Common Morphia Pitfalls When Using MongoDB
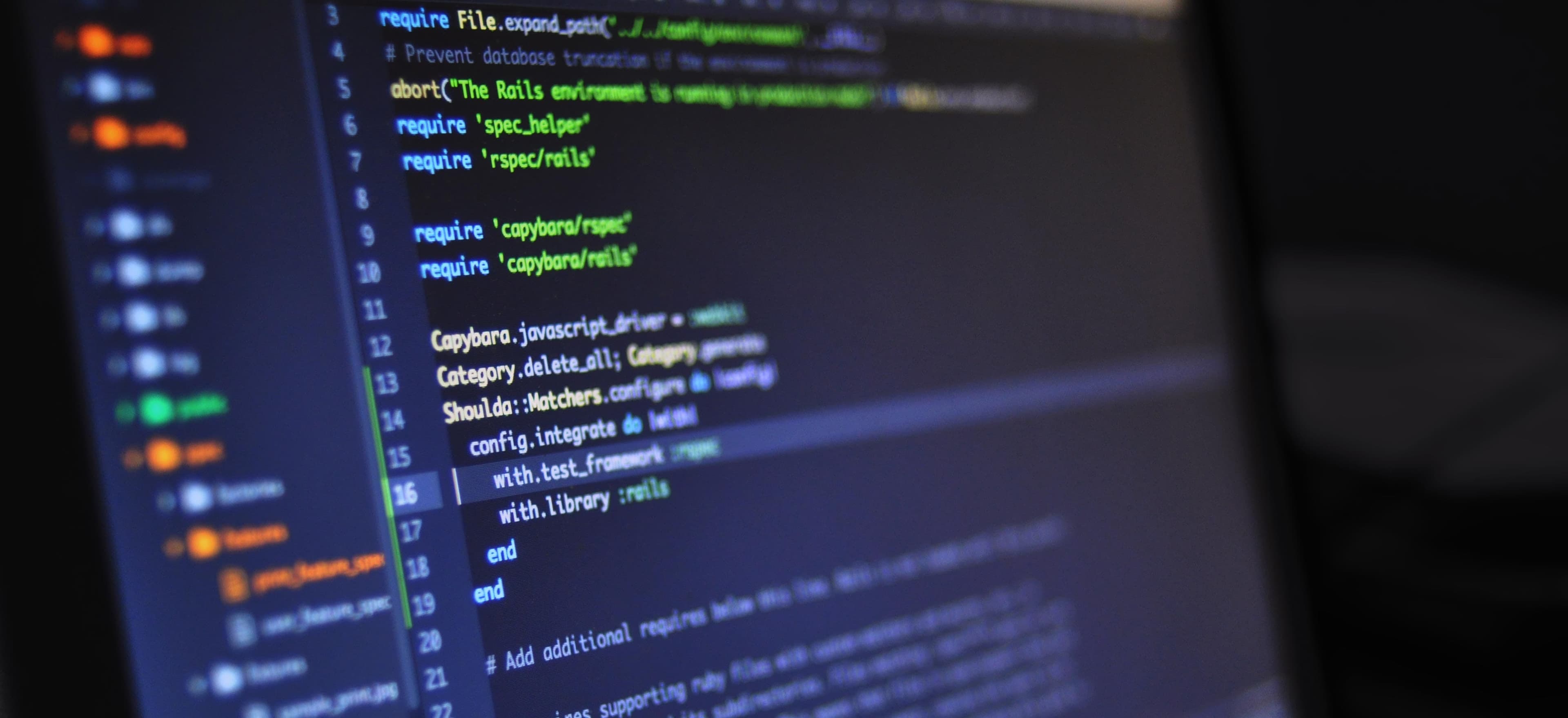
- Published on
Common Morphia Pitfalls When Using MongoDB
Morphia is a powerful yet simple Object-Document Mapper (ODM) for MongoDB that integrates seamlessly with Java. While it simplifies data interactions, developers can encounter pitfalls that can complicate, or even sabotage, their efforts. In this blog, we will explore these common pitfalls, helping you harness the full potential of Morphia in your Java applications.
Understanding Morphia
Before diving into the pitfalls, it’s essential to have a foundational understanding of Morphia. Morphia maps Java objects to MongoDB documents. This means you can work with Java classes while utilizing the underlying benefits of MongoDB, such as flexible schemas and rich data types (learn more about MongoDB with Morphia).
Pitfall 1: Not Using @Entity Correctly
Morphia relies heavily on annotations to map Java classes to MongoDB documents. The @Entity
annotation is fundamental. Failing to apply it correctly can result in your entities not being stored correctly.
Example
import org.mongodb.morphia.annotations.Entity;
@Entity("users")
public class User {
private String username;
private String email;
// Getters and setters
}
Why It Matters
Without the @Entity
annotation, Morphia cannot identify the class as a document to be stored in the database. Always ensure that you apply it to classes intended for database representation.
Pitfall 2: Ignoring Access Modifiers
Morphia requires a default (no-argument) constructor for serialization. If you do not provide one, you may encounter InstantiationException
.
Example
import org.mongodb.morphia.annotations.Entity;
@Entity("users")
public class User {
private String username;
private String email;
// Default constructor
public User() {}
// Getters and setters
}
Why It Matters
The default constructor allows Morphia to create an instance of the class during data retrieval from MongoDB. Ignoring this will lead to runtime exceptions that can derail your data access logic.
Pitfall 3: Misunderstanding Basic Types
When declaring fields in Morphia entities, it’s crucial to be aware of how Morphia handles basic types versus their wrapper classes. For example, using int
instead of Integer
can lead to unexpected behavior.
Example
public class User {
private String username;
private Integer age; // Prefer Integer over int
}
Why It Matters
MongoDB’s BSON does not support numeric types like int
directly. Instead, Integer
maps correctly to BSON types in Morphia. Misunderstanding types may lead to data inconsistency or exceptions.
Pitfall 4: Not Using the Correct Type for Collections
Morphia supports various collection types, though it’s best to use List
or Set
over traditional arrays or other collection types.
Example
import org.mongodb.morphia.annotations.Entity;
import org.mongodb.morphia.annotations.Transient;
import java.util.List;
@Entity("users")
public class User {
private String username;
private List<String> friends; // Using List for collection
// Getters and setters
}
Why It Matters
Using collections like arrays can cause issues during data retrieval and manipulations. Morphia works best with its supported types, ensuring seamless data interactions.
Pitfall 5: Forgetting to Use @Id Annotation
The presence of an identifier for each MongoDB document is crucial. If you do not specify an ID field with the @Id
annotation, Morphia will not correctly manage your document’s identity.
Example
import org.mongodb.morphia.annotations.Entity;
import org.mongodb.morphia.annotations.Id;
@Entity("users")
public class User {
@Id
private String id; // Use @Id here
private String username;
// Getters and setters
}
Why It Matters
The @Id
annotation allows Morphia to manage the unique identification of documents. Forgetting it means risking duplicate entries or improper retrievals.
Pitfall 6: Not Leveraging Transactions
While MongoDB supports atomic operations at a document level, Morphia enhances this functionality through its transaction support. Neglecting to use transactions appropriately can lead to data inconsistencies.
Example
import org.mongodb.morphia.Datastore;
import org.mongodb.morphia.MongoClient;
public class TransactionExample {
public void performTransaction() {
Datastore datastore = new MongoClient().getDatastore();
datastore.ensureIndexes();
try {
datastore.getMongo().startSession();
datastore.getMongo().startTransaction();
// Perform multiple writes or updates
datastore.save(new User("JohnDoe"));
datastore.save(new User("JaneDoe"));
datastore.getMongo().commitTransaction(); // Commit the transaction
} catch (Exception e) {
datastore.getMongo().abortTransaction(); // Rollback on errors
}
}
}
Why It Matters
Transactions enable multiple operations to be executed in an all-or-nothing manner. Not using them could leave your database in an inconsistent state when updates fail.
Pitfall 7: Not Configuring Indexes Properly
MongoDB’s indexing is crucial for performance. Simply relying on Morphia's automatic indexing without understanding your query patterns can lead to suboptimal performance.
Example
import org.mongodb.morphia.annotations.Index;
@Entity("users")
@Index("username")
public class User {
@Id
private String id;
private String username;
// Getters and setters
}
Why It Matters
Proper indexing can drastically reduce query time. Ensure that you examine your access patterns and configure indexes accordingly to enhance performance.
Practical Tips to Avoid These Pitfalls
-
Thoroughly Read Morphia Documentation: Familiarize yourself with the official Morphia documentation for comprehensive details.
-
Use IDE Support: Tools such as IntelliJ IDEA offer plugins for MongoDB and Morphia, enhancing your development experience and reducing mistakes.
-
Unit Test Your Entities: Implement unit tests to check the serialization and deserialization of your entities, ensuring that everything functions as expected.
-
Profile Your Queries: Leverage MongoDB's built-in profilers to understand your application's behavior and optimize where necessary.
-
Stay Updated: Keep track of updates in Morphia and MongoDB to harness new features and enhancements.
In Conclusion, Here is What Matters
Using Morphia with MongoDB can significantly ease data manipulation in your Java applications. However, avoiding these common pitfalls while understanding the "why" behind coding practices is paramount. Armed with this knowledge, you can build robust and efficient data access layers, enhancing your application's performance and reliability.
Have you experienced any pitfalls when using Morphia? Share your experiences in the comments below!
Checkout our other articles