Optimize Database Queries for Fiber-Based Data Management
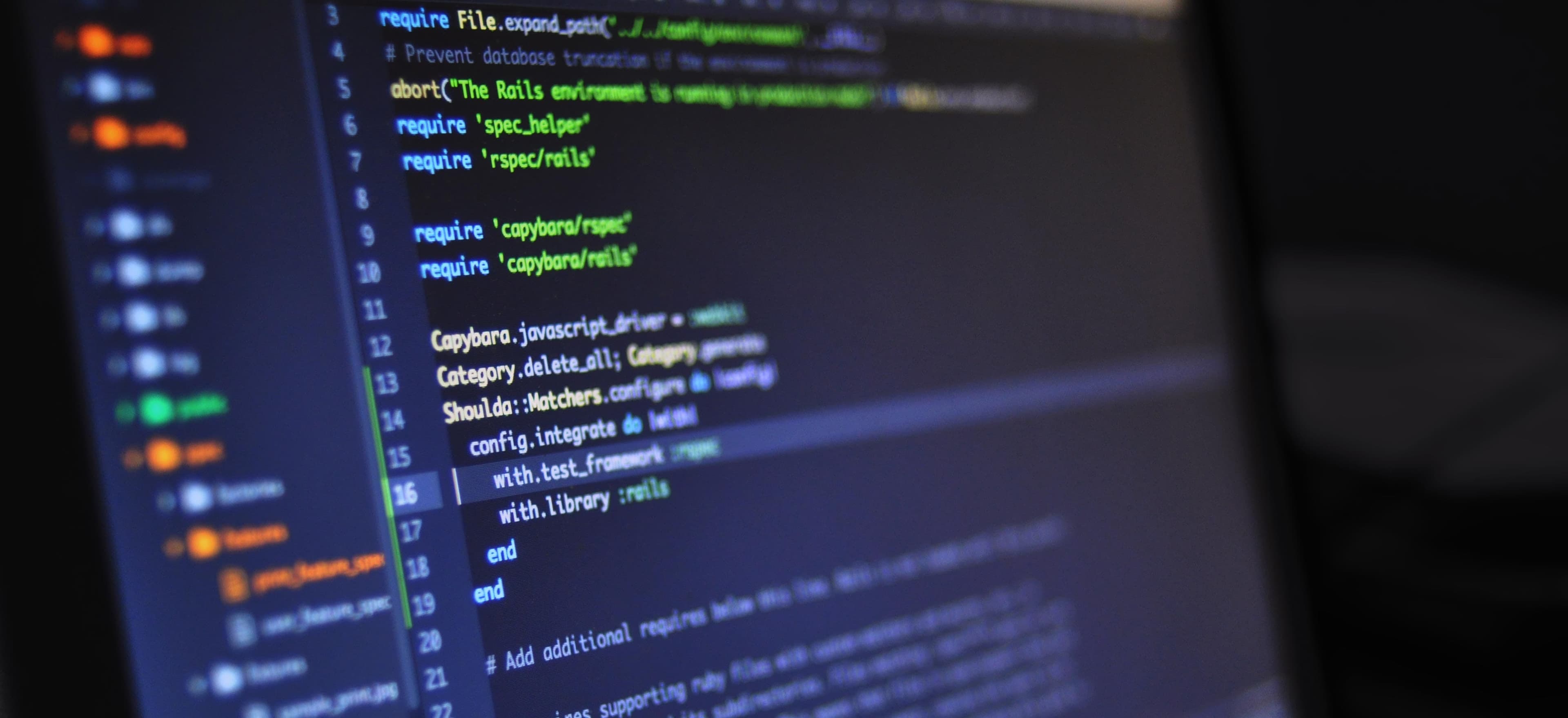
- Published on
Optimize Database Queries for Fiber-Based Data Management
In today's data-driven world, optimizing database queries is crucial for performance, particularly within fiber-based data management systems. Whether you are dealing with a small application or a large-scale enterprise system, efficiently managing queries can significantly impact performance, resource utilization, and overall user experience. In this post, we will explore strategies to optimize database queries specifically for fiber-based data management.
Understanding Fiber-Based Data Management
Fiber-based data management systems utilize a fiber's ability to manage asynchronous operations efficiently. Fibers allow for lightweight thread management, enhancing the performance of I/O-bound operations typical in database interactions. This means that when designing queries, special considerations must be made to leverage the benefits of fiber-based frameworks.
Key Concepts of Fiber-Based Operations
-
Asynchronous Processing: Unlike traditional synchronous processing, fiber-based systems handle multiple requests concurrently, enabling better resource utilization.
