Abstract Class vs Interface: Choosing the Right One in Java
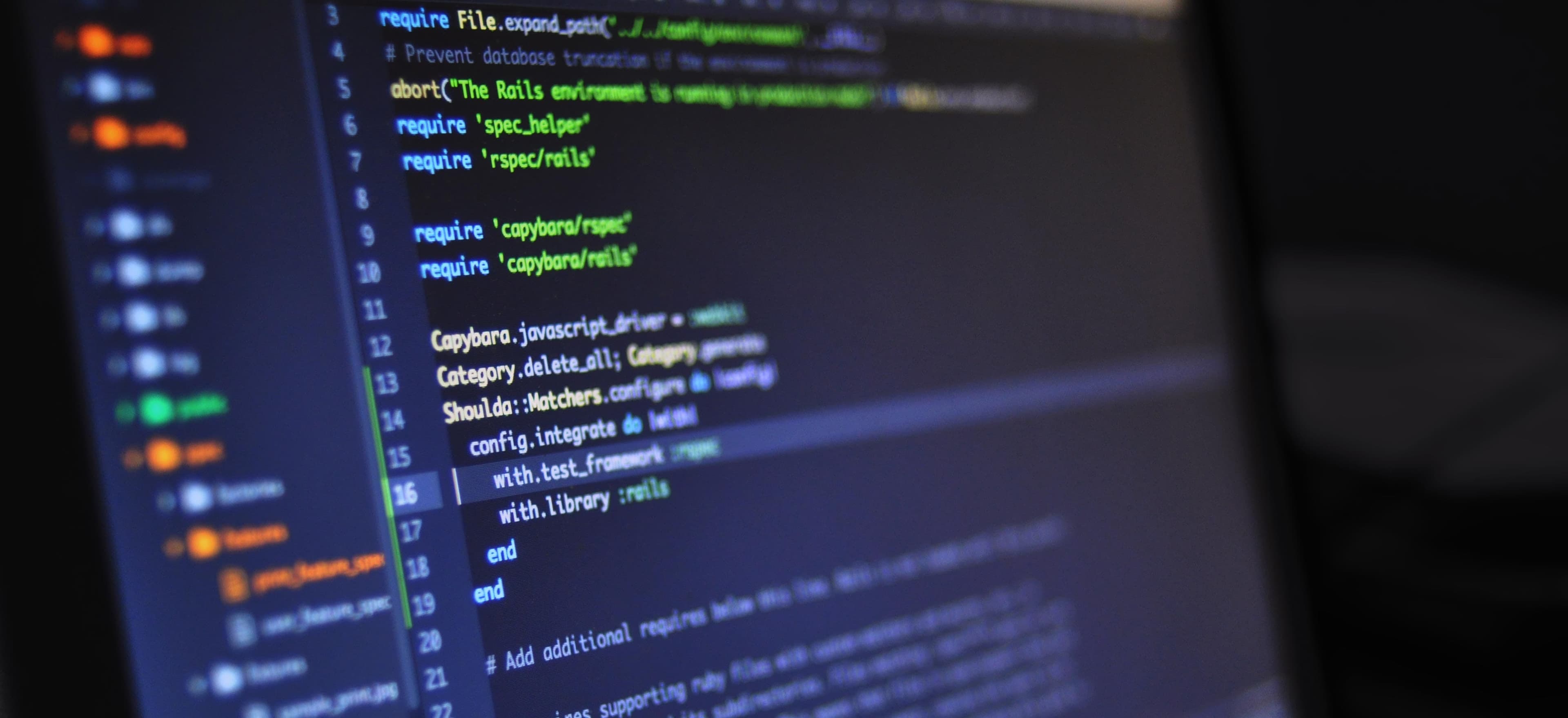
- Published on
Abstract Class vs Interface: Choosing the Right One in Java
When diving into object-oriented programming in Java, understanding the differences between abstract classes and interfaces is a foundational concept. Each serves its own unique purpose, providing developers with powerful tools to structure their code effectively. In this blog post, we will explore the nuances of these constructs, when to use each one, and provide you with illustrative code snippets for better clarity.
What is an Abstract Class?
An abstract class is a class that cannot be instantiated on its own and may contain abstract methods (methods without a body) as well as concrete methods (methods with implementation). Abstract classes are often used when you want to provide a common base for several related classes.
Use Cases for Abstract Classes:
- Common Base Functionality: When several classes share common behaviors or properties.
