Managing Dependencies in Isolated Development Environments
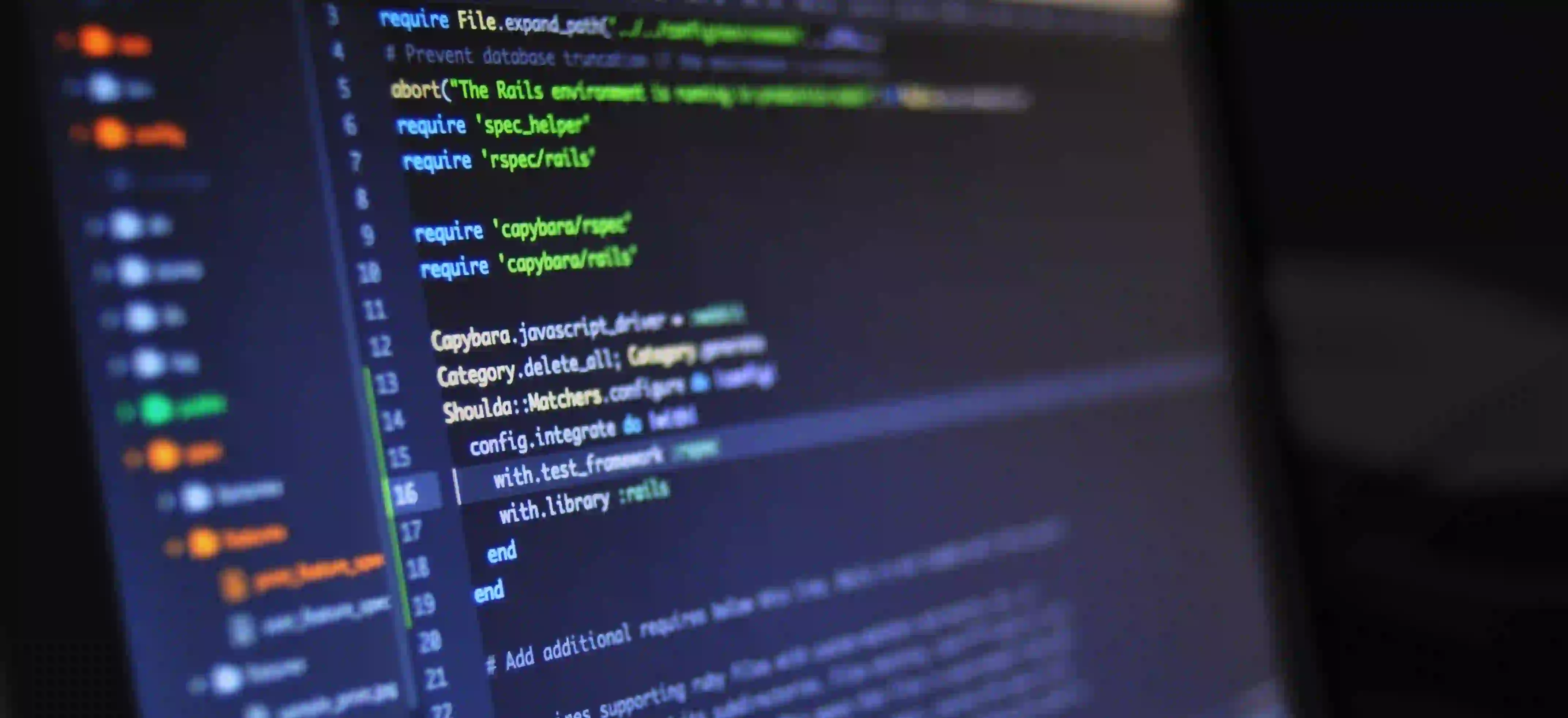
Managing Dependencies in Isolated Development Environments
In today’s fast-paced software development landscape, managing dependencies is more important than ever. The ability to create isolated development environments allows developers to avoid the chaos of dependency conflicts and version mismatches. This blog post delves into the techniques, tools, and practices to effectively manage dependencies, ensuring a smoother development experience.
Understanding Dependency Management
What is a Dependency?
A dependency in software development is any library, tool, or resource that your code relies upon to function correctly. For example, if you're working on a Java project that requires Apache Commons libraries, those dependencies must be managed properly to avoid conflicts.
Why is Dependency Management crucial?
- Isolation: Different projects might need different versions of the same library. Managing dependencies in isolation helps maintain stability.
- Reproducibility: You want to ensure that your code behaves the same way, regardless of the environment it runs in.
- Documentation: Keeping track of dependencies provides insight into what your project relies on and can aid future developers in understanding the codebase.
Tools for Managing Dependencies
There are several tools available that can help manage dependencies effectively:
1. Maven
Maven is one of the most popular build automation tools used primarily for Java projects. It encourages a convention-over-configuration approach and helps manage project dependencies through a single pom.xml
file.
Example: Basic Maven POM Configuration
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
</project>
Why Choose Maven?
Maven's declarative nature allows developers to focus on what they need, rather than how to get it. The dependencies specified in pom.xml
ensure that the required libraries are downloaded and included in the classpath seamlessly.
2. Gradle
Gradle is another powerful build tool that is gaining popularity. It combines the best features of Ant and Maven while using Groovy or Kotlin DSL for configuration.
Example: Basic Gradle Build Configuration
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.apache.commons:commons-lang3:3.12.0'
}
Why Choose Gradle?
Gradle allows for more advanced configuration scenarios and offers better performance through its incremental builds. It is particularly well-suited for multi-project builds, which are common in large software systems.
3. Docker
While Maven and Gradle focus on managing dependencies at the project level, Docker provides a way to manage runtime environments, including all dependencies, through containerization.
Example: Dockerfile Configuration
FROM openjdk:11
COPY target/my-app-1.0-SNAPSHOT.jar my-app.jar
CMD ["java", "-jar", "my-app.jar"]
Why Choose Docker?
Using Docker allows you to encapsulate your application and its dependencies into a single container image. This means improved portability and consistent behavior across different environments, from development to production.
Best Practices for Managing Dependencies
-
Use Dependency Management Tools Wisely: Understand the tools' features and leverage them to simplify your dependency management process.
-
Keep Dependencies Up-to-Date: Regularly check for updates to your dependencies and apply them judiciously to avoid security vulnerabilities.
-
Isolate Development Environments: Use containers or virtual environments to ensure that each project works with its specific dependencies without interference.
-
Document Dependencies: Maintain clear documentation about what libraries and tools are in use and their roles in the project.
-
Leverage Version Control: Use version control systems like Git along with branch strategies to manage changes in dependencies effectively.
-
Employ Dependency Scanners: Leverage tools like OWASP Dependency-Check or Snyk to identify security vulnerabilities in the libraries that you depend on.
Final Considerations
Managing dependencies effectively in isolated development environments is crucial for modern software development. By using tools like Maven, Gradle, and Docker, developers can ensure that their projects remain stable and portable. Following best practices helps in maintaining a healthy, secure codebase.
For further learning, consider checking out Apache Maven Official Documentation and Gradle User Manual.
Embracing these practices equips developers to handle the complexities of dependency management and leads to more efficient and productive software development workflows. Happy coding!