Overcoming Common Kafka Integration Issues with Apache Flink
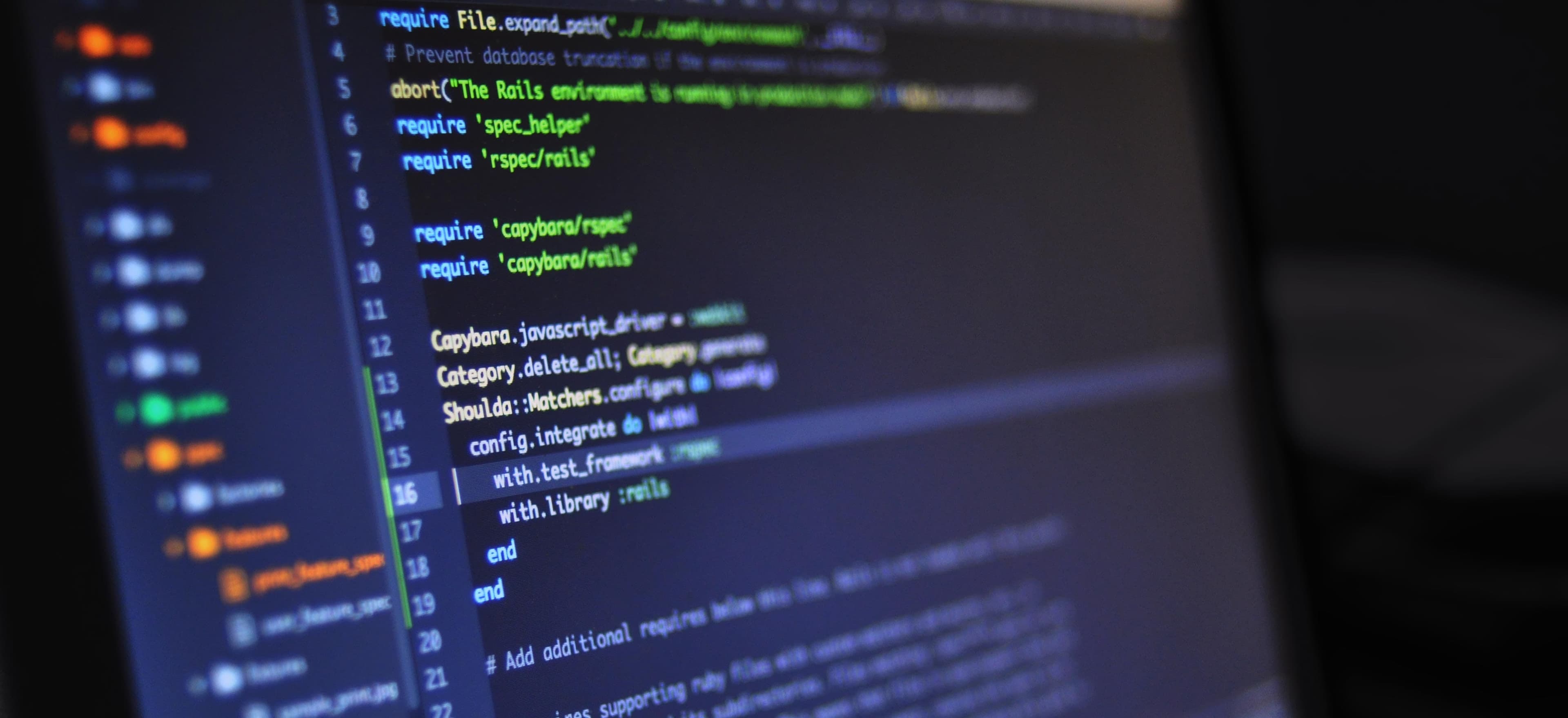
- Published on
Overcoming Common Kafka Integration Issues with Apache Flink
Apache Kafka is a popular platform for building real-time data pipelines. However, integrating Kafka with data processing frameworks such as Apache Flink can introduce specific challenges. In this blog post, we will explore some common Kafka integration issues with Flink and how to overcome them effectively.
Understanding Kafka and Flink
Before diving into the integration concerns, let’s clarify the roles of Kafka and Flink.
-
Apache Kafka: A distributed messaging system that can handle real-time data feeds. It is designed for high-throughput and fault tolerance, making it an excellent choice for event-driven architectures.
-
Apache Flink: A stream processing framework for big data analytics. Flink is known for its event-driven capabilities and supports batch processing as well.
The synergy between Kafka and Flink allows for powerful real-time data processing solutions, but there are specific pitfalls to be mindful of.
Setup and Configuration
Installing Kafka and Flink: Before we address integration issues, install both components. You can follow the official Kafka documentation and Flink documentation for detailed installation instructions.
