Common SSL Issues When Deploying Java Microservices with Nginx
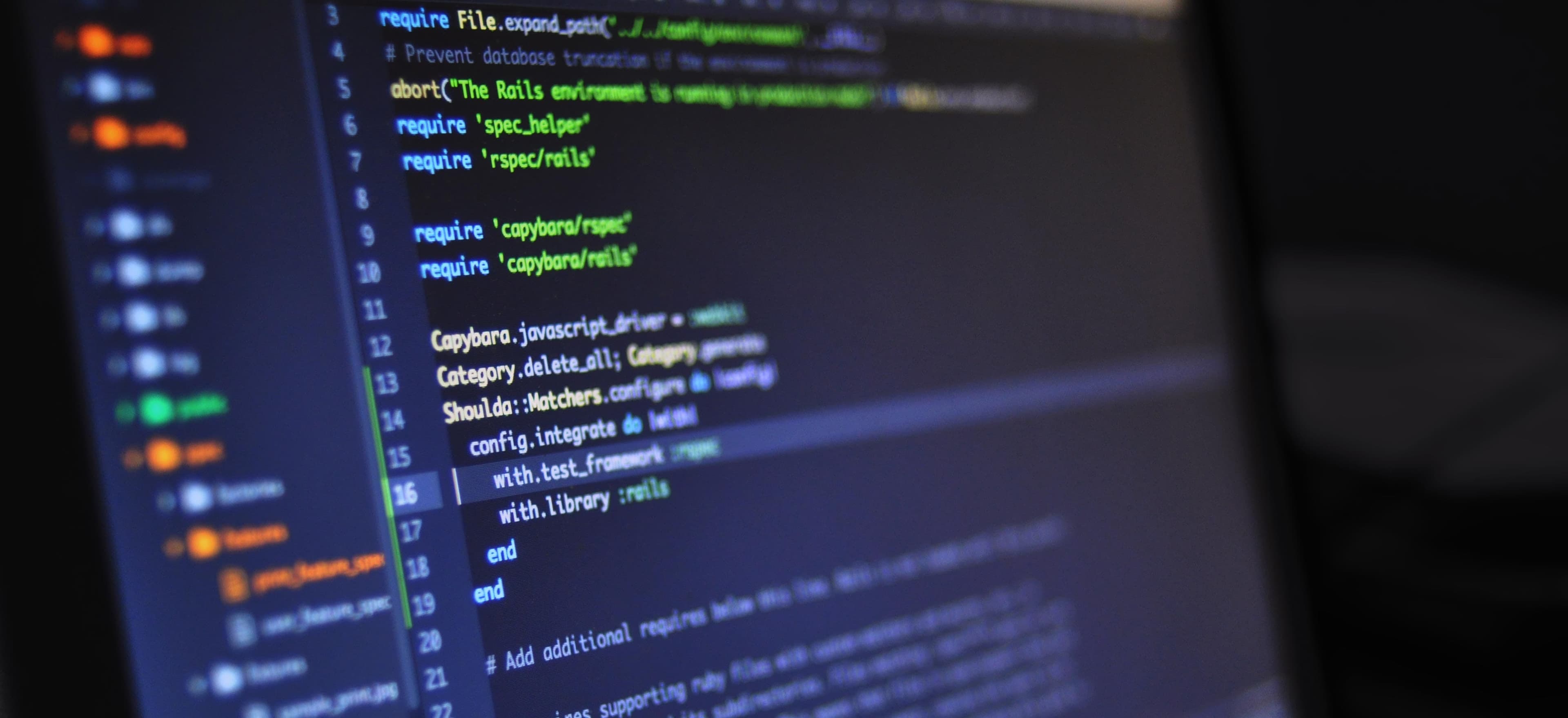
- Published on
Common SSL Issues When Deploying Java Microservices with Nginx
In today's digital landscape, securing your application with SSL (Secure Sockets Layer) is paramount, especially when deploying Java microservices. When using Nginx as a reverse proxy, integrating SSL certificates properly is essential for maintaining data integrity and confidentiality. In this blog post, we’ll explore common SSL issues faced during the deployment of Java microservices with Nginx, how to diagnose them, and provide practical solutions.
