Mastering Live Templates in IntelliJ IDEA: Boost Your Coding!
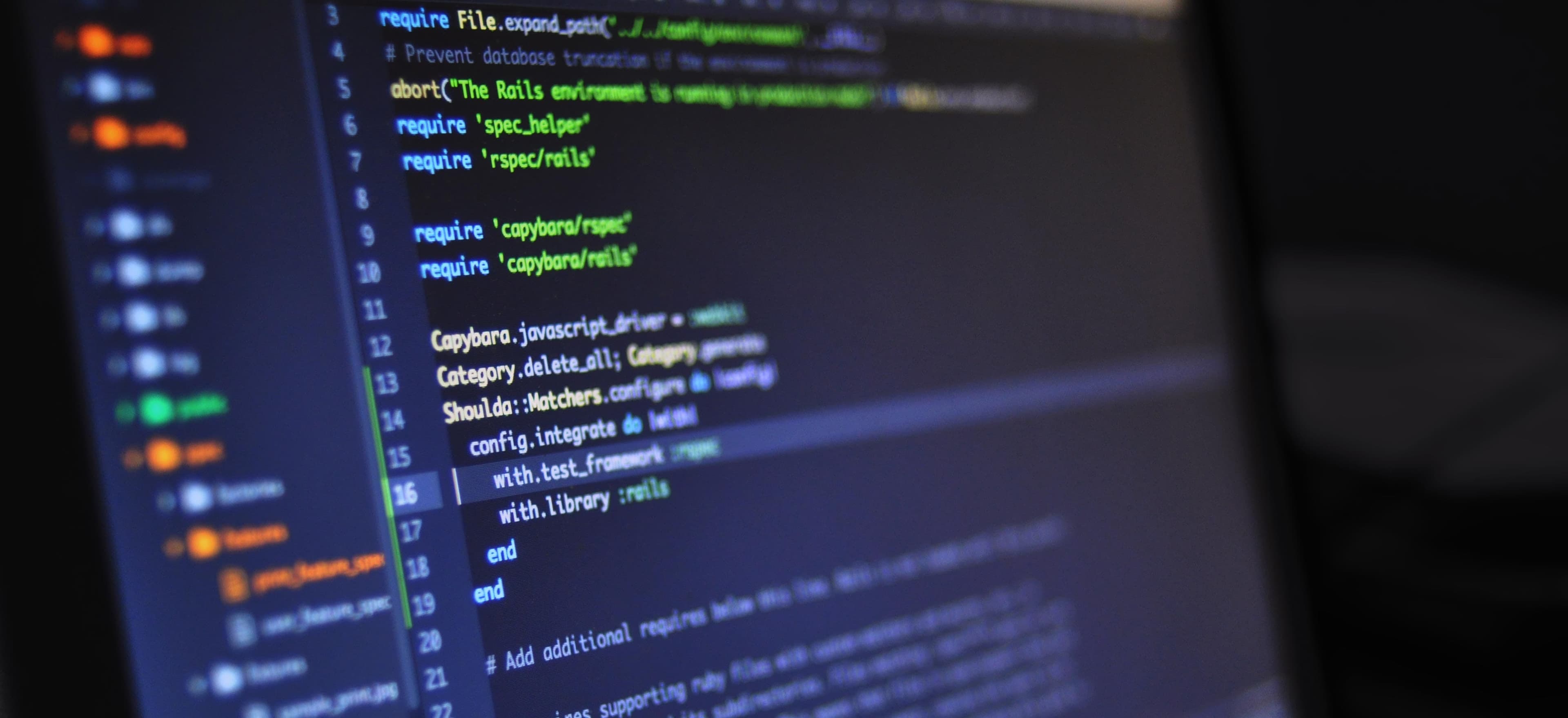
- Published on
Mastering Live Templates in IntelliJ IDEA: Boost Your Coding!
IntelliJ IDEA is not just a powerful Integrated Development Environment (IDE) for Java development; it's a toolkit that can drastically enhance your coding efficiency. One of its most underrated features is the Live Templates functionality. In this post, we will dive deep into Live Templates, how to create and use them effectively, and why you should incorporate them into your daily coding routine.
What are Live Templates?
Live Templates are essentially code snippets that allow you to insert frequently used code constructs quickly. These templates can be customized and configured according to your needs, saving you countless keystrokes and time while coding.
