Securing Apache Derby: JVM Policy Pitfalls to Avoid
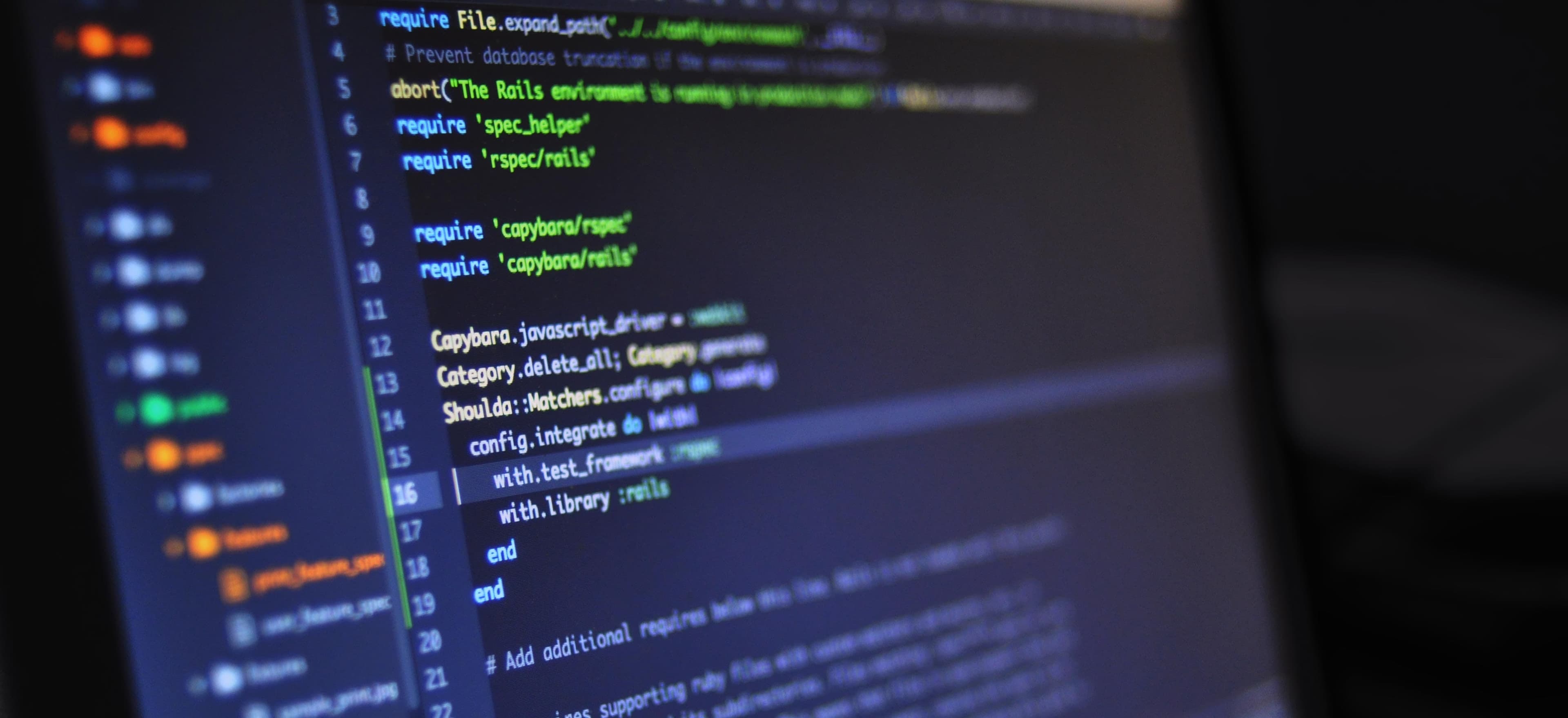
- Published on
Securing Apache Derby: JVM Policy Pitfalls to Avoid
Apache Derby, an open-source relational database implemented in Java, offers a lightweight, easy-to-use platform for developers. However, securing any database is paramount, and Apache Derby is no exception. In this blog post, we will discuss how Java Virtual Machine (JVM) policies can impact the security of your Apache Derby deployment. We will explore common pitfalls, best practices, and provide code snippets to help streamline your security efforts.
Understanding JVM Security Policy
The Java Security Manager is a key component of the Java security architecture, allowing you to define a security policy that can restrict what resources (like files or network connections) a Java application can access. Apache Derby runs within a JVM, which means these security policies are essential in protecting the database from unauthorized access and potential vulnerabilities.
