Troubleshooting JSON Parsing in Your Weather App
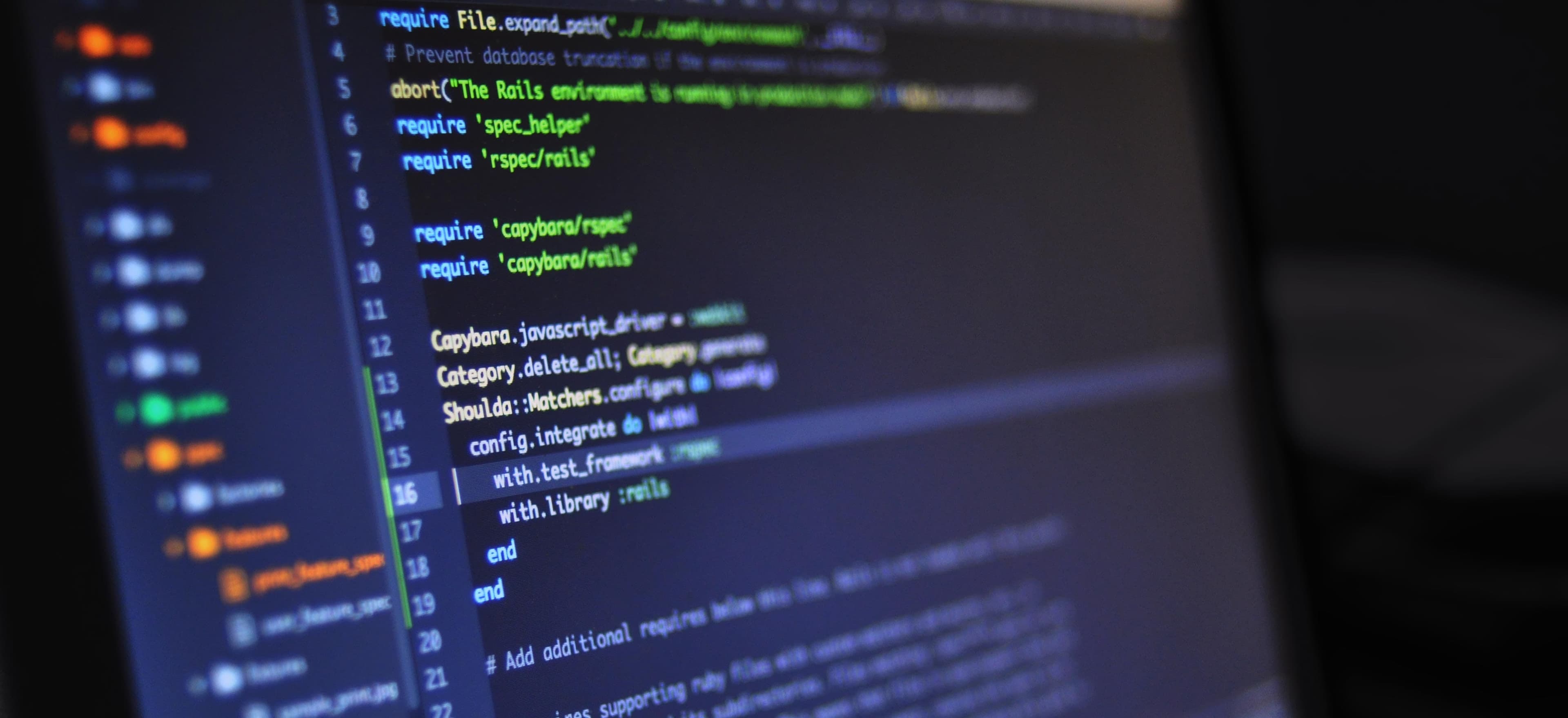
- Published on
Troubleshooting JSON Parsing in Your Weather App
When developing an application that relies on external APIs, such as a weather app that fetches current weather data, JSON (JavaScript Object Notation) becomes your best friend. JSON is a lightweight data interchange format that's easy to read and write for humans and machines alike. However, issues often arise during JSON parsing, which can lead to frustrating bugs and crashes. This blog post will guide you through common pitfalls and solutions for effectively troubleshooting JSON parsing in your weather app.
Why JSON?
JSON is favored for several reasons:
- Lightweight: It has minimal syntax making it easier to read and write.
- Language agnostic: It can be used with various programming languages including Java, JavaScript, Python, etc.
