Common Pitfalls When Creating Custom Hamcrest Matchers
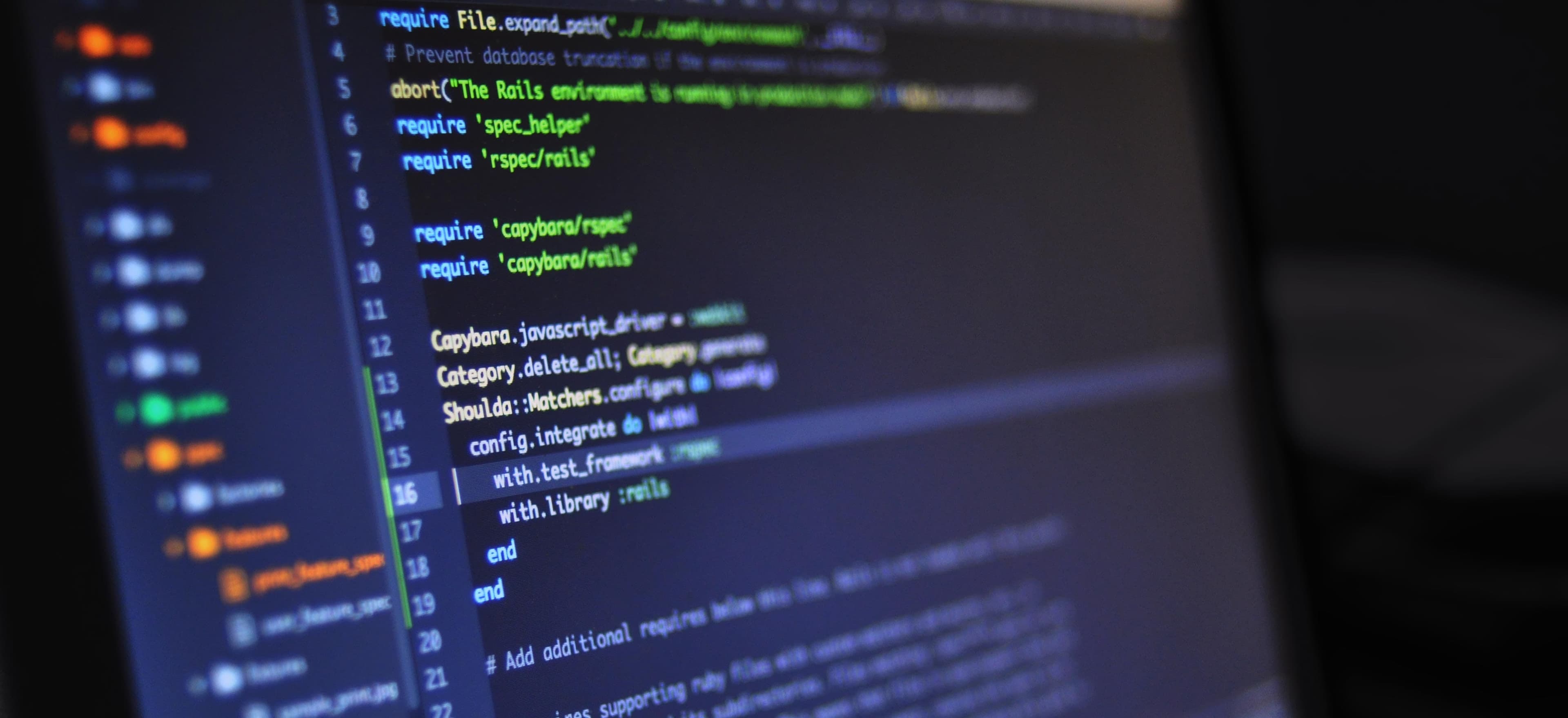
- Published on
Common Pitfalls When Creating Custom Hamcrest Matchers
Hamcrest is a powerful library that allows for expressive and flexible assertions in unit tests. If you've worked with JUnit, you've probably come across Hamcrest matchers without even realizing it. They enable a more readable syntax and provide meaningful error messages when tests fail. However, creating custom matchers can be tricky. This post will explore common pitfalls developers encounter when crafting their own Hamcrest matchers and how to avoid them.
Why Use Hamcrest Matchers?
