Extracting Column Names from Excel Using Apache POI: A Step-by-Step Guide
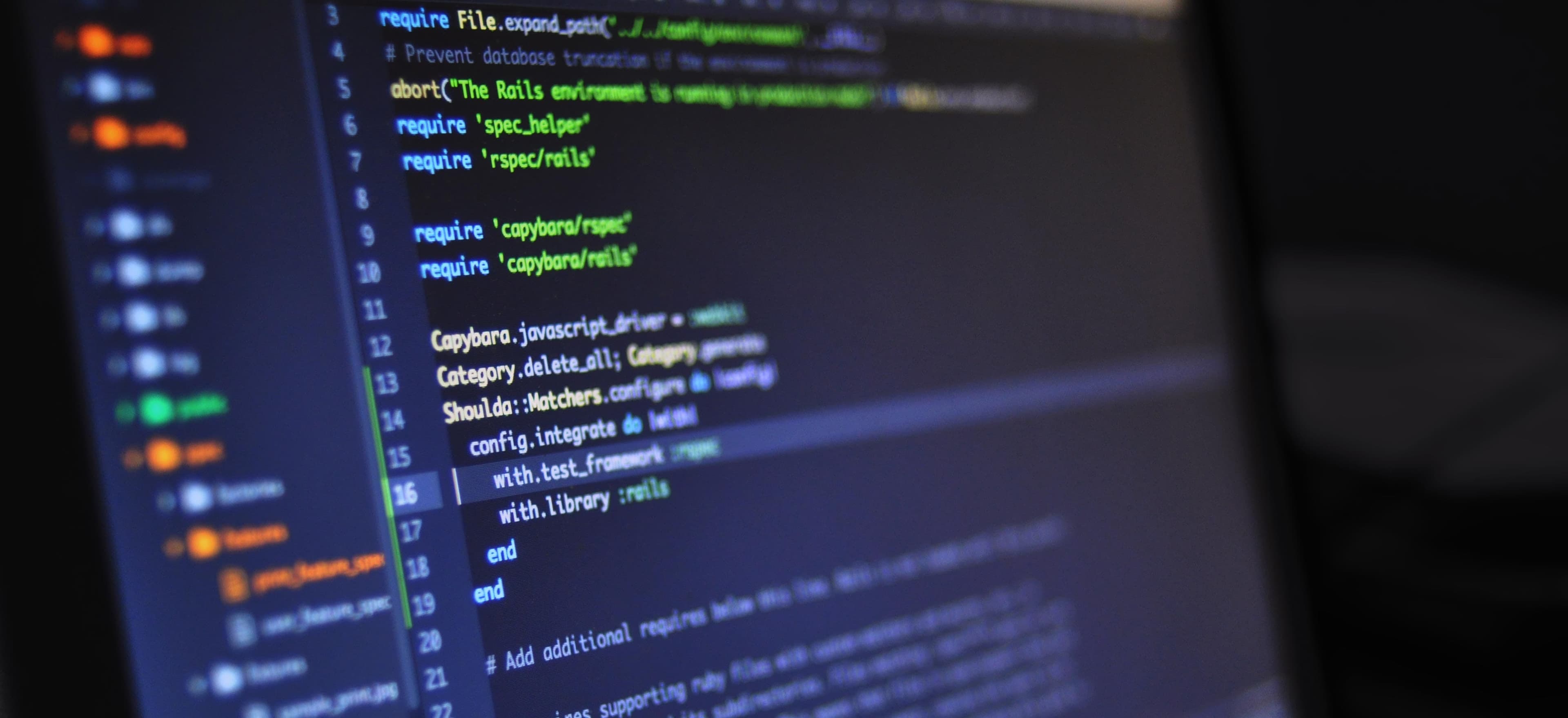
- Published on
Extracting Column Names from Excel Using Apache POI: A Step-by-Step Guide
Excel spreadsheets are prevalent in data management and analysis tasks. Often, developers need to extract data, including column names, for processing, reporting, or integration into applications. Apache POI, a powerful Java library, provides functionality for reading and writing Excel files. In this post, we’ll explore how to extract column names from Excel sheets using Apache POI, and we will break this down into manageable steps.
What is Apache POI?
Apache POI is an open-source Java library that allows you to read and write Microsoft Office formats, including Excel (.xls and .xlsx) files. With POI, your Java applications can easily manipulate Excel data, making it a popular choice for Java developers working with Excel.
Why Extract Column Names?
Extracting column names is essential for several reasons:
- Data Processing: You may need to know the schema of your data.
- Dynamic Queries: Building SQL queries dynamically requires knowledge of the column names.
- Automated Reporting: Identifying which data corresponds to which columns helps create meaningful reports.
Setting Up Apache POI in Your Project
Before we dive into the code, you must have the following dependencies in your project. If you're using Maven, add this snippet to your pom.xml
file:
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.2.3</version>
</dependency>
This dependency includes everything needed to work with .xlsx files. If you're also working with older .xls files, you might consider including the poi
dependency as well.
Step-by-Step Guide to Extracting Column Names
Step 1: Setting Up Your Java Project
Ensure your Java project is set up with the necessary libraries. You might use an IDE like IntelliJ IDEA or Eclipse, which simplifies the process of managing dependencies.
Step 2: Writing the Code
Here’s how to extract column names using Apache POI.
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class ExcelColumnExtractor {
public static List<String> extractColumnNames(String excelFilePath) {
List<String> columnNames = new ArrayList<>(); // Initialize a list to hold column names
try (FileInputStream fileInputStream = new FileInputStream(excelFilePath);
Workbook workbook = new XSSFWorkbook(fileInputStream)) {
Sheet sheet = workbook.getSheetAt(0); // Access the first sheet
Row headerRow = sheet.getRow(0); // Assuming the first row contains column names
// Loop through the cells in the header row
for (Cell cell : headerRow) {
columnNames.add(cell.getStringCellValue()); // Add column name to the list
}
} catch (IOException e) {
e.printStackTrace();
}
return columnNames; // Return the list of extracted column names
}
public static void main(String[] args) {
String path = "example.xlsx"; // Define the path to your Excel file
List<String> columnNames = extractColumnNames(path); // Extract column names
// Print extracted column names
System.out.println("Column Names: " + columnNames);
}
}
Code Breakdown
-
Import Statements: We import essential classes from Apache POI that handle Excel files.
-
Method Definition: We define the
extractColumnNames
method, which:- Takes a file path as an argument.
- Initializes a list for storing column names.
-
File Input Stream: We read the Excel file using a
FileInputStream
, wrapped in a try-with-resources statement to ensure proper resource management. -
Workbook and Sheet: We create an instance of
Workbook
and fetch the first sheet usinggetSheetAt(0)
. -
Column Name Extraction: We iterate over each cell in the first row (assuming it contains the headers) and add the string values to our list.
-
Main Method: This is where execution starts. We specify the path to our Excel file, call the extraction method, and print the results.
Explanation of Key Points
-
Error Handling: Proper exception handling is crucial for managing file IO operations. The
IOException
will help catch issues like file not found or access errors. -
Flexibility: The code currently retrieves names from the first row. If your file structure varies, you may need to modify this behavior to accommodate different rows or sheets as needed.
-
Performance Considerations: For large files, consider using
SXSSFWorkbook
, which is more memory-efficient, particularly if you intend to write data back to Excel.
Additional Features: Customizing Column Selection
You can easily extend this functionality based on your needs. For instance, if you want to skip empty columns or process specific columns by their indices, you would modify the iteration logic.
for (int i = 0; i < headerRow.getPhysicalNumberOfCells(); i++) {
Cell cell = headerRow.getCell(i);
if (cell != null && cell.getCellType() == CellType.STRING) {
columnNames.add(cell.getStringCellValue());
}
}
This addition checks if the cell is not null and that its type is STRING before adding it to the list.
Common Issues and Solutions
Issue: POI Version Compatibility
Make sure you're using compatible versions of Apache POI libraries. Incompatibilities can lead to class not found exceptions.
Issue: File Not Found
Ensure the file path is correct. You can use an absolute path for testing purposes, but relative paths might be more appropriate in production.
The Bottom Line
In this guide, we walked through the process of extracting column names from an Excel file using Apache POI in Java. The simplicity and versatility of Apache POI make it a robust choice for any program needing to work with Excel files.
For deeper insights into both Excel development and Apache POI, you may refer to Apache POI Documentation and explore more advanced functionalities such as cell analysis and manipulation.
Now it's your turn: try implementing this code in your project and see how easily you can manage Excel data! Happy coding!
Checkout our other articles