Extracting the Last Segment from URIs in Java Made Easy
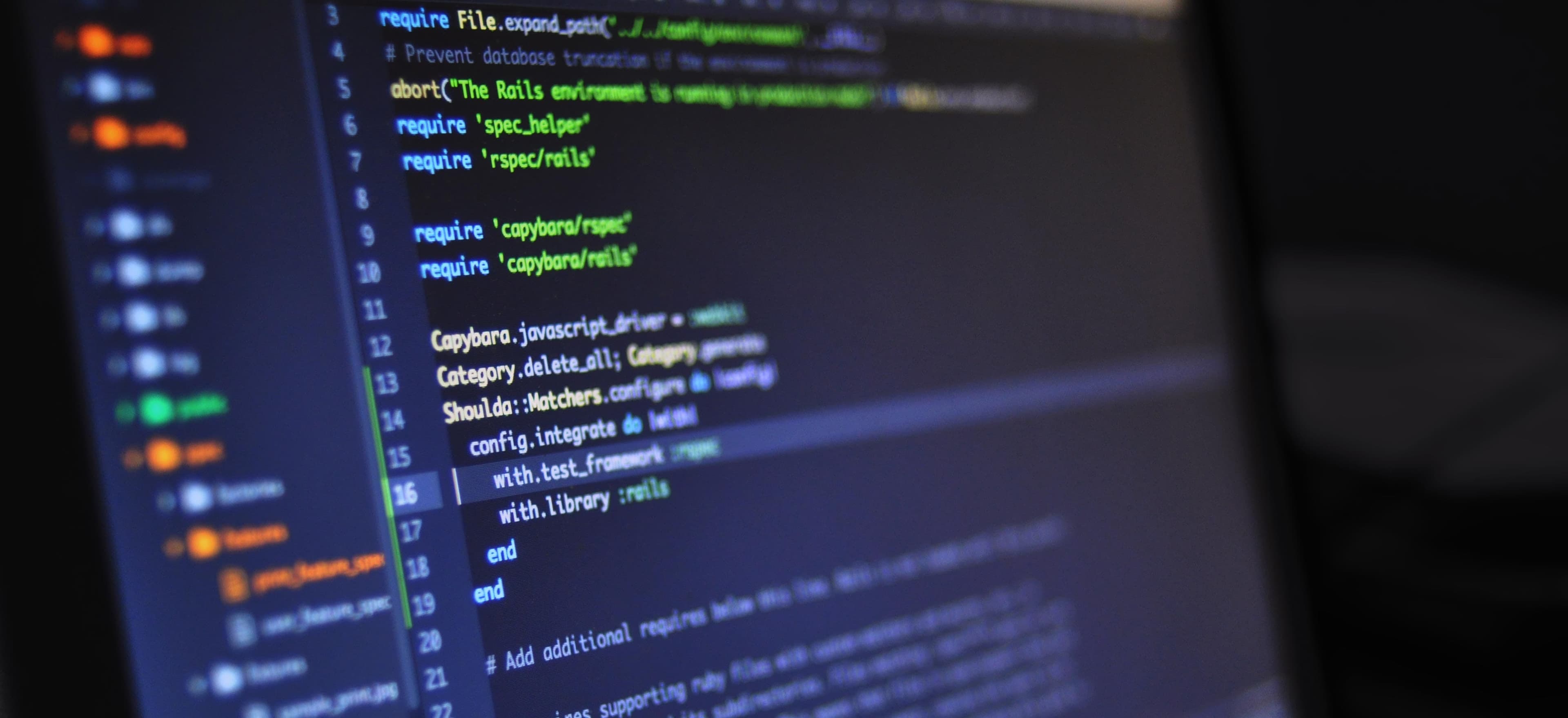
- Published on
Extracting the Last Segment from URIs in Java Made Easy
When working with URIs (Uniform Resource Identifiers) in Java, developers often face the challenge of extracting specific segments from the URL. One common requirement is to retrieve the last segment of a URI. This task may seem trivial, but several nuances can complicate it, such as trailing slashes, variable delimiters, or even special characters.
In this blog post, we'll explore simple methods to extract the last segment from a URI in Java. We will cover practical examples, discuss various approaches, and include best practices for handling URIs effectively.