Using Hamcrest matchers in your tests improves readability. A matcher like containsString("foo")
is easier to understand at a glance compared to writing out a complex assertion. And when you start to create your own matchers, you're extending that readability to your team.
In many cases, you might enhance your matcher to accommodate specific conditions in your domain or application. However, pitfalls lie in wait. Let’s dive into some of the most common ones.
1. Forgetting to Override the matches()
Method Correctly
One of the essential components of any custom matcher is the matches()
method. This method determines whether the object under test meets the criteria defined in the matcher.
public class MyStringMatcher extends org.hamcrest.TypeSafeMatcher<String> {
@Override
public boolean matches(Object item) {
// Ensure item is a string
if (!(item instanceof String)) {
return false;
}
String str = (String) item;
return str.startsWith("Hello");
}
@Override
public void describeTo(Description description) {
description.appendText("a string starting with 'Hello'");
}
}
The Pitfall
If you forget to check the type of the input object and directly cast it, you may encounter a ClassCastException
. Always handle type-checking in your matches()
method.
The Fix
Use instanceof
to ensure the object is of the expected type. This not only prevents runtime errors but also makes your matcher more robust.
2. Neglecting the describeMismatch()
Method
The describeMismatch()
method is critical for debugging. It provides context in error messages when a match fails. Failing to implement this can leave users of your matcher guessing why their tests are failing.
@Override
public void describeMismatch(Object item, Description mismatchDescription) {
if (item instanceof String) {
mismatchDescription.appendText("was a string that started with: ").appendText((String) item);
} else {
mismatchDescription.appendText("was not a string");
}
}
The Pitfall
Omitting the describeMismatch()
method means that your matcher won’t provide specific feedback when it fails. This oversight can lead to frustrating debugging experiences.
The Fix
Implement describeMismatch()
to clearly articulate why the input does not satisfy the matcher. This will save time and increase developer trust in your test suite.
3. Overcomplicating Matchers
Creating complex matchers that do too much can make your tests harder to read and maintain. Aim for simplicity. A matcher should ideally express a single condition.
public class EndsWithMatcher extends TypeSafeMatcher<String> {
private final String suffix;
public EndsWithMatcher(String suffix) {
this.suffix = suffix;
}
@Override
protected boolean matchesSafely(String item) {
return item != null && item.endsWith(suffix);
}
@Override
public void describeTo(Description description) {
description.appendText("a string ending with ").appendValue(suffix);
}
}
The Pitfall
Overly complex matchers can muddy your test logic. If you find yourself adding numerous checks or various conditions into a single matcher, it’s time to reconsider your approach.
The Fix
Break complex conditions into multiple simpler matchers. Not only does this improve readability, but it also allows for easier reuse of individual matchers.
4. Ignoring Thread Safety
If your matcher relies on mutable state or external conditions, it may lead to unreliable tests, especially in multi-threaded environments. Ensure that your matchers are thread-safe.
The Pitfall
Using shared states across multiple threads without synchronization can cause intermittent test failures, making the test results unreliable.
The Fix
Use stateless matchers whenever possible or apply proper synchronization mechanisms when state must be shared.
5. Not Testing Your Matchers
Creating a custom matcher without a corresponding set of unit tests is a significant oversight. Always aim to include tests for your matcher's behavior.
The Pitfall
Skipping tests may lead to unnoticed bugs. This is especially true when you change related components.
The Fix
Write comprehensive unit tests for your custom matchers. For example:
@Test
public void testEndsWithMatcher() {
Matcher<String> matcher = new EndsWithMatcher("World");
assertThat("Hello World", matcher);
assertThat("Hello", not(matcher));
}
Closing Remarks
Creating custom Hamcrest matchers can greatly enhance the expressiveness of your tests. However, it comes with its own set of challenges. By avoiding the pitfalls discussed in this article—type-checking, mismatch descriptions, complexity, thread safety, and the necessity of testing—you can create robust matchers that enhance your team's workflow and testing experience.
For more insights regarding best practices in testing, consider checking out JUnit's official documentation and Hamcrest's comprehensive user guide.
By adopting these strategies, you can ensure that your custom matchers are not only functional but also maintainable and easy to understand. Happy testing!
Checkout our other articles