Feature Toggles vs Feature Branches: The Deployment Dilemma
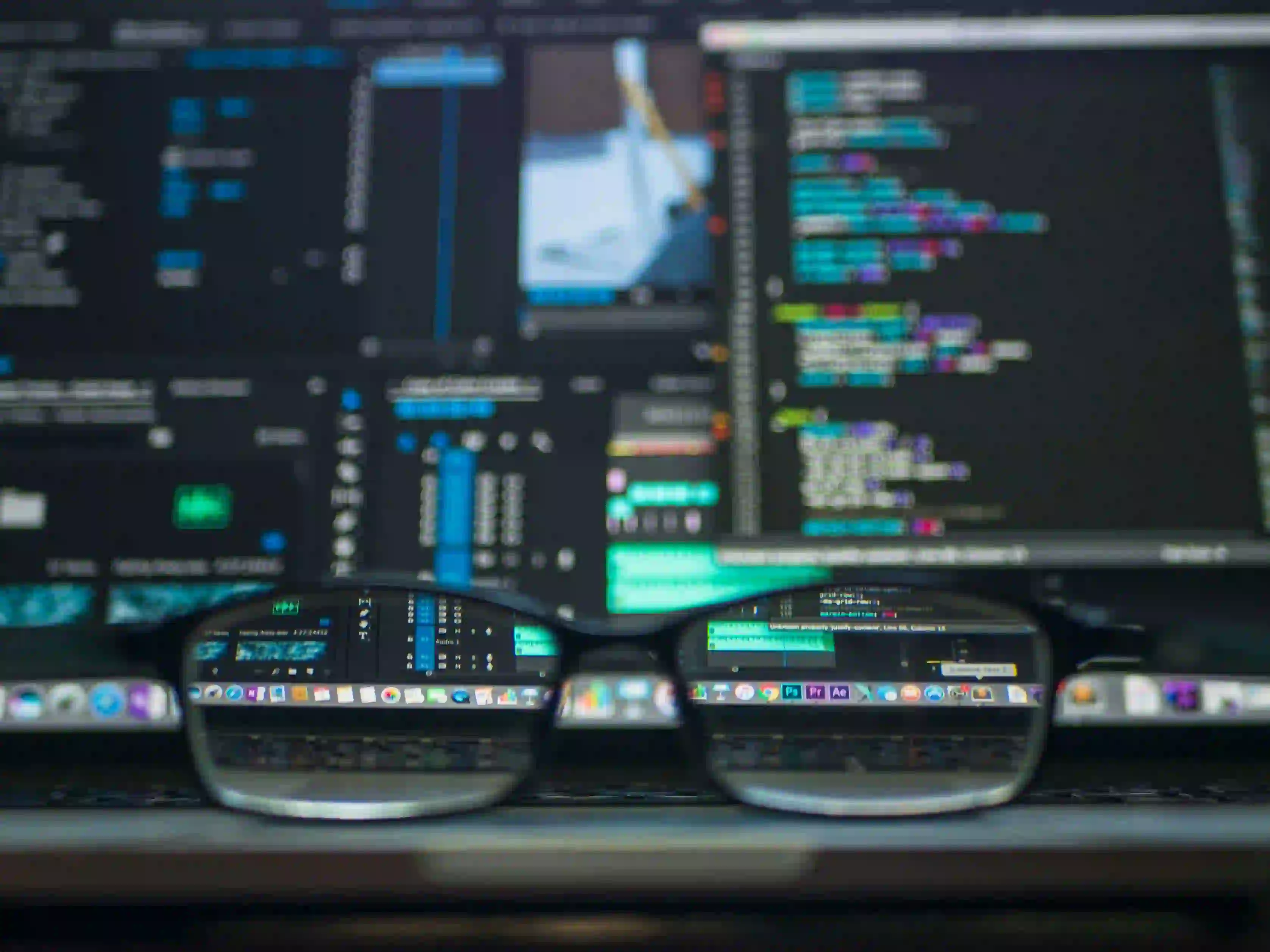
Feature Toggles vs Feature Branches: The Deployment Dilemma
In the world of software development, the strategies for deploying new features are often varied and complex. Two popular methodologies are feature toggles and feature branches. Both contribute to a smoother deployment process, but they come with their own sets of advantages and drawbacks. In this blog post, we'll explore both approaches, compare them, and help you decide which might be the best strategy for your development team.
