Overcoming Common Challenges with Java Management Extensions