Next, you need to configure Kafka and Flink to facilitate seamless integration.
Code Snippet: Configuring Flink to Consume from Kafka
Below is a simple Java code snippet that demonstrates how to set up a Flink job to consume messages from a Kafka topic.
import org.apache.flink.api.common.serialization.SimpleStringSchema;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.connectors.kafka.FlinkKafkaConsumer;
import java.util.Properties;
public class KafkaFlinkIntegration {
public static void main(String[] args) throws Exception {
// Set up the execution environment
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
// Kafka Consumer properties
Properties properties = new Properties();
properties.setProperty("bootstrap.servers", "localhost:9092");
properties.setProperty("group.id", "test");
// Create a Kafka Consumer
FlinkKafkaConsumer<String> consumer = new FlinkKafkaConsumer<>(
"my-topic",
new SimpleStringSchema(),
properties
);
// Add the consumer as a source to the environment
env.addSource(consumer).print();
// Execute the Flink job
env.execute("Kafka Flink Integration");
}
}
Commentary on the Code
In the code snippet above, we start by setting up the Flink execution environment. You always need this as the backbone of your Flink job. The properties for the Kafka Consumer include the bootstrap.servers
(the Kafka broker address) and group.id
(to identify the consumer group).
This integration exemplifies a key aspect of Flink: its ability to manage stream data effortlessly. Now, let’s discuss common issues that arise with this integration.
Common Kafka Integration Issues
1. Connection Issues to Kafka Broker
A frequent problem developers face is establishing a connection to the Kafka Broker. This can happen for various reasons:
-
Incorrect Bootstrap Server Configuration: Ensure that the correct IP address and port are set (default is usually 9092).
-
Kafka Not Running: Verify that the Kafka server is running. You can start it using the command:
bin/kafka-server-start.sh config/server.properties
- Network Issues: Check firewall settings or security groups if Kafka is hosted in the cloud.
2. Serialization and Deserialization Problems
Messages in Kafka are stored as byte arrays. Flink must know how to serialize and deserialize these messages.
The previously mentioned use of SimpleStringSchema
works when you are dealing with string messages. If your data format is JSON or Avro, you might need custom schemata.
Code Snippet: Custom Serialization
Here’s how to implement a custom deserialization schema for handling JSON:
import org.apache.flink.api.common.serialization.DeserializationSchema;
import java.io.IOException;
public class MyJsonDeserializationSchema implements DeserializationSchema<MyData> {
@Override
public MyData deserialize(byte[] bytes) throws IOException {
// Implement logic to deserialize JSON into MyData object
}
@Override
public boolean isEndOfStream(MyData nextElement) {
return false;
}
@Override
public TypeInformation<MyData> getProducedType() {
return TypeExtractor.getForClass(MyData.class);
}
}
Commentary on Custom Serialization
This code defines a custom deserialization schema, allowing Flink to process complex data types seamlessly. Effective serialization ensures data integrity throughout the pipeline.
3. Performance and Throughput Issues
While Kafka and Flink are designed for high throughput, performance can dwindle if not appropriately configured.
- Parallelism: Flink allows setting parallelism levels that can help scale the consumption of Kafka messages.
env.setParallelism(4); // Adjust based on your use case
- Kafka Topic Partitioning: Ensure your Kafka topic is partitioned adequately. More partitions lead to better parallel processing in Flink.
4. Handling Backpressure
Backpressure occurs when the data flow rate in your pipeline exceeds the processing rate. This can result in increased latency or even failure of the Flink job.
To handle backpressure:
-
Buffer Sizes: Monitor and tweak buffer sizes both in Kafka and Flink.
-
Async I/O: Employ asynchronous I/O for any external call Flink makes (like to databases or external services).
Best Practices for Kafka and Flink Integration
-
Monitor Your Metrics: Utilize monitoring tools like Prometheus and Grafana to visualize performance and latency.
-
Implement Error Handling: Use checkpointing and savepoints to maintain consistency and handle failures gracefully.
-
Manage Schema Evolution: When using data formats like Avro, ensure versioning strategies to accommodate schema changes.
Additional Resources
For further reading on the topics discussed, please refer to:
- The official Apache Flink Documentation for comprehensive integration examples.
- An insightful medium article that dives deep into advanced Kafka Flink configurations.
To Wrap Things Up
Integrating Apache Kafka with Apache Flink can significantly enhance your streaming data capabilities. While you may encounter challenges ranging from connection issues to performance constraints, understanding these pitfalls and applying best practices will empower you to build robust data processing applications.
By leveraging the right configurations, ensuring proper serialization mechanisms, and adhering to performance best practices, you can fully harness the potential of both Kafka and Flink in your real-time data processing workflows.
If you have any questions or experiences to share, feel free to comment below! Happy coding!
Checkout our other articles