- Support for complex structures: JSON supports nested data structures, which is beneficial for representing real-world data like weather information.
This leads us to the heart of our discussion: effectively managing JSON data within your Java weather app.
Understanding JSON Structure
Before we dive into troubleshooting, let’s understand the JSON format you'll likely deal with in your weather app. A typical weather API might return data structured like this:
{
"location": {
"city": "San Francisco",
"country": "USA"
},
"current": {
"temperature": 18,
"condition": "Sunny",
"humidity": 60
}
}
This structure illustrates how location and weather data are nested. Understanding this is crucial when you parse the JSON.
Common JSON Parsing Libraries in Java
Java offers several libraries for JSON parsing. The most popular include:
- Jackson: A high-performance JSON processor.
- Gson: Google’s library for converting Java objects into JSON and vice versa.
- org.json: A simple library for parsing and encoding JSON.
For this blog, we will focus on Gson due to its ease of use and integration.
// Maven dependency for Gson
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.8</version>
</dependency>
Step-By-Step JSON Parsing in a Weather App
Step 1: Fetching Data
To fetch data, ensure your app correctly makes HTTP requests to the weather API. The following example demonstrates using HttpURLConnection
for this purpose:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class WeatherFetcher {
public String fetchWeather(String apiUrl) throws Exception {
URL url = new URL(apiUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
// Check for request success
if (conn.getResponseCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream()));
StringBuilder output = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
output.append(line);
}
conn.disconnect();
return output.toString();
}
}
Why This Matters: The HTTP response code must be checked to ensure that your request was successful before attempting to parse the JSON. A failure should raise an exception to avoid further complications.
Step 2: Parsing JSON
Once you've fetched your JSON data as a String
, you can parse it into Java objects using Gson:
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class WeatherParser {
public WeatherResponse parseWeather(String jsonData) {
Gson gson = new GsonBuilder().create();
return gson.fromJson(jsonData, WeatherResponse.class);
}
}
Step 3: Defining Your Data Model
You'll need to create classes that mirror the structure of your JSON data. Here's an example:
class WeatherResponse {
Location location;
Current current;
class Location {
String city;
String country;
}
class Current {
int temperature;
String condition;
int humidity;
}
}
Why This Structure: By creating a model that resembles the JSON structure, Gson can automatically map fields, minimizing manual data handling.
Step 4: Handling Parsing Exceptions
It's crucial to handle exceptions that may arise when parsing JSON. Below is an enhanced version of the parseWeather
method that includes error handling:
public WeatherResponse parseWeather(String jsonData) {
Gson gson = new GsonBuilder().create();
try {
return gson.fromJson(jsonData, WeatherResponse.class);
} catch (JsonSyntaxException e) {
System.err.println("Error parsing JSON: " + e.getMessage());
return null;
}
}
Why You Need Exception Handling: JSON structures can vary, leading to exceptions when fields are missing or wrongly typed. Handling these exceptions gracefully can prevent crashes and enhance user experience.
Step 5: Validating JSON Structure
Sometimes the JSON structure might be different from what you expect. It's helpful to validate the JSON response before parsing. You can use the org.json
library to do this:
import org.json.JSONObject;
public boolean validateJson(String jsonData) {
try {
JSONObject json = new JSONObject(jsonData);
// Check for necessary fields
return json.has("location") && json.has("current");
} catch (Exception e) {
System.err.println("Invalid JSON: " + e.getMessage());
return false;
}
}
Why Validation Matters: This proactive approach helps in identifying errors early in the flow, allowing for graceful degradation of your app’s functionality.
Best Practices for JSON Parsing
- Keep Your Models Simple: Complex models can lead to deserialization issues.
- Use Optional Fields: If certain JSON fields might be absent, consider using
Optional
in your models. - Log Errors: Make sure to log any errors encountered during data retrieval and parsing to ease debugging.
- Test with Various Scenarios: Test your app against a variety of JSON structures to ensure stability.
To Wrap Things Up
Troubleshooting JSON parsing in your weather app can be simplified with a clear understanding of JSON structures and best practices in Java. By implementing robust error handling, validation, and effective data models, you will not only improve the reliability of your app but also enhance user experience.
Have you faced any JSON parsing issues in your development journey? Share your thoughts in the comments below! For further reading on handling JSON data in Java, check out the official Gson documentation.
Happy coding!
Checkout our other articles