Mastering Fragment Lifecycles for Seamless Multi-Screen Apps
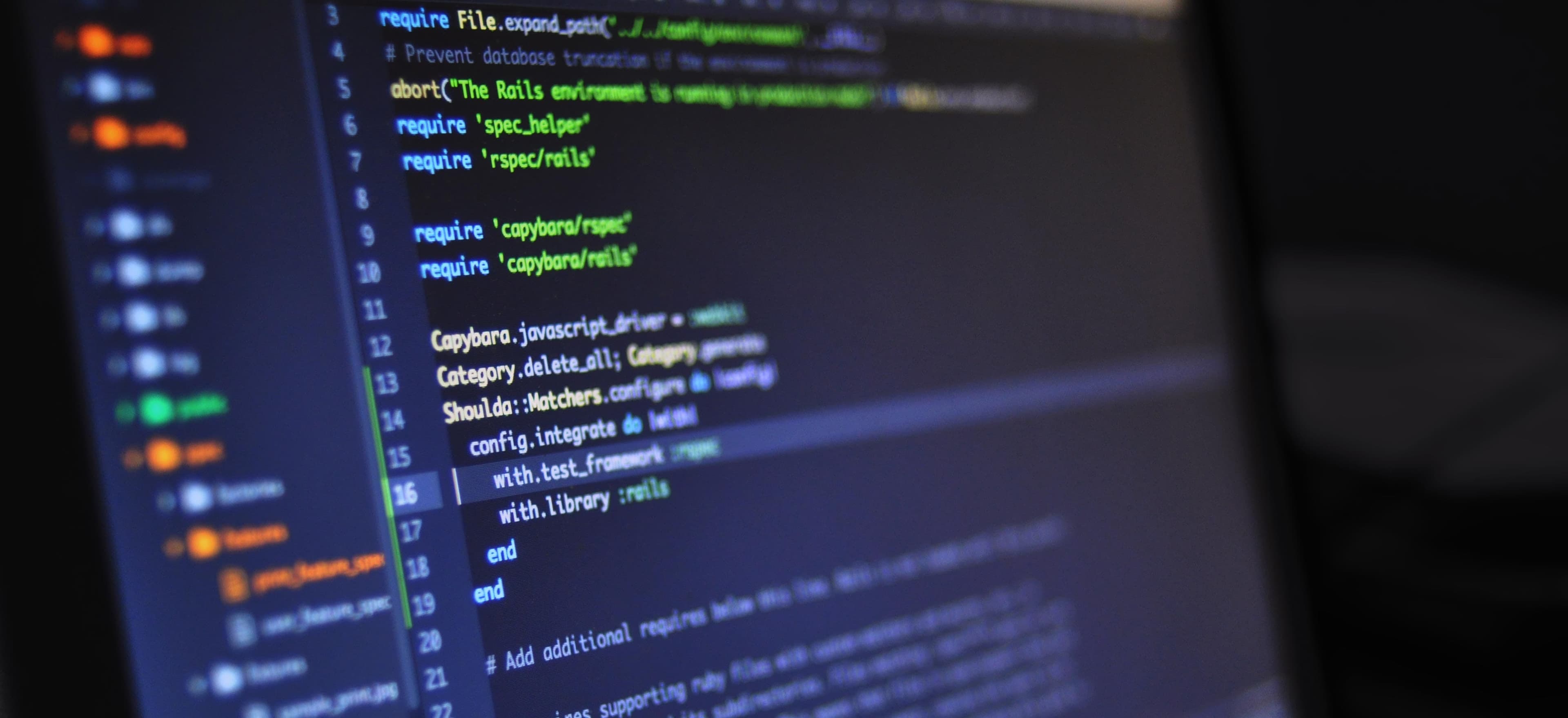
- Published on
Mastering Fragment Lifecycles for Seamless Multi-Screen Apps
In the world of Android development, achieving a seamless user experience across multiple screens is paramount. One of the core components enabling this flexibility is the Fragment. Understanding the Fragment lifecycle is crucial for developers looking to create responsive and efficient multi-screen apps. In this blog post, we will delve deeply into Fragment lifecycles, providing you with clear explanations, exemplary code snippets, and practical tips to master their use.
