Maximizing Performance in High Concurrency HTTP Servers
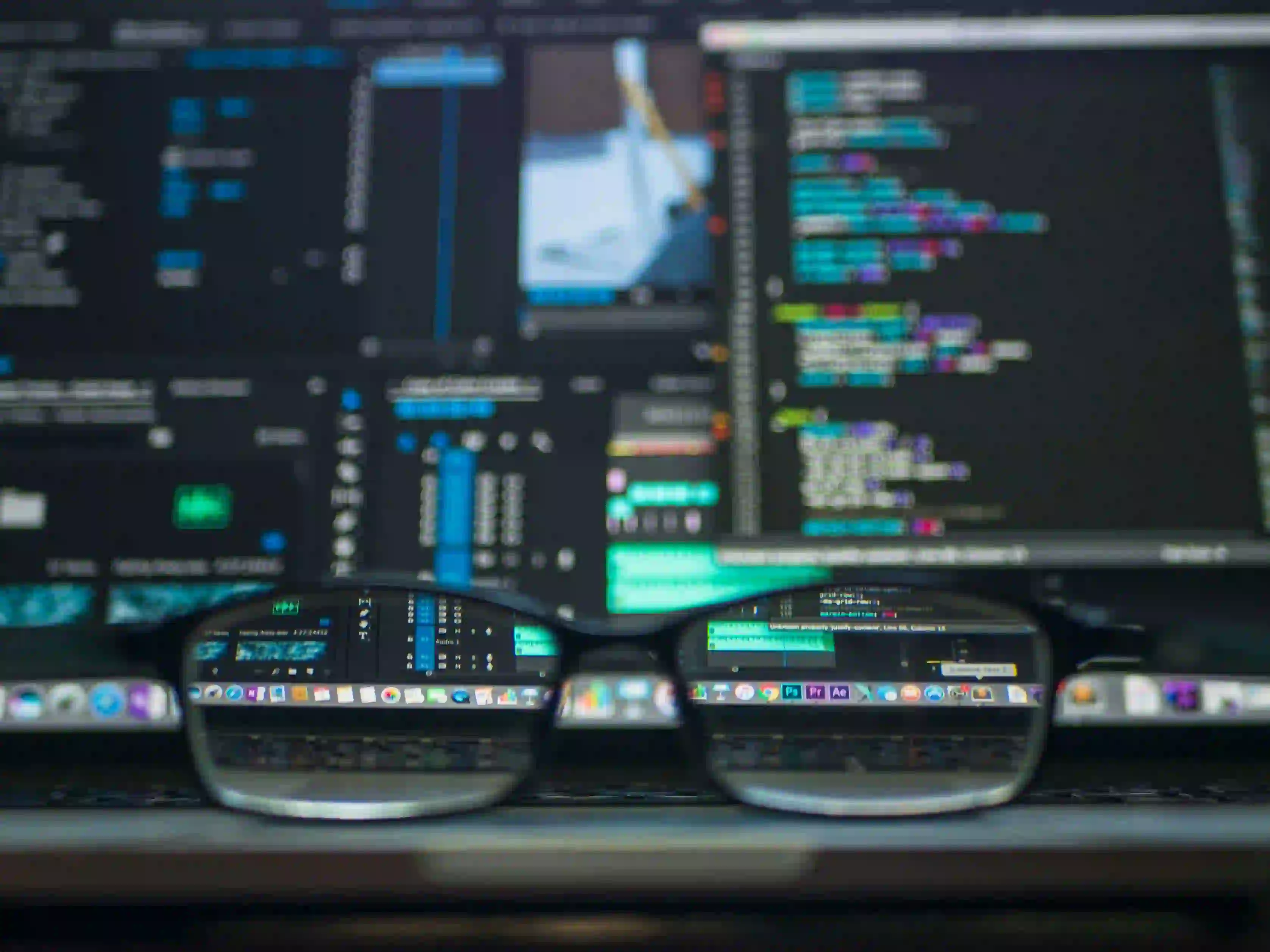
Maximizing Performance in High Concurrency HTTP Servers
As the digital landscape evolves, the demand for responsive and high-performing web applications increases. One of the critical challenges developers face is building HTTP servers that can handle high concurrency, serving thousands or even millions of requests simultaneously. In this blog post, we'll discuss strategies for maximizing performance in high concurrency HTTP servers, primarily using Java.
Understanding Concurrency in HTTP Servers
Concurrency refers to the ability of a server to handle multiple requests at the same time. With a web server, this often translates to receiving HTTP requests from numerous clients and responding to them seamlessly. Understanding how to maximize concurrency is crucial for ensuring a smooth user experience.
Key Characteristics of High Concurrency Servers
- Scalability: The server should efficiently manage an increasing number of requests without a decline in performance.
- Efficiency: Use of system resources (CPU and memory) should be optimized for low overhead and high throughput.
- Responsiveness: Users expect quick response times; even under load, requests should be processed promptly.
Building a High Concurrency HTTP Server with Java
When building a high-performance HTTP server in Java, we can leverage asynchronous programming, lightweight frameworks, and efficient resource management. Below, we will examine a few architectural patterns and techniques.
1. Asynchronous Programming with Java NIO
Java NIO (New Input/Output) enables non-blocking I/O operations, allowing a single thread to manage multiple connections. This can greatly improve the throughput of concurrent requests.
Example Code Snippet: Setting Up a Simple NIO Server
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.*;
public class NioHttpServer {
private Selector selector;
private static final int PORT = 8080;
public NioHttpServer() throws IOException {
selector = Selector.open();
ServerSocketChannel serverSocket = ServerSocketChannel.open();
serverSocket.bind(new InetSocketAddress(PORT));
serverSocket.configureBlocking(false);
serverSocket.register(selector, SelectionKey.OP_ACCEPT);
System.out.println("Server started on port: " + PORT);
}
public void start() throws IOException {
while (true) {
selector.select();
for (SelectionKey key : selector.selectedKeys()) {
if (key.isAcceptable()) {
handleAccept(key);
} else if (key.isReadable()) {
handleRead(key);
}
selector.selectedKeys().remove(key);
}
}
}
private void handleAccept(SelectionKey key) throws IOException {
ServerSocketChannel serverSocket = (ServerSocketChannel) key.channel();
SocketChannel clientChannel = serverSocket.accept();
clientChannel.configureBlocking(false);
clientChannel.register(selector, SelectionKey.OP_READ);
}
private void handleRead(SelectionKey key) throws IOException {
SocketChannel clientChannel = (SocketChannel) key.channel();
ByteBuffer buffer = ByteBuffer.allocate(256);
int bytesRead = clientChannel.read(buffer);
if (bytesRead == -1) {
clientChannel.close();
} else {
// Process request and send response
// Here you would parse HTTP request and generate response
buffer.flip();
clientChannel.write(buffer);
}
}
public static void main(String[] args) throws IOException {
new NioHttpServer().start();
}
}
Commentary
This example demonstrates how to set up a basic NIO server that can accept multiple clients simultaneously. By avoiding blocking I/O, this server can efficiently manage client connections, resulting in high concurrency.
2. Using a Thread Pool for Request Handling
While NIO can manage a large number of foreign requests, it may not always be suitable for processing heavy computational tasks. Here’s where a thread pool becomes invaluable. By delegating the processing of requests to worker threads, we can ensure responsiveness.
Example Code Snippet: Integrating Thread Pool
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
// Inside your NioHttpServer class:
private ExecutorService executorService;
public NioHttpServer() throws IOException {
// Existing setup code
executorService = Executors.newFixedThreadPool(10); // Customize size based on workload
}
private void handleRead(SelectionKey key) throws IOException {
SocketChannel clientChannel = (SocketChannel) key.channel();
ByteBuffer buffer = ByteBuffer.allocate(256);
int bytesRead = clientChannel.read(buffer);
if (bytesRead != -1) {
buffer.flip();
// Submit the request handling to the executor
executorService.submit(() -> processRequest(buffer, clientChannel));
} else {
clientChannel.close();
}
}
private void processRequest(ByteBuffer buffer, SocketChannel clientChannel) {
// Process your HTTP request and prepare the response
// Example: Parsing HTTP headers, generating responses, etc.
}
Commentary
Using an ExecutorService allows us to decouple I/O operations from request processing. While one thread manages I/O, others can be busy executing business logic. This approach balances load effectively, especially in high concurrency scenarios.
3. Employing Caching for Improved Performance
Caching can significantly reduce the load on your server and improve response times for frequently requested resources. By storing responses or computational results, you can avoid repeat processing, giving a quick response to users.
Example Code Snippet: Simple Caching Mechanism
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class SimpleCache {
private final Map<String, String> cache = new ConcurrentHashMap<>();
public String get(String key) {
return cache.get(key);
}
public void put(String key, String value) {
cache.put(key, value);
}
}
// Usage in your processRequest method
String cachedResponse = cache.get(requestKey);
if (cachedResponse != null) {
sendResponse(clientChannel, cachedResponse);
} else {
// Process and cache the response
String response = generateResponse(request);
cache.put(requestKey, response);
sendResponse(clientChannel, response);
}
Commentary
A simple cache implementation utilizing ConcurrentHashMap allows for thread-safe operations. When your server responds to requests, this caching mechanism ensures that frequently accessed content is served faster.
4. Load Balancing for Distribution of Requests
In a production environment, scaling horizontally by deploying multiple instances of your server can be essential to handle high traffic. A load balancer can effectively distribute requests between these instances.
The Bottom Line
Maximizing performance in high concurrency HTTP servers requires careful consideration of the architecture and individual components involved. Java's capabilities, including NIO for non-blocking I/O, thread pools for efficient processing, caching to minimize redundancy, and load balancing for scalability, make it a robust choice for developing high-performance applications.
By implementing these strategies, developers can ensure their web services are efficient, scalable, and resilient against high traffic. If you're looking to understand more about concurrent programming or seek further insights into server optimization techniques, check out the Java Concurrency tutorial or explore the Java NIO documentation for deeper insights.
With the right design patterns, you can build a server capable of handling the demands of today's web applications. So go ahead and implement these practices in your next project to build a performant high-concurrency HTTP server in Java!