Sorting Lists with Null Values: A Comparator Guide
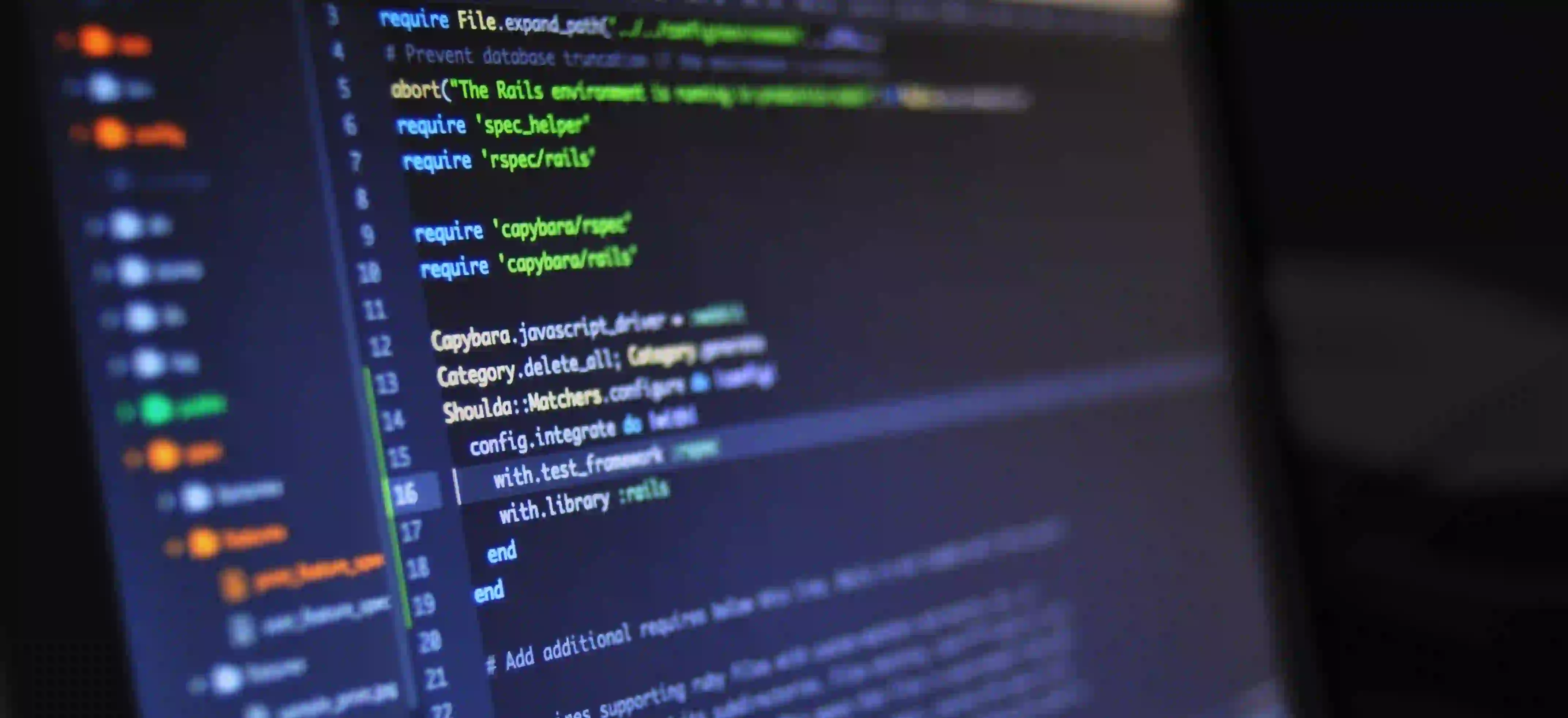
Sorting Lists with Null Values: A Comparator Guide in Java
When working with data in Java, you often need to sort lists. However, what happens when your lists contain null values? Sorting null values requires special consideration, as they can lead to unexpected behavior and exceptions if not handled properly. This blog post will guide you on using comparators effectively to manage null elements during the sorting process.
Understanding Comparators
In Java, a Comparator
is an interface that defines a method for comparing two objects. This interface is particularly useful when you want to customize the natural ordering of a list. Let's start by breaking down how we can use a comparator for sorting lists with nulls.
