Common Spring Property Misconfigurations and How to Fix Them
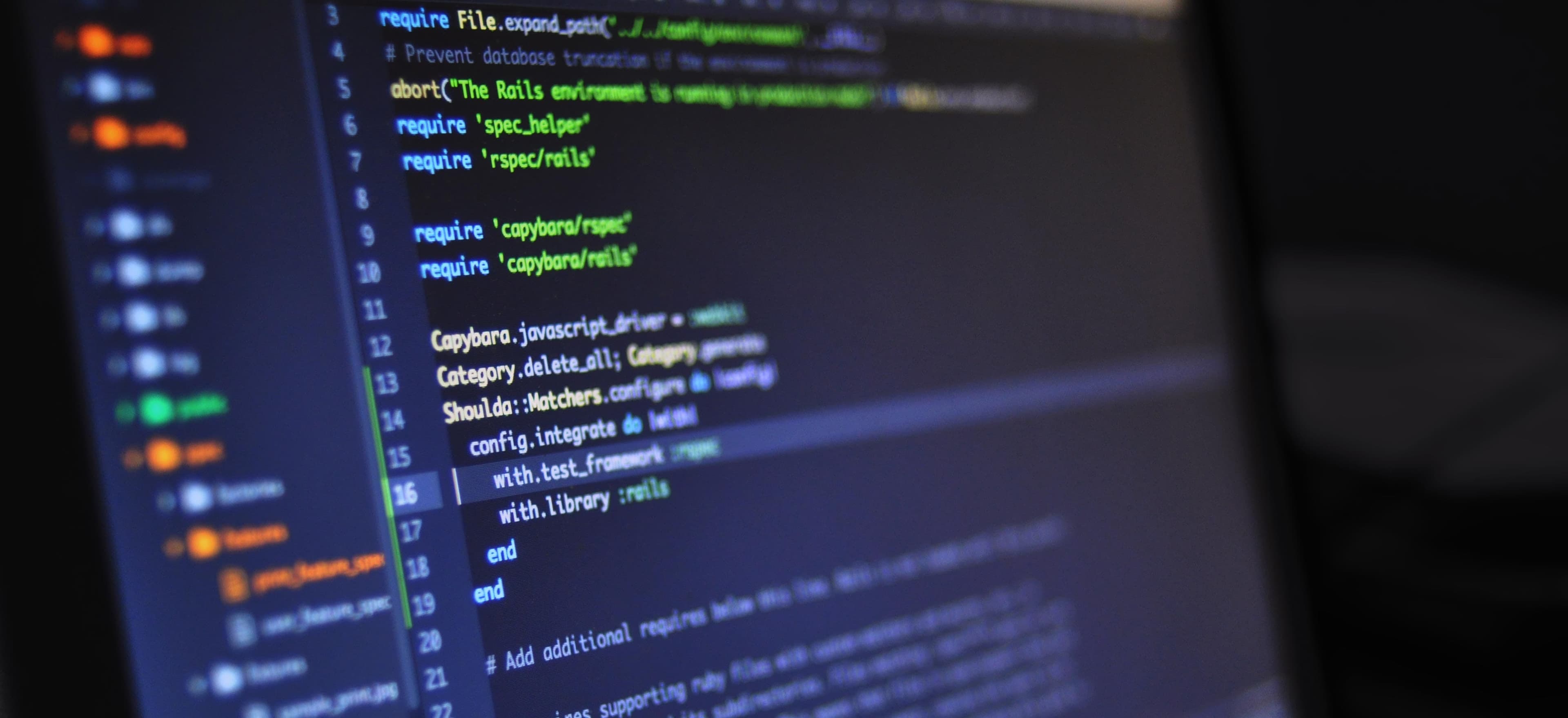
- Published on
Common Spring Property Misconfigurations and How to Fix Them
Spring Framework is one of the most widely used frameworks for Java development. It provides comprehensive infrastructure support for developing Java applications. However, working with Spring also comes with its share of challenges, especially when it comes to configuration. In this blog post, we will explore common property misconfigurations that can occur while using Spring and how to rectify them effectively.