Understanding URIs
Before diving into the code, let's clarify what a URI is. A URI is a string that identifies a resource. It's crucial for Web development or any system that interacts with resources over HTTP.
A typical URI looks like this:
https://example.com/resource/12345
In this URI:
https
is the scheme.example.com
is the host./resource/12345
is the path.
Here, our task is to extract 12345
, the last segment of the path.
Building a Simple Java Method
We can easily extract the last segment using Java's built-in classes from the java.net
package. Here’s a straightforward method you can utilize:
import java.net.URI;
import java.net.URISyntaxException;
public class URISegmentExtractor {
public static String extractLastSegment(String uriString) throws URISyntaxException {
URI uri = new URI(uriString);
String path = uri.getPath();
// Split the path by "/" and get the last segment
String[] segments = path.split("/");
// Handle empty segments and return the last one
return segments.length > 0 ? segments[segments.length - 1] : "";
}
public static void main(String[] args) {
try {
String uri1 = "https://example.com/resource/12345";
String result1 = extractLastSegment(uri1);
System.out.println("Last segment: " + result1); // Outputs: 12345
String uri2 = "https://example.com/resource/";
String result2 = extractLastSegment(uri2);
System.out.println("Last segment: " + result2); // Outputs: (empty string)
} catch (URISyntaxException e) {
e.printStackTrace();
}
}
}
Explanation of the Code
-
Import Statements: We import the classes required to work with URI and handle exceptions.
-
The
extractLastSegment
Method:- We create a new
URI
object from the string. - Then, we retrieve the path with
uri.getPath()
. - The path is split using the delimiter
/
, creating an array of segments.
- We create a new
-
Segment Handling:
- We check if the
segments
array has any elements. - If not empty, we return the last element; otherwise, we return an empty string.
- We check if the
-
Testing the Method: In the
main
method, we demonstrate how to call this method and print the results.
Handling Trailing Slashes
One consideration is how trailing slashes affect the extraction. The example above correctly handles URIs that end with a slash by returning an empty string. This behavior is essential to ensure consistency and avoid confusion.
Alternative Approach: Using Path
For UNIX-like path semantics, another trendy approach is using the java.nio.file
package:
import java.net.URI;
import java.nio.file.Paths;
import java.nio.file.Path;
public class URISegmentExtractor {
public static String extractLastSegment(String uriString) throws Exception {
URI uri = new URI(uriString);
Path path = Paths.get(uri.getPath());
// Get the last element in the path
return path.getFileName().toString();
}
public static void main(String[] args) {
try {
String uri1 = "https://example.com/resource/12345";
String result1 = extractLastSegment(uri1);
System.out.println("Last segment: " + result1); // Outputs: 12345
String uri2 = "https://example.com/resource/";
String result2 = extractLastSegment(uri2);
System.out.println("Last segment: " + result2); // Outputs: (empty string)
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why Use Path
?
- Simplifies Last Segment Extraction: The
getFileName()
method abstracts much of the manual checking required with splitting. - Better Integration: It integrates better with file-based operations in Java.
Real-World Use Cases
The need to extract segments from URIs is widespread. Here are a few scenarios:
-
Web APIs: When retrieving data from RESTful services, the last segment often identifies a specific resource.
-
URL Routing: Web frameworks may need to interpret the URL structure where the last segment often represents an ID.
-
Logging and Monitoring: By extracting segments, developers can log user actions more efficiently.
Best Practices
-
Validate Input: Before processing a URI, ensure it's valid by catching exceptions. Always handle
URISyntaxException
. -
Use Standard Libraries: Java's built-in libraries provide robust functionalities for URI handling.
-
Consider Edge Cases: Always test your methods against various edge cases such as empty URIs, trailing slashes, and uncommon characters.
-
Performance: For high-performance applications, consider profiling your URI-handling logic, especially if it's in a hot path.
My Closing Thoughts on the Matter
Extracting the last segment from URIs in Java can be executed efficiently using the built-in URI and Path classes. Choosing between these methods depends on the specific context of your application, developer preferences, and performance considerations.
For more information on Java's URI handling, check out the Java Documentation for java.net and NIO file handling.
Now that you are armed with this knowledge, it's time to implement it in your projects and make URI management a breeze! Happy coding!
Checkout our other articles