Why SSL Matters for Microservices
SSL protects sensitive data transmitted between clients (like browsers) and servers. With the rise of microservices architecture, where applications are broken into smaller, independently deployable services, each service often requires its own SSL configuration. This ensures that all inter-service communications are encrypted.
Setting Up Nginx with SSL
Before diving into common issues, let's quickly cover how to set up Nginx with SSL. Here’s a straightforward way to configure Nginx to handle SSL:
Nginx Configuration Snippet
server {
listen 443 ssl;
server_name yourdomain.com;
ssl_certificate /etc/ssl/certs/your_certificate.crt;
ssl_certificate_key /etc/ssl/private/your_private.key;
location /api {
proxy_pass http://localhost:8080; # Point to your Java microservice
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
Commentary on the Code
server { listen 443 ssl; }
: This line specifies that the server will listen for SSL traffic on port 443.ssl_certificate
andssl_certificate_key
: These directives are essential for defining your SSL certificate and key path. Misconfiguration here is a common source of errors.proxy_pass
: This directive is where you specify the backend service, like a Spring Boot application running on port 8080.
Common SSL Issues
1. Certificate Not Trusted
One of the most frequent issues is that clients don’t trust the SSL certificate being used. This often happens with self-signed certificates.
Solution: Use a trusted Certificate Authority (CA). For development, you might choose to use self-signed certificates, but ultimately, production should utilize certificates from recognized CAs such as Let's Encrypt for free SSL certificates.
2. Mixed Content Errors
When your website is served over HTTPS but loads resources (like scripts, stylesheets, or images) over HTTP, browsers flag these as mixed content issues.
Solution: Always load resources over HTTPS. Update your Java microservice configuration to ensure API calls and resource links use HTTPS.
3. Incorrect Certificate Chain
If the server does not send the full certificate chain (particularly intermediate certificates), browsers may reject the certificate.
Solution: Ensure that you include the full chain in your Nginx configuration.
ssl_certificate /etc/ssl/certs/your_fullchain.pem; # Use fullchain
4. Expired Certificates
SSL certificates have expiration dates. An expired certificate will prevent users from accessing your microservices.
Solution: Implement a monitoring system to alert you when your SSL certificates are nearing expiration. Tools like Certbot, which automates the renewal process for Let's Encrypt, can help.
5. Client-Side Configuration Issues
Sometimes, the error comes from the client, especially if they're using outdated browsers or have their own SSL issues (e.g., missing root certificates).
Solution: Ensure compatibility by recommending your users upgrade their browsers or check their local machine’s certificate store.
Debugging SSL Issues
When an SSL issue arises, debugging is crucial. Here are some useful tools and commands:
1. OpenSSL Command
You can use OpenSSL to test your SSL certificate:
openssl s_client -connect yourdomain.com:443
This command provides detailed information about the SSL handshake process. Look for issues like certificate chain errors.
2. Nginx Logs
Nginx's error logs can be invaluable when resolving SSL issues. Check the logs usually found at:
/var/log/nginx/error.log
3. Browser Developer Tools
Utilize the Developer Tools in browsers (F12) to investigate errors shown in the console. Look under the "Security" tab to see certificate details.
Bringing It All Together
Integrating SSL into your Java microservices architecture using Nginx is vital for protecting sensitive data. Understanding common SSL issues and their solutions can save you much troubleshooting time.
Whether you’re using trusted certificates from a well-known CA or self-signed certificates for development, always ensure that your SSL configurations are sound. Remember to stay ahead of issues by implementing regular monitoring and renewal practices.
Further Reading
If you're interested in a deeper dive into microservices architecture and security considerations, here are some recommended resources:
- Java Microservices
- Nginx Documentation
- SSL Best Practices
Happy coding! If you have questions, remember to join community forums or seek advice from experts to enhance your SSL deployment processes.
Checkout our other articles