How to Avoid IndexOutOfBoundsException with SubLists in Java
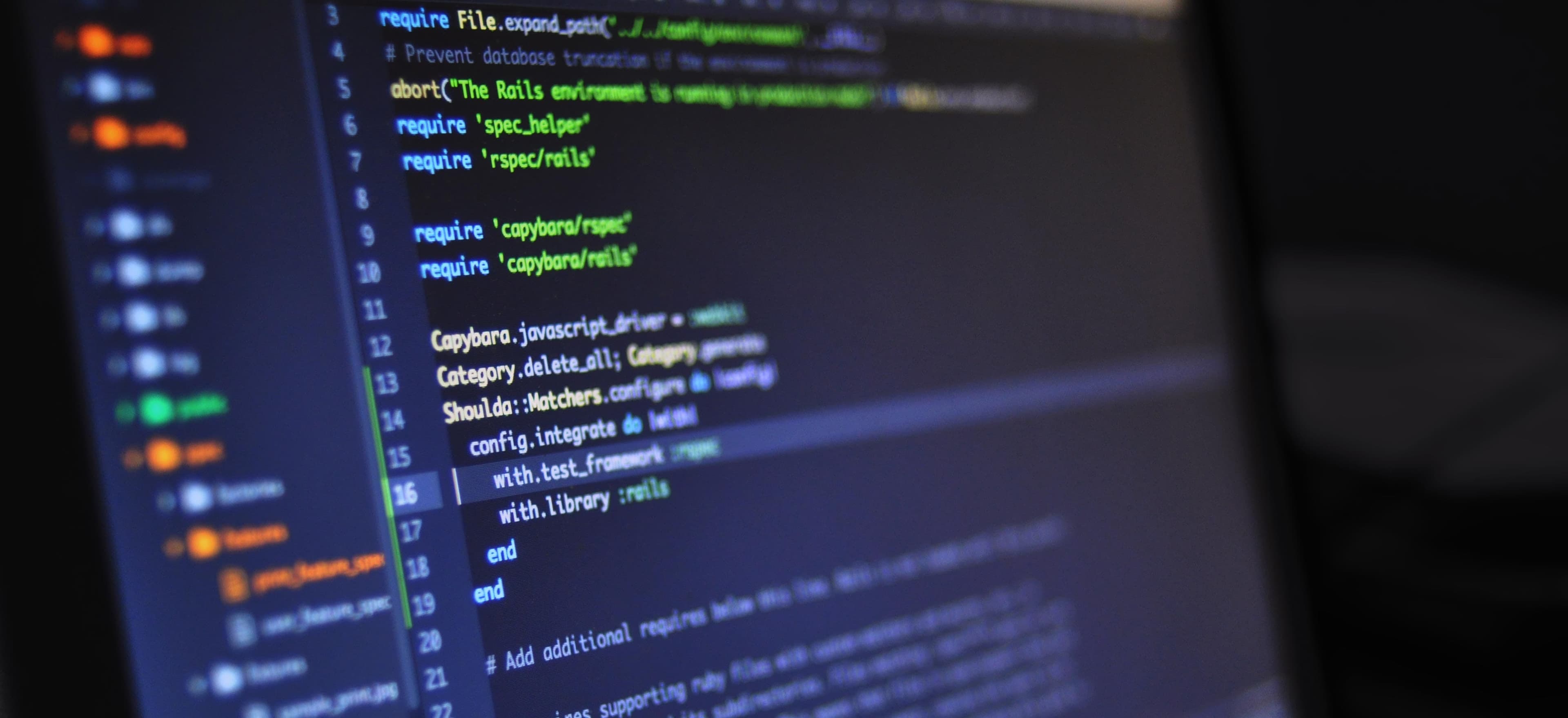
- Published on
How to Avoid IndexOutOfBoundsException with SubLists in Java
The IndexOutOfBoundsException is one of the common runtime exceptions encountered while working with collections in Java. Specifically, this exception often surfaces when dealing with subLists. This post delves into understanding why this exception occurs and how to avoid it while effectively utilizing Java's list manipulation features.