What is a Fragment?
A Fragment is a modular section of an activity that has its own lifecycle, receives its own input events, and can be added or removed while the activity is running. Think of fragments as the building blocks of your user interface, allowing you to create dynamic and flexible applications.
Why Use Fragments?
- Modularity: Fragments promote code reusability.
- Responsive UI: They allow for different UI layouts on various screen sizes.
- Back Stack Management: Fragments can manage their own back stack.
Understanding Fragment Lifecycle
The Fragment lifecycle is largely dependent on the parent Activity lifecycle. Understanding these states allows you to maintain a smooth user experience while managing resources effectively.
Here’s a visual representation of the Fragment lifecycle for clarity:
[Activity Created] --> [onAttach]
|
v
[onCreate]
|
v
[onCreateView]
|
v
[onViewCreated]
|
v
[onActivityCreated]
Key Lifecycle Methods
-
onAttach(): Called when the Fragment is first attached to its context. It's a good place to retrieve references to the Activity.
@Override public void onAttach(Context context) { super.onAttach(context); Log.d("Fragment", "Fragment Attached"); }
-
onCreate(): This is where you initialize your fragment, often for setting up arguments or performing configurations.
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); Log.d("Fragment", "Fragment Created"); }
-
onCreateView(): This method is used for inflating the layout for the fragment. It returns the View to display.
@Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment return inflater.inflate(R.layout.fragment_layout, container, false); }
-
onActivityCreated(): Invoked when the activity's onCreate() method has returned. It's safe to use the activity's UI components here.
-
onStart(): The fragment is now visible to the user. This is a good time to start animations, initialize components, or interact with the Activity.
-
onResume(): At this point, the fragment is ready to interact with the user. Use it to start animations or acquire user inputs.
-
onPause() and onStop(): These methods are crucial for resource management. Pause ongoing tasks or release heavy resources when the fragment is no longer in the foreground.
-
onDestroyView(): Cleanup your views and save data to prevent memory leaks.
-
onDetach(): Called when the fragment is detached from the activity. Good for releasing references to avoid memory leaks.
Managing Fragment Transactions
Fragment transactions allow you to manipulate fragments in your application. You can add, remove, replace, and perform other operations dynamically:
FragmentTransaction transaction = getFragmentManager().beginTransaction();
MyFragment fragment = new MyFragment();
transaction.add(R.id.fragment_container, fragment);
transaction.addToBackStack(null); // Allows for back navigation
transaction.commit();
Explanation of the code:
- FragmentTransaction: This class allows you to perform actions on a fragment.
- add(): This method adds a fragment to an activity.
- addToBackStack(): This method allows the user to navigate back to the previous fragment, which is essential for a good user experience.
Tips for Mastering Fragment Lifecycles
-
Use ViewModels: Encapsulate your app's data to retain it across configuration changes. Using ViewModels with Fragments helps you to keep UI-related data alive.
-
Avoid Memory Leaks: Be mindful of holding on to references of the Activity within your Fragment to prevent memory leaks. Use WeakReferences where necessary.
-
Handle State Restoration: Implement onSaveInstanceState to save the state of your fragments when a configuration changes occur, such as screen rotation.
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("key", "value");
}
Useful Resources
To deepen your understanding of using Fragments and enhancing your Android projects, I recommend checking the following resources:
Final Thoughts
Mastering Fragment lifecycles is essential for developing robust Android applications with seamless multi-screen capabilities. Through wise management of Fragment lifecycles, developers can provide smoother user experiences while ensuring efficient resource utilization.
Remember, the key to working effectively with Fragments lies in understanding their lifecycle, managing state diligently, and crafting a modular approach to your UI. Keep experimenting with your concepts, and you will be well on your way to creating exceptional Android applications.
For any questions or to share your experiences with Fragments, feel free to leave comments below!
By breaking down and understanding the Fragment lifecycle, and implementing the tips provided, developers can dramatically improve their multi-screen Android applications. Happy coding!