- Partial Implementation: When you want to provide some method implementations while leaving others to the subclasses.
- State Management: Abstract classes can have state (attributes) that can be inherited.
Example of an Abstract Class
Consider the following example where we define an abstract class Animal
.
abstract class Animal {
private String name;
public Animal(String name) {
this.name = name;
}
public String getName() {
return name;
}
abstract void sound(); // Abstract method
}
class Dog extends Animal {
public Dog(String name) {
super(name);
}
@Override
void sound() {
System.out.println("Woof!");
}
}
class Cat extends Animal {
public Cat(String name) {
super(name);
}
@Override
void sound() {
System.out.println("Meow!");
}
}
In the above code, Animal
serves as a base class for Dog
and Cat
. Each subclass implements the sound
method while inheriting the name
property and the getName
method from Animal
. This demonstrates how abstract classes allow for partial implementation and shared state.
What is an Interface?
An interface is a contract that defines a set of methods without providing their implementation. Classes implementing an interface must provide concrete implementations for all of its methods. Interfaces are ideal for defining capabilities that can be shared among unrelated classes.
Use Cases for Interfaces:
- Multiple Inheritance: Java allows a class to implement multiple interfaces, which can be useful for supporting multiple behaviors.
- Decoupling: Interfaces provide a way to decouple code; you can change implementations without altering the code that consumes the interface.
- Event Handling: Interfaces are commonly used for callback mechanisms in event-driven programming.
Example of an Interface
Now consider an example where we define an interface Playable
.
interface Playable {
void play();
}
class Piano implements Playable {
@Override
public void play() {
System.out.println("Playing the piano.");
}
}
class Guitar implements Playable {
@Override
public void play() {
System.out.println("Strumming the guitar.");
}
}
In this scenario, Playable
is an interface that defines a behavior—all classes that implement it must provide their version of the play
method. The Piano
and Guitar
classes showcase how different classes can share behaviors through interfaces.
Key Differences Between Abstract Classes and Interfaces
1. Instantiation:
- Abstract classes cannot be instantiated directly.
- Interfaces also cannot be instantiated.
2. Method Implementation:
- Abstract classes can have both abstract and concrete methods.
- Interfaces can only have abstract methods (until Java 8, which allows default and static methods).
3. State:
- Abstract classes can have instance variables and constructors that help to maintain state.
- Interfaces cannot have instance variables (they can have constants).
4. Inheritance:
- A class can extend only one abstract class.
- A class can implement multiple interfaces, which offers more flexibility.
5. Use Cases:
- Use abstract classes when you want to share code among closely related classes.
- Use interfaces when you want to define a contract for classes that may be unrelated.
When to Choose What?
The choice between abstract classes and interfaces often depends on the specific requirements of your application. Here are some guiding principles:
- Complex Hierarchy with Shared State: If you are designing a complex class hierarchy where subclasses have a lot in common, an abstract class is appropriate.
- Unrelated Classes with Shared Behavior: Use interfaces if the classes do not share a common parent but must adhere to the same contract—this is particularly useful in a large codebase with different modules that independently implement certain functionalities.
Real-World Use Cases
In a large-scale application, consider this hypothetical scenario: you're building a multimedia application that supports different media types (audio, video, etc.).
- You could create an abstract class
Media
to provide common features (likeplay()
,pause()
, orstop()
) shared across various media types, enforcing that every media class will have these methods. - You may want to implement an interface like
Recordable
for classes that can record, making the recording functionality available to multiple media types without sharing a base class.
Here’s how this might look:
abstract class Media {
public abstract void play();
public abstract void pause();
}
interface Recordable {
void record();
}
class Audio extends Media implements Recordable {
public void play() {
System.out.println("Playing audio.");
}
public void pause() {
System.out.println("Pausing audio.");
}
public void record() {
System.out.println("Recording audio.");
}
}
In this design, Audio
extends Media
, inheriting its capabilities while also implementing the Recordable
interface, fulfilling both needs of shared and disparate functionalities.
Lessons Learned
Both abstract classes and interfaces are vital tools in Java's object-oriented programming arsenal. Understanding when to utilize each can significantly enhance code organization, reusability, and overall design clarity. So, the next time you are faced with designing a class structure in Java, consider these key differences and guidelines to make an informed decision.
For further reading, check out the Java Documentation on Interfaces and the detailed Java Abstract Classes Guide.
Feel free to explore the above examples in your own Java IDE to better grasp how abstract classes and interfaces work together to create robust, maintainable code. Happy coding!