 Support
One of the most significant updates in Spring Boot 3.5 is its support for Java 21. This compatibility ensures that developers can leverage the latest performance improvements and new language features in their applications.
What makes Java 21 noteworthy? It introduces features such as:
- Pattern Matching for Switch: This simplifies coding by allowing developers to switch on types and values directly.
Example:
switch (obj) {
case String s -> System.out.println("String value: " + s);
case Integer i -> System.out.println("Integer value: " + i);
default -> System.out.println("Unknown type");
}
This feature reduces boilerplate code, making the code cleaner and more readable.
- Record Classes: These help manage immutable data easily, enhancing the readability of the data models within your application.
Overall, Java 21's features promote cleaner, more maintainable code, improving the overall developer experience.
2.2 Enhanced Configuration Properties
In Spring Boot 3.5, the emphasis on configuration management takes a leap forward. The @ConfigurationProperties
annotation can now automatically map to more complex types.
Consider this example:
@ConfigurationProperties(prefix = "app.settings")
public class AppSettings {
private String name;
private List<String> values;
// Getters and Setters
}
This allows Spring Boot to bind properties in your application.yml
or application.properties
files directly to an object. Spring Boot can validate these properties at startup, ensuring that your application fails fast if necessary properties are missing. This makes configuration management intuitive and error-free.
2.3 Native Image Support
With continued focus on performance, Spring Boot 3.5 extends its support for GraalVM Native Image. This functionality allows developers to compile Spring applications into native binaries, which can significantly reduce startup time and memory usage.
Why go native?
- Fast Startup: Native images start within seconds, making them ideal for microservices and serverless architectures.
- Reduced Memory Footprint: Applications running as native images consume less memory.
Here's a simple build configuration snippet for creating a native image:
spring:
native:
build:
image:
active: true
To generate the native image, you can use:
./mvnw package -Pnative
When you run your application, you can expect enhanced performance, making it suitable for resource-constrained environments.
2.4 Improved Dependency Injection
The dependency injection container in Spring Boot 3.5 has been fine-tuned to reduce boilerplate even further.
Consider this example of creating a service class:
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
This DI mechanism is straightforward and clearer. Spring Boot 3.5 also introduces improved runtime validation of dependencies, quickly notifying developers of configuration issues.
Comprehensive error messages allow developers to fix errors promptly. Enhanced diagnostics mean you can track down problems more quickly. You can focus on building your application rather than chasing down misconfigured beans.
2.5 Spring Security Enhancements
Security is paramount in modern applications, and Spring Boot 3.5 brings several enhancements to Spring Security.
Notably, the new features include:
- Passwordless Authentication: Users can log in using methods like SMS or email tokens, which enhance user experience and security.
- OAuth 2.1: Combined OAuth 2.0 and OpenID Connect into a simple package to make implementing secure access simpler.
To implement passwordless authentication, you might create a controller like this:
@RestController
public class AuthController {
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody LoginRequest request) {
// Validate user login and send a token
}
}
With these features, securing your application becomes less convoluted, allowing developers to implement robust security features with ease.
The Bottom Line
Spring Boot 3.5 is truly a gem for developers who embrace the modern development paradigm. With comprehensive support for the latest Java features, enhanced configuration handling, native image capabilities, streamlined dependency injection, and upgraded security features, this release is designed to make developers' lives easier and their applications more powerful.
These key features not only enhance productivity but also ensure that applications built using Spring Boot leverage scalability and maintainability.
Whether you are a seasoned developer or just getting started, exploring Spring Boot 3.5 is well worth your time. To dive deeper, consider checking out the official Spring documentation or explore some hands-on projects to see these features in action.
Happy coding!