Understanding SubLists
In Java, the List
interface represents an ordered collection (also known as a sequence). A common method provided by the List interface is subList(int fromIndex, int toIndex)
. This method returns a view of the portion of the list between the specified fromIndex
, inclusive, and toIndex
, exclusive.
import java.util.ArrayList;
import java.util.List;
public class SubListDemo {
public static void main(String[] args) {
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
fruits.add("Date");
fruits.add("Elderberry");
List<String> subList = fruits.subList(1, 4);
System.out.println(subList); // Output: [Banana, Cherry, Date]
}
}
Key Points:
- The
fromIndex
is inclusive: the element at this index is included in the subList. - The
toIndex
is exclusive: the element at this index is not included in the subList. - Always remember that the
toIndex
must be greater thanfromIndex
, and both should be within the bounds of the original list.
The Risk of IndexOutOfBoundsException
Understanding the Exception
An IndexOutOfBoundsException occurs when you try to access an index that is outside the valid range of a list (0 to size-1). With subLists, you might run into one of two common pitfalls:
- Invalid index parameters: If
fromIndex
ortoIndex
is less than zero or greater than the size of the list. - Modifying the original list: If you modify the original list after creating a subList, it might lead to an invalid view of the original list.
Example of Invalid Parameters
Consider the following code snippet that attempts to create a subList with incorrect parameters:
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
List<String> subList = vegetables.subList(-1, 2); // Throws IllegalArgumentException
In this case, attempting to access an index less than zero will result in an IllegalArgumentException
rather than an IndexOutOfBoundsException
.
Modifying the Original List
Modifying the original list after creating a subList can also lead to unexpected behavior. The subList is backed by the original list, so any structural modifications to the original list will affect the sublist.
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
List<String> subList = fruits.subList(0, 2);
fruits.add("Date"); // Modifying the original list
System.out.println(subList.get(0)); // Throws ConcurrentModificationException
In the example above, adding "Date" to the original list modifies its structure, leading to a ConcurrentModificationException
when trying to access elements in the subList.
Best Practices to Prevent IndexOutOfBoundsException
1. Always Validate Your Indices
Before creating a subList, ensure your indices are valid. Use conditions to validate fromIndex
and toIndex
.
public static List<String> safeSubList(List<String> list, int fromIndex, int toIndex) {
if (fromIndex < 0 || toIndex > list.size() || fromIndex > toIndex) {
throw new IndexOutOfBoundsException("Invalid indices: fromIndex=" + fromIndex + ", toIndex=" + toIndex);
}
return list.subList(fromIndex, toIndex);
}
This method checks index validity before calling subList
, ensuring your program remains error-free.
2. Avoid Modifying the Base List After Creating the SubList
To prevent unexpected changes, avoid adding or removing elements from the original list after creating a subList. Instead, consider creating a copy of the original list.
List<String> fruitsCopy = new ArrayList<>(fruits); // Create a copy
List<String> subList = fruitsCopy.subList(0, 2);
fruitsCopy.add("Date"); // Safe to modify the copy
3. Use Collections.unmodifiableList
To provide additional safety, consider using Collections.unmodifiableList()
which prevents modification of the original list.
List<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
List<String> unmodifiableFruits = Collections.unmodifiableList(fruits);
List<String> subList = unmodifiableFruits.subList(0, 2);
// Now trying to modify unmodifiableFruits will throw UnsupportedOperationException
4. Deep Copy for Nested Structures
When dealing with lists of lists (nested collections), consider creating a deep copy to prevent nested changes affecting the subLists.
List<List<String>> nestedList = new ArrayList<>();
nestedList.add(new ArrayList<>(List.of("Apple", "Banana")));
nestedList.add(new ArrayList<>(List.of("Cherry", "Date")));
// Work with a deep copy
List<List<String>> deepCopy = new ArrayList<>();
for (List<String> innerList : nestedList) {
deepCopy.add(new ArrayList<>(innerList));
}
List<String> sublist = deepCopy.get(0);
sublist.add("Elderberry");
My Closing Thoughts on the Matter
Avoiding IndexOutOfBoundsException
in Java while working with subLists hinges on proper index validation, avoiding unwanted structural modifications, and utilizing deep copies for nested lists. By implementing these best practices, you can develop robust applications in Java that handle collections efficiently.
For further reading, consult the official Java documentation on List interfaces and Java Collections Framework for an in-depth understanding of Java collections.
Java's collection framework is powerful but can lead to runtime exceptions if not managed carefully. Following the strategies outlined here will significantly lessen your risks and improve your programming fluency in handling lists effectively.