Key Components of JVM Security Policies
- Policy Files: These are text files that specify permissions granted to code sources.
- Code Sources: Locations where the code originated, such as filesystem paths or JAR files.
- Permissions: Rights defined in the policy file to grant or restrict access.
A well-defined JVM policy can prevent unauthorized access but misconfiguration can open dangerous security loopholes.
Common JVM Policy Pitfalls
Understanding common pitfalls when configuring JVM policies can help heighten the security of your Apache Derby implementation.
1. Granting Broad Permissions
One of the most frequent mistakes developers make is granting overly broad permissions. For instance, giving full access to prevent any potential issues can actually create vulnerabilities.
// Too permissive policy granting all rights
grant {
permission java.security.AllPermission;
};
Why This is Dangerous: This configuration allows any code running in the JVM to perform any operation, effectively negating the purpose of security. Instead, aim to define only the permissions needed.
2. Not Specifying Code Source
Failing to specify the code source can lead to unwanted access by untrustworthy code.
// Missing code source specification
grant {
permission java.io.FilePermission "/path/to/derby.log", "read,write";
};
Why This is Dangerous: When the code source is unspecified, any code can gain access to sensitive files, increasing vulnerability. Always specify where the code comes from.
3. Including Sensitive Information in Policy Files
Storing sensitive information, such as database credentials or connection strings, directly in the policy files is a substantial security risk.
// Insecurity due to hardcoded credentials
grant codeBase "file:/path/to/derby.jar" {
permission java.lang.RuntimePermission "accessClassInPackage.com.sensitive", "requiredPrivilege=your-secret-value";
};
Why This is Dangerous: Anyone with access to the policy file can read the credentials, putting your entire deployment at risk. Instead, use environment variables or a secure credential store.
Best Practices for Securing Apache Derby with JVM Policies
To effectively secure Apache Derby using JVM policies, follow these best practices:
1. Least Privilege Principle
Always adhere to the least privilege principle, which dictates that code should only have the permissions necessary to function.
// Grant only required permissions
grant codeBase "file:/path/to/derby.jar" {
permission java.sql.SQLPermission "setNetworkTimeout";
permission java.io.FilePermission "/path/to/derby.log", "read,write";
};
Commentary: This example limits Derby’s permissions to only what is strictly necessary, reducing the attack surface.
2. Regular Audits
Perform regular audits of your JVM policy files. Check for overly permissive grants and ensure compliance with your security policies.
3. Use Secure Configuration Tools
Implement secure configuration management tools (e.g., HashiCorp Vault, AWS Secrets Manager) to manage sensitive information and credentials instead of hardcoding them.
4. Encourage Code Reviews
Encourage diligent code reviews, particularly on security-related configuration. Team members can spot potential pitfalls and suggest improvements.
Example Configuration for Apache Derby
Below is an example of a well-defined policy file for securing an Apache Derby installation. This example is more focused and restricts permissions effectively.
Sample Policy File
// Example policy file for Apache Derby
grant codeBase "file:/path/to/derby.jar" {
// Allow basic JDBC operations
permission java.sql.SQLPermission "setNetworkTimeout";
permission java.sql.SQLPermission "setQueryTimeout";
// Allow reading, writing of log files
permission java.io.FilePermission "/path/to/derby.log", "read,write";
// Restrict to specific file path
permission java.io.FilePermission "/path/to/database/*", "read,write";
};
// Deny anything without explicit permission
grant {
// Explicitly denying broad permissions
permission java.security.AllPermission;
};
Why It Matters
By limiting permissions and explicitly denying broad grants, this configuration minimizes the risk of exploitation.
The Closing Argument
Securing Apache Derby from potential threats starts with a robust JVM policy file. Steering clear of common pitfalls like granting broad permissions, failing to specify the code source, and including sensitive information in the policy are crucial steps towards enhancing database security. By adhering to best practices and maintaining a focus on the least privilege principle, you can create a safer environment for your applications.
For further reading on Java security, consider checking Oracle's official documentation. This thorough understanding will not only help in securing Derby but also any other Java-based application you may be working with.
By integrating clear explanations, practical examples, and actionable recommendations, this blog post aims to equip developers with the knowledge they need to secure their Apache Derby installations effectively. Should you encounter questions or require further assistance, feel free to reach out in the comments below!
Checkout our other articles