What are Feature Toggles?
Feature toggles, also known as feature flags, are a way to turn specific features on or off without deploying new code. This enables developers to integrate unfinished features into the main codebase while keeping them hidden from users until they are fully operational.
How Feature Toggles Work
Feature toggles allow developers to launch code in a dormant state. The code is merged into the main branch, but it isn't active. Instead, a configuration option controls whether the feature is visible or not. This can be controlled via configuration files, database entries, or feature management tools.
Example of a Feature Toggle in Java
Consider a simple feature toggle for a user notification method within a Java application.
public class NotificationService {
private boolean featureToggle;
public NotificationService(boolean featureToggle) {
this.featureToggle = featureToggle;
}
public void sendNotification(String message) {
if (featureToggle) {
// Code for sending notification
System.out.println("Notification sent: " + message);
} else {
// Feature is toggled off, ignore the message
System.out.println("Notification feature is off.");
}
}
}
Why Use Feature Toggles?
- Rapid Testing: With feature toggles, developers can deploy code to production without exposing new features immediately. They can test in real scenarios under production load.
- Gradual Rollout: Features can be rolled out to a subset of users first. This helps identify bugs and gather feedback.
- Seamless Rollbacks: If an issue arises, toggling a feature off can quickly eliminate problems without the need for redeployment.
What are Feature Branches?
Feature branches are a method of working on new features in isolation from the main codebase. Developers create a separate branch from the main code repository where they can develop, test, and refine their new features without impacting the production environment.
How Feature Branches Work
When a new feature is under development, a developer creates a new branch. This branch incorporates all the changes needed for that feature. Once the feature is ready, it is merged back into the main branch via a pull request (PR).
Example of Creating a Feature Branch
Here is how you can create a feature branch in Git:
git checkout -b feature/new_notification_service
Why Use Feature Branches?
- Separation of Concerns: Developers can work on separate features without impacting each other. This leads to cleaner code management.
- Code Review: PRs allow team members to review and discuss changes before they are integrated, aiding quality assurance.
- Complete Isolation: The entire feature development happens in isolation which prevents half-finished features from being merged.
Feature Toggles vs Feature Branches: An Overview
Pros and Cons of Feature Toggles
| Pros | Cons | |------|------| | Rapid testing in production environments | May introduce technical debt if toggles are not properly managed | | Enables gradual rollouts and A/B testing | Requires a plan to clean up old toggles | | Allows for easy rollbacks | Complexity in monitoring feature usage |
Pros and Cons of Feature Branches
| Pros | Cons | |------|------| | Cleaner separation of features | Higher risk of merge conflicts | | Easier collaboration and code reviews | Longer deployment times due to integration overhead | | Full isolation prevents half-finished features | Can lead to long-lived branches that drift away from main |
When to Use Which Strategy
Choosing between feature toggles and feature branches often depends on the specific needs of the project and the development team.
Use Feature Toggles When:
- You need to deploy code frequently without exposing new features right away.
- You are performing A/B testing and need to roll out features incrementally.
- You want to make it easy to toggle features on and off based on feedback.
Use Feature Branches When:
- You are working in a more traditional development environment with longer release cycles.
- You require a clean separation of code to facilitate code reviews.
- You want to isolate changes that aren't ready to merge.
Combining Both Approaches
Many organizations find success in combining both strategies. For example, feature branches can be used for significant features while feature toggles can manage accessibility during different release stages or for A/B testing. This strategy effectively capitalizes on the advantages of both methodologies while mitigating their respective weaknesses.
Final Thoughts
Both feature toggles and feature branches offer valuable means for managing software development and deployment. Which one you choose can depend on your team's workflow, the nature of your project, and specific deployment needs. Implementing either practice can greatly enhance your development cycle, improve code quality, and reduce deployment risks.
For more details on agile methodologies and deployment strategies, check out these resources:
By understanding and effectively implementing both feature toggles and feature branches, you can streamline your development process, ensure higher quality in releases, and ultimately deliver a better experience for your users. Choose wisely, and happy coding!