Basic Syntax of a Comparator
Here's the basic syntax for implementing a Comparator:
import java.util.Comparator;
class Employee {
String name;
public Employee(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
class EmployeeComparator implements Comparator<Employee> {
@Override
public int compare(Employee e1, Employee e2) {
return e1.getName().compareTo(e2.getName());
}
}
In the above code:
- The
Employee
class has a name. - The
EmployeeComparator
class implementsComparator<Employee>
, allowing custom sorting based on employee names.
Sorting Lists with Null Values
When you sort lists containing null values, you need to define how those nulls should be treated. By default, attempting to sort an array with null values using natural order will lead to a NullPointerException
.
Defining Null Handling in Comparators
You can build your comparator to handle null values gracefully. Here’s an example that demonstrates both allowing nulls as greater than and treating them as lesser than:
Handling Nulls as Lesser Than
import java.util.*;
public class NullHandlingExample {
public static void main(String[] args) {
List<Employee> employees = Arrays.asList(
new Employee("Alice"),
null,
new Employee("Bob"),
new Employee("Charlie"),
null
);
Collections.sort(employees, new Comparator<>() {
@Override
public int compare(Employee e1, Employee e2) {
if (e1 == null && e2 == null) return 0;
if (e1 == null) return -1; // Null is lesser
if (e2 == null) return 1; // Null is lesser
return e1.getName().compareTo(e2.getName());
}
});
employees.forEach(e -> System.out.println(e != null ? e.getName() : "null"));
}
}
Explanation of the Comparator Logic
- Check for Nulls: This comparator first checks if either of the employees is null.
- Order of Nulls: If both are null, it returns 0, indicating equality. If the first employee is null, it returns -1, making null treated as lesser. If the second employee is null, it returns 1.
- Comparison: If both are not null, it proceeds to compare their names.
When executed, this code will output:
null
Alice
Bob
Charlie
null
The code sorts the names while placing null values at the beginning.
Handling Nulls as Greater Than
If you would like to prioritize null values and place them at the end of the list, you can modify your comparator like this:
Collections.sort(employees, new Comparator<>() {
@Override
public int compare(Employee e1, Employee e2) {
if (e1 == null && e2 == null) return 0;
if (e1 == null) return 1; // Null is greater
if (e2 == null) return -1; // Null is greater
return e1.getName().compareTo(e2.getName());
}
});
In this scenario, when executed, the sorted output will look like this:
Alice
Bob
Charlie
null
null
Java 8 and Beyond: Lambda Expressions
Starting with Java 8, you can use lambda expressions to write more concise comparator implementations. Here's how to achieve the same sorting using a lambda:
Collections.sort(employees,
(e1, e2) -> {
if (e1 == null && e2 == null) return 0;
if (e1 == null) return 1; // Null is greater
if (e2 == null) return -1; // Null is greater
return e1.getName().compareTo(e2.getName());
});
Code (with Lambda Explanation)
Using a lambda expression simplifies the comparator definition—reducing boilerplate code and enhancing readability. The logic remains unchanged, but now it is more succinct and easy to follow.
Using Comparator.nullsFirst()
and Comparator.nullsLast()
Java also provides built-in methods in the Comparator interface to handle nulls elegantly. You can use Comparator.nullsFirst() and Comparator.nullsLast() to quickly define how null values should be sorted:
Collections.sort(employees,
Comparator.comparing(Employee::getName, Comparator.nullsLast(Comparator.naturalOrder())));
Brief Explanation
This method chain first sorts the employees by name while treating null values as greater than non-null values. This makes it easy to maintain readability and minimize errors.
My Closing Thoughts on the Matter
Sorting lists that contain null values poses unique challenges. However, by leveraging Java's Comparator
interface, including custom comparators or built-in static methods, you can effectively handle nulls according to your specific requirements.
Understanding how to manage null values can make your Java applications safer and more robust. The examples provided here demonstrate how to implement effective sorting mechanisms while maintaining clarity in your code.
If you wish to dive deeper into the world of Java collections, check out Oracle's Collections Framework documentation. Happy coding!