For example, instead of typing out an entire for
loop or try-catch block every time, you can create a Live Template that expands to that structure with just a few keystrokes.
Why Use Live Templates?
- Time-Saving: You can insert code with minimal effort, reducing repetitive typing.
- Consistency: Templates help enforce coding standards, as the same structure will be used across your codebase.
- Focus on Logic: With less boilerplate to worry about, you can concentrate on the logic of your application.
Creating Your First Live Template
Let's walk through the steps of creating your first Live Template in IntelliJ IDEA.
-
Open IntelliJ IDEA.
-
Go to Settings: Navigate to File > Settings (or Preferences on macOS).
-
Select Live Templates: In the settings window, go to Editor > Live Templates.
-
Add a New Template: Click the "+" button and choose "Template Group" to create a new group if you want to organize your templates or directly add a new template.
-
Define the Template: In the template editing area, enter your snippet. For instance, let's create a template for a
for
loop:for (int $index$ = 0; $index$ < $limit$; $index$++) { $END$ }
Here,
$index$
and$limit$
are variables that will be replaced when you invoke the template.$END$
is a placeholder that moves the cursor to the end of the template after expansion. -
Set the Context: Specify where the template can be used. For a Java for loop, choose "Java" as the applicable context.
-
Save Your Template: Click OK to save your changes.
Code Snippet Explanation
The above code represents a for
loop template. Here's the breakdown:
$index$
: A variable to represent the loop index.$limit$
: A variable to specify the end condition for the loop.$END$
: A point where the cursor stops after template expansion, allowing you to start typing immediately.
To invoke your new Live Template, type the abbreviation you assigned and hit the Tab
key – voila, you have a ready-to-go for
loop!
Advanced Features of Live Templates
1. Adding Conditions
You can create templates that become available only in certain contexts. For example, if you want that for
loop to appear only in "main" methods:
#if ($METHOD_NAME$ == "main")
for (int $index$ = 0; $index$ < $limit$; $index$++) {
$END$
}
#end
This ensures that your template remains relevant to the specific scope of your application.
2. Using Expressions
Live Templates also support expressions as a dynamic way to customize the template content based on the context. You can leverage built-in variables like date()
or user()
to automatically fill in values. For instance, to print the current date:
System.out.println("Today is " + date("yyyy-MM-dd") + ".");
3. Setting Default Values
By configuring variable options, you can set default values for template parameters. For instance:
String $name$ = "$name$";
Where $name$
can default to "User" if you leave it unassigned when using the template.
Common Live Templates
1. Try-Catch Block
Here's how you can create a template for a try-catch block:
try {
$END$
} catch ($EXCEPTION_TYPE$ $ex$) {
$ex$.printStackTrace();
}
This simplifies exception handling greatly and encourages proper error management.
2. Getter and Setter
Creating getters and setters for class variables can also be tedious. You can create a template like this:
public $type$ get$NameCapitalized$() {
return $name$;
}
Where $type$
and $name$
can take user input or default values.
3. JUnit Test
Creating boilerplate code for a JUnit test can be efficiently handled through:
@Test
public void $methodName$() {
$END$
}
Instantly creates a method scaffold for a new JUnit test.
Best Practices for Using Live Templates
- Keep It Simple: Avoid over-complicating your templates. They should enhance your coding experience, not make it more cumbersome.
- Categorize Your Templates: Use groups to categorize templates based on functionality, e.g.,
Loops
,Exceptions
,Testing
. - Review and Refine: Regularly check your templates for relevance and effectiveness. Update them based on your experience.
- Share with Your Team: If you’re part of a team, share your Live Templates to maintain consistency and speed across the project.
In Conclusion, Here is What Matters
Live Templates in IntelliJ IDEA are an outstanding feature that can significantly speed up your coding process while maintaining consistency in your code structure. From simple loops to advanced expressions, the flexibility and efficiency offered by Live Templates can help you focus on what truly matters: your application logic.
By incorporating Live Templates into your workflow, you're poised to boost your productivity, lessen repetitive tasks, and ultimately become a more effective developer. So why not give it a go? Explore, create, and watch your coding experience evolve!
For additional information on customizing your IntelliJ IDEA experience, you may want to check out the official JetBrains documentation on Live Templates.
Happy coding!
Checkout our other articles