-
Lightweight Context Switching: Fibers are less resource-intensive than threads, leading to quicker context switches and minimized overhead.
-
Streamlined I/O Operations: Database operations that often involve waiting for data retrieval can benefit significantly from the non-blocking nature of fibers.
Benefits of Optimizing Database Queries
Optimizing your database queries leads to various advantages, including:
- Improved Application Performance: Faster query execution translates into a more responsive application.
- Reduced Resource Consumption: Efficient queries result in lower CPU and memory usage.
- Enhanced User Experience: Users appreciate applications that provide quick responses, improving retention rates.
Strategies for Optimizing Database Queries
Now that we understand the significance of optimizing queries in the context of fiber-based data management, let’s delve into specific strategies to improve performance.
1. Indexing
Why?
Indexes significantly reduce the amount of data the database needs to scan when executing queries. Properly indexing your database can result in dramatic performance improvements.
Example:
CREATE INDEX idx_user_email ON users(email);
This index on the email
column allows the database to locate user records quickly when searching for a specific email.
Tip: Regularly analyze your queries to determine which columns are frequently accessed. Consider removing unused indexes as they can slow down write operations.
2. Query Optimization Techniques
Why?
Complex queries can often be simplified to improve performance. Look for ways to rewrite queries while retaining their intended output.
Example Improvement:
-- Initial complex query
SELECT a.name, b.amount FROM users a JOIN orders b ON a.id = b.user_id WHERE b.amount > 1000;
This query could be optimized by filtering results early.
-- Optimized query
SELECT a.name, b.amount
FROM (SELECT * FROM orders WHERE amount > 1000) b
JOIN users a ON a.id = b.user_id;
By filtering the orders
table first, we reduce the dataset being joined, resulting in faster execution.
3. Using Parameterized Queries
Why?
Parameterized queries enhance performance and protect against SQL injection attacks. They allow the database to cache execution plans.
Example:
PreparedStatement ps = connection.prepareStatement("SELECT * FROM users WHERE email = ?");
ps.setString(1, userEmail);
ResultSet rs = ps.executeQuery();
This Java code uses a prepared statement. The database can optimize and reuse the execution path whenever the same query structure is called with different parameters.
4. Limit Data Retrieval
Why?
Fetching only the required data rather than all available data minimizes processing and transmission costs.
Example:
SELECT name, email FROM users WHERE status = 'active';
Instead of selecting all columns (SELECT *
), specify only the columns you need. This adjustment can significantly reduce the size of the data returned.
5. Analyze Query Plans
Why?
Understanding how the database executes a query reveals opportunities for optimization, such as identifying bottlenecks or unused indexes.
In PostgreSQL, you can use:
EXPLAIN ANALYZE SELECT * FROM users WHERE email = 'example@example.com';
This command shows the query execution plan, helping you identify slow operations.
6. Batch Processing
Why?
Batching multiple queries into a single transaction improves performance by reducing the overhead of individual transactions.
Example:
Instead of executing multiple insert statements one at a time:
Statement stmt = connection.createStatement();
stmt.executeUpdate("INSERT INTO users (name, email) VALUES ('User1', 'user1@example.com')");
stmt.executeUpdate("INSERT INTO users (name, email) VALUES ('User2', 'user2@example.com')");
You can batch them:
Statement stmt = connection.createStatement();
stmt.addBatch("INSERT INTO users (name, email) VALUES ('User1', 'user1@example.com')");
stmt.addBatch("INSERT INTO users (name, email) VALUES ('User2', 'user2@example.com')");
stmt.executeBatch();
7. Connection Pooling
Why?
Managing a large number of database connections can be resource-intensive. Connection pooling allows your application to reuse connections, reducing overhead.
Example Configuration in Java with HikariCP:
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("user");
config.setPassword("password");
config.setMaximumPoolSize(10);
HikariDataSource ds = new HikariDataSource(config);
By configuring pooling, applications can check out a connection when needed and return it afterward, which can greatly improve performance, particularly in high-load situations.
Closing the Chapter
Optimizing database queries for fiber-based data management is essential to enhancing application performance, reducing resource consumption, and improving user experience. Incorporating strategies such as effective indexing, query optimization, and connection pooling can lead to significant improvements.
To stay current on optimization techniques and best practices, it’s important to periodically review your database queries and performance. For further reading, the following resources are invaluable:
- Database Indexing
- SQL Injection Prevention
- Understanding the EXPLAIN Command
By following these practices, you can ensure a high-performing application that leverages the full power of fiber-based data management systems.
Checkout our other articles