Table of Contents
- Understanding Spring Properties
- Common Misconfigurations
- Best Practices to Avoid Misconfigurations
- Conclusion
1. Understanding Spring Properties
Spring utilizes property files (like application.properties
or application.yml
) to externalize configuration. This allows you to modify various parameters without changing the code. For instance, database URLs, user credentials, and third-party API keys can all be managed via these property files.
Having a clear understanding of how to configure and manage these properties is critical to avoid problems during development.
2. Common Misconfigurations
2.1 Missing Property Placeholders
The Issue
One common pitfall in Spring applications is forgetting to use placeholders for properties. For instance, consider the following configuration in application.properties
:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
If you need to inject this into a bean, you should use the @Value
annotation:
@Value("${spring.datasource.url}")
private String dataSourceUrl;
However, if the placeholder is missing, Spring won't be able to resolve it, leading to IllegalArgumentException
.
The Fix
Ensure you've included the annotation:
@Value("${spring.datasource.url}")
private String dataSourceUrl;
This will ensure that Spring resolves the property correctly.
2.2 Profile-Specific Configurations
The Issue
Spring allows different property files for different environments, or "profiles", (e.g., application-dev.properties
, application-prod.properties
). Sometimes, developers forget to activate the profile, leading to the application loading the default configuration.
The Fix
To activate a specific profile, add the following to your application.properties
:
spring.profiles.active=dev
Alternatively, you can set the profile as an environment variable or as a command-line argument:
-Dspring.profiles.active=dev
2.3 Misconfigured Data Sources
The Issue
Incorrect configuration of data source properties is a frequent source of bugs. For example:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=incorrectPassword
This incorrect configuration results in a failure to connect to the database.
The Fix
Double-check your credentials and URL. Additionally, use a connection pool like HikariCP for better performance:
spring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.minimum-idle=5
2.4 Incorrect Bean Definitions
The Issue
When you define beans in a Spring application, you might accidentally specify properties incorrectly. For instance, if you define a service bean expecting a repository but fail to configure it correctly:
@Service
public class UserService {
@Autowired
private UserRepository userRepo;
}
If UserRepository
is not annotated with @Repository
, it won't be found.
The Fix
Ensure that any class you want to inject is appropriately annotated:
@Repository
public class UserRepository {
// Database operations
}
Additionally, consider the scope of your beans. Use the appropriate scope (singleton, prototype, etc.) to avoid unintended behavior.
2.5 Logging Configuration
The Issue
Logging misconfigurations, such as using the wrong logger level, can seriously complicate debugging. For example, if your log4j.properties
is misconfigured:
log4j.rootLogger=INFO, stdout
You won't see debug-level logs, making it hard to diagnose issues.
The Fix
Adjust your logging level per your requirements:
log4j.rootLogger=DEBUG, stdout
Also, ensure you're logging to the appropriate appender.
3. Best Practices to Avoid Misconfigurations
-
Use Profile-Specific Configuration: Utilize profile-specific properties to manage configurations for different environments. This keeps your code clean and environment-specific.
-
Organize Your Properties: Keep your property files organized; use comments and sectioning to clarify what each part does.
-
Use Default Values: Where appropriate, use defaults to prevent application failure. Use the Spring's
@Value
annotation to set defaults.@Value("${property.name:default_value}") private String propertyName;
-
Validation: Implement validations on configuration properties to avoid runtime exceptions. Spring has support for
@ConfigurationProperties
, which can help validate fields based on their presence or values. -
Test Your Configuration: Always test your application in different configurations to ensure that you've accounted for all possible scenarios.
4. Conclusion
Misconfigurations in Spring properties can lead to frustrating debugging experiences. By being aware of common pitfalls and leveraging best practices, you can streamline your development process and significantly reduce the chances of encountering issues.
For further deeper dives into Spring configuration, consider reading the Spring Framework Documentation.
If you’ve experienced any Spring misconfigurations or have tips for best practices, feel free to share in the comments. Happy coding!
Checkout our other articles