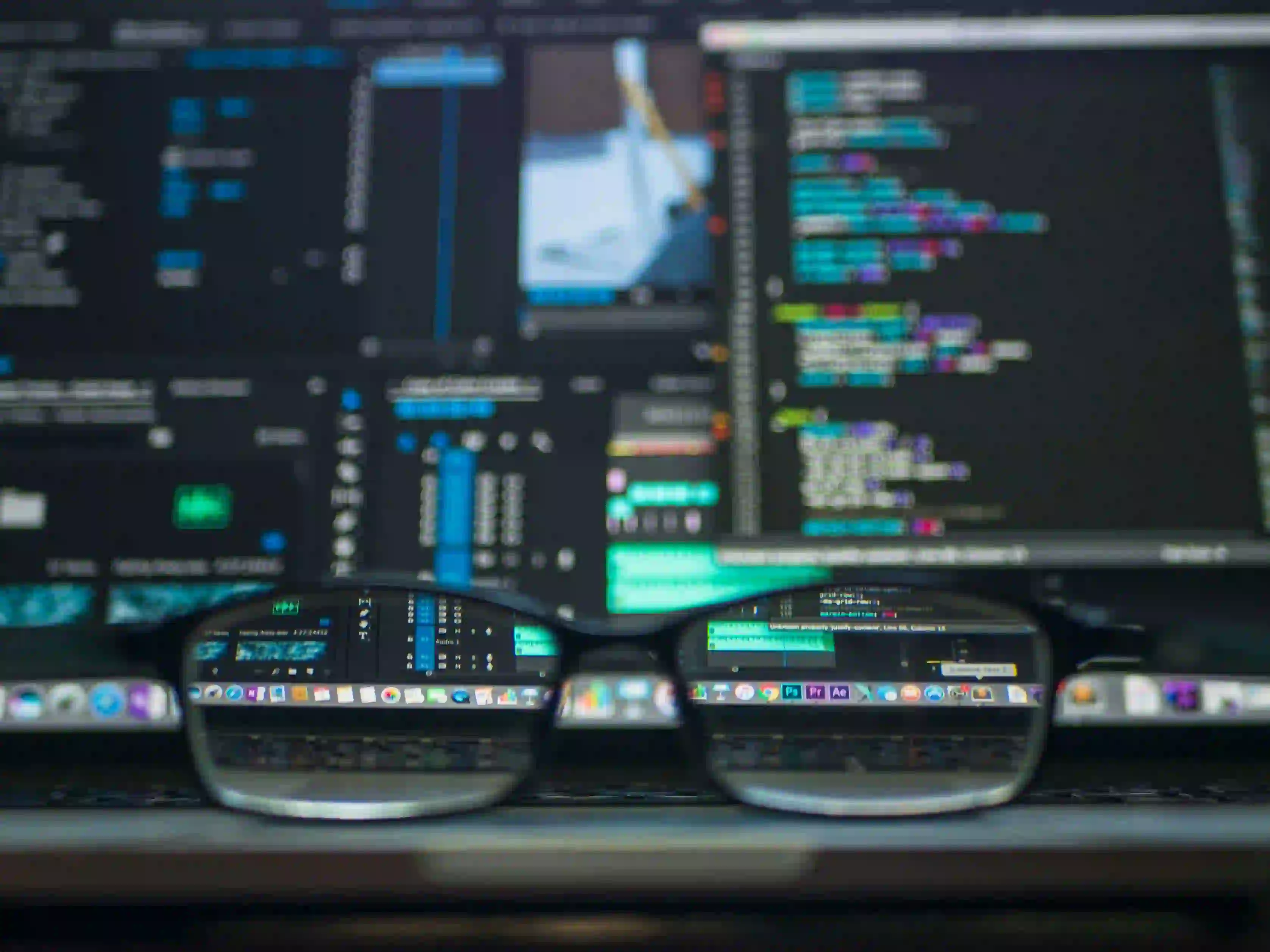
Mastering Shake to Refresh: Avoiding User Frustration
In modern mobile app development, user experience (UX) is a key consideration. Among the myriad of features available, one standout interaction is the "Shake to Refresh" functionality. However, improperly implementing this feature can lead to user frustration. In this blog post, we will explore how to effectively master the Shake to Refresh functionality in your Java applications while ensuring a delightful user experience.
What is Shake to Refresh?
Shake to Refresh is an intuitive way for users to refresh content in an app simply by shaking their device. While it can enhance interactivity, it can also easily become a source of annoyance if not optimized correctly. Users expect features like this to work seamlessly and predictably.
The Importance of Context
Before diving into the implementation details, it's critical to understand the context in which this feature is being used. Are users likely to shake their devices? What content are they expecting to refresh? Understanding your application's use case helps you design the Shake to Refresh feature appropriately.
Implementation Overview
To implement Shake to Refresh in a Java application, particularly for Android, we can use the SensorManager
class to detect shake gestures. Below, we'll walk through a simple implementation and discuss how to enhance user experience.
Step 1: Setting Up Your Android Project
If you're working on an Android project, make sure to include the necessary dependencies in your build.gradle
file. You'll need the AndroidX libraries for sensors:
dependencies {
implementation 'androidx.appcompat:appcompat:1.3.0'
implementation 'androidx.core:core-ktx:1.6.0'
}
Step 2: Creating the Shake Detection Logic
We'll set up shake detection using the accelerometer. The basic idea is to measure the acceleration of the device and determine when it has been shaken. Here’s a simple implementation:
import android.content.Context;
import android.hardware.Sensor;
import android.hardware.SensorEvent;
import android.hardware.SensorEventListener;
import android.hardware.SensorManager;
public class ShakeDetector implements SensorEventListener {
private static final float SHAKE_THRESHOLD = 15.0f;
private SensorManager sensorManager;
private Sensor accelerometer;
private OnShakeListener onShakeListener;
public ShakeDetector(Context context, OnShakeListener listener) {
sensorManager = (SensorManager) context.getSystemService(Context.SENSOR_SERVICE);
accelerometer = sensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER);
this.onShakeListener = listener;
}
public void start() {
sensorManager.registerListener(this, accelerometer, SensorManager.SENSOR_DELAY_NORMAL);
}
public void stop() {
sensorManager.unregisterListener(this);
}
@Override
public void onSensorChanged(SensorEvent event) {
float x = event.values[0];
float y = event.values[1];
float z = event.values[2];
float acceleration = (float) Math.sqrt(x * x + y * y + z * z);
if (acceleration > SHAKE_THRESHOLD && onShakeListener != null) {
onShakeListener.onShake();
}
}
@Override
public void onAccuracyChanged(Sensor sensor, int accuracy) { }
public interface OnShakeListener {
void onShake();
}
}
Code Explanation
- Contextual Initialization: We set up a
SensorManager
and register the accelerometer listener. - Shake Detection: The
onSensorChanged
method applies a basic calculation to determine if the acceleration exceeds the defined threshold, indicating a shake. - Listener Interface: We use an interface (
OnShakeListener
) for callbacks so that the activity can define what happens during a shake.
Step 3: Integrating Shake Detection
Now we’ll integrate the ShakeDetector
into an Android activity. Here’s how you can do this:
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private ShakeDetector shakeDetector;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
shakeDetector = new ShakeDetector(this, new ShakeDetector.OnShakeListener() {
@Override
public void onShake() {
// Refresh content
refreshContent();
Toast.makeText(MainActivity.this, "Content Refreshed", Toast.LENGTH_SHORT).show();
}
});
}
@Override
protected void onResume() {
super.onResume();
shakeDetector.start();
}
@Override
protected void onPause() {
super.onPause();
shakeDetector.stop();
}
private void refreshContent() {
// Logic to refresh content
}
}
Key Points
- The
ShakeDetector
is initialized inonCreate
, binding the shake event to the content refresh method. - The registration and unregistration of the sensor listener in
onResume
andonPause
prevent unnecessary battery drain and enhance performance.
Tips for Enhancing User Experience
While the above implementation provides a functional Shake to Refresh feature, consider the following tips to avoid user frustration:
1. Sensitivity Control
Allow users to adjust the sensitivity of the shake detection. This customization caters to varying preferences and reduces false triggers.
2. Visual Feedback
Always provide visual feedback upon a shake event, such as a loading spinner. This reassurance confirms that the app is processing the user's request.
private void refreshContent() {
progressBar.setVisibility(View.VISIBLE);
// Logic to fetch new content goes here
// Hide progressBar when done
}
3. Avoiding Conflicts
Ensure that shake detection doesn’t conflict with other gestures or inputs. If your app uses other motion-sensitive features, you may want to implement a way to disable shake detection contextually.
4. User Education
Educate users about the feature through onboarding or prompts. Not all users are familiar with Shake to Refresh, and a gentle introduction can improve engagement.
5. Handle Errors Gracefully
Ensure error messages are clear and informative when fetching new content fails. Avoid technical jargon and suggest actionable steps for users.
Closing the Chapter
Mastering the Shake to Refresh feature not only enhances engagement but also reinforces a fluid and dynamic user experience. By implementing proper detection mechanisms, offering customization options, and providing visual feedback, you can avoid user frustration and elevate your app’s usability.
For further reading, you can check these resources for more tips on enhancing user experience in mobile applications:
- Android Developer Guidelines
- Optimizing User Experience
By keeping user needs and expectations at the forefront of your development process, you'll foster a more enjoyable app experience. Happy coding!