Resolving Hierarchical Job Status Confusion in Jenkins
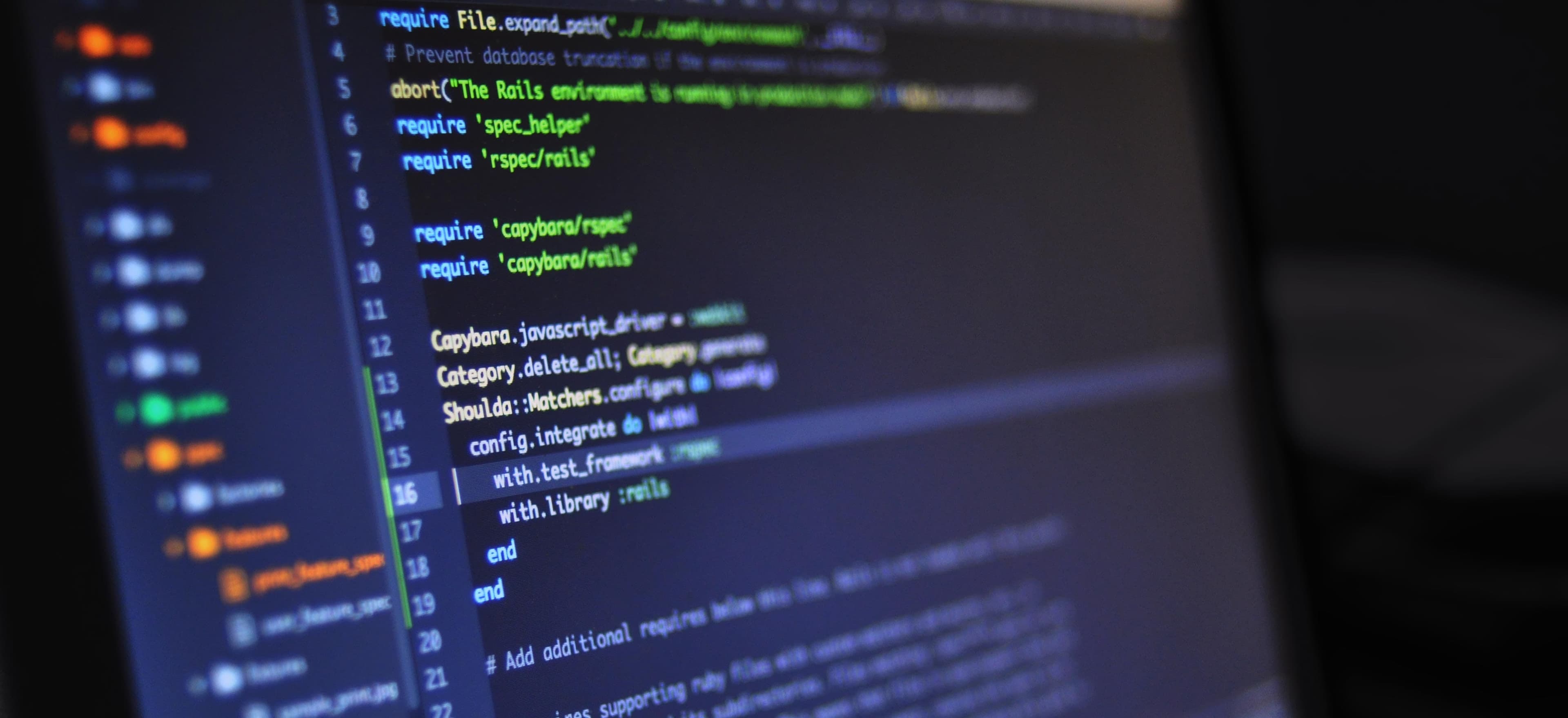
- Published on
Resolving Hierarchical Job Status Confusion in Jenkins
As continuous integration and continuous delivery (CI/CD) become prevalent in software development, Jenkins serves as a powerful tool for automating parts of our project workflow. However, managing multiple jobs can lead to confusion, especially when it comes to understanding the status of hierarchically dependent jobs. In this blog post, we will delve into the intricacies of hierarchical job status in Jenkins, clarify potential pitfalls, and provide you with actionable solutions to keep your CI/CD process efficient and clear.
Understanding Jenkins Jobs and Their Hierarchy
Jenkins jobs can be structured in various ways, often reflecting complex dependencies. For example, a project might have a job for building the code, a job for testing, and another for deployment. If these jobs are interconnected—where one job triggers another—the hierarchy may become complex.
Example of Job Hierarchy
Suppose we have the following jobs:
- Build Job: Compiles the code and creates artifacts.
- Test Job: Runs tests on the built artifacts.
- Deploy Job: Deploys the artifacts to a staging or production environment.
The typical flow looks like this:
- Build Job runs and is successful.
- Test Job is triggered.
- If the Test Job is successful, the Deploy Job is initiated.
While this structure is efficient, ambiguity can arise when jobs fail, or status information is not properly communicated back to the user.
Identifying Job Status Confusion
Job status confusion can manifest in various forms:
- Inconsistent Status Reporting: A downstream job may show "Success" when its upstream job has failed. This can happen if Jenkins is not configured correctly to propagate status.
- False Negatives: Jobs may complete without errors, but related jobs could fail silently due to configuration issues.
- Lack of Visibility into Dependencies: Users might find it challenging to understand which job is dependent on others.
So, how can we resolve this confusion? Let's explore some practical solutions.
Solution #1: Use Job DSL for Clarity
Jenkins Job DSL enables users to define jobs as code. This can improve the understanding of job configurations and dependencies.
job('Build Job') {
steps {
shell('mvn clean install')
}
}
job('Test Job') {
steps {
shell('mvn test')
}
triggers {
upstream('Build Job', 'SUCCESS')
}
}
job('Deploy Job') {
steps {
shell('deploy.sh')
}
triggers {
upstream('Test Job', 'SUCCESS')
}
}
Why Use Job DSL?
- Clarity: Defining jobs in a single coherent language makes it easier to understand relationships.
- Version Control: You can store job configurations in version control systems, making it easier to track changes.
Solution #2: Leverage Pipeline as Code
Jenkins Pipeline provides a way to define job logic in a more detailed and subjectively clearer manner. Pipelines can be created using a Jenkinsfile
which specifies all tasks sequentially or in parallel.
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
def buildResult = sh(script: 'mvn clean install', returnStatus: true)
if (buildResult != 0) {
error "Build failed"
}
}
}
}
stage('Test') {
steps {
script {
def testResult = sh(script: 'mvn test', returnStatus: true)
if (testResult != 0) {
error "Tests failed"
}
}
}
}
stage('Deploy') {
steps {
script {
sh './deploy.sh'
}
}
}
}
}
Why Use Pipelines?
- Error Handling: You can use script blocks to check the status of each step and respond accordingly.
- More Control: Pipelines provide extensive control over execution flow and allow parallel executions, enhancing productivity.
Solution #3: Visualizing Job Dependencies
Using plugins such as the Build Blocker Plugin and the Pipeline Graph View Plugin can help visualize and manage job dependencies.
- Build Blocker Plugin can prevent certain builds from running based on specific triggers.
- Pipeline Graph View Plugin allows a graphical representation of the job flow.
Why Visualize?
- Clarity: Visual representations enhance cognitive understanding of job dependencies, reducing potential confusion.
- Quick Insights: It's easier to determine which part of the pipeline could be causing an error just by identifying it in the graph.
Solution #4: Notifications and Monitoring
Integrating Jenkins with messaging services like Slack, Microsoft Teams, or email notifications can effectively communicate job statuses.
Example Slack Notification
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
try {
sh 'mvn clean install'
} catch (Exception e) {
slackSend(channel: '#jenkins-notifications', message: "Build failed: ${e.message}")
error "Build failed"
}
}
}
}
// Additional stages...
}
}
Why Implement Notifications?
- Instant Awareness: Teams get immediate notifications about job failures.
- Proactive Resolutions: Early alerts allow teams to act quickly, reducing downtime.
A Final Look
By understanding and managing hierarchically dependent jobs in Jenkins, teams can mitigate confusion surrounding job statuses. Utilizing tools such as Job DSL, Pipelines, visualization plugins, and monitoring features will enhance clarity and efficiency.
As CI/CD practices continue to evolve, staying ahead of job status management ensures the robustness and reliability of software delivery.
For further insights on enhancing your Jenkins experience, consider checking out the Jenkins official documentation and the GitHub repository for Jenkins Blue Ocean, which offers a modern user experience that often simplifies the complexities discussed in this article.
Happy coding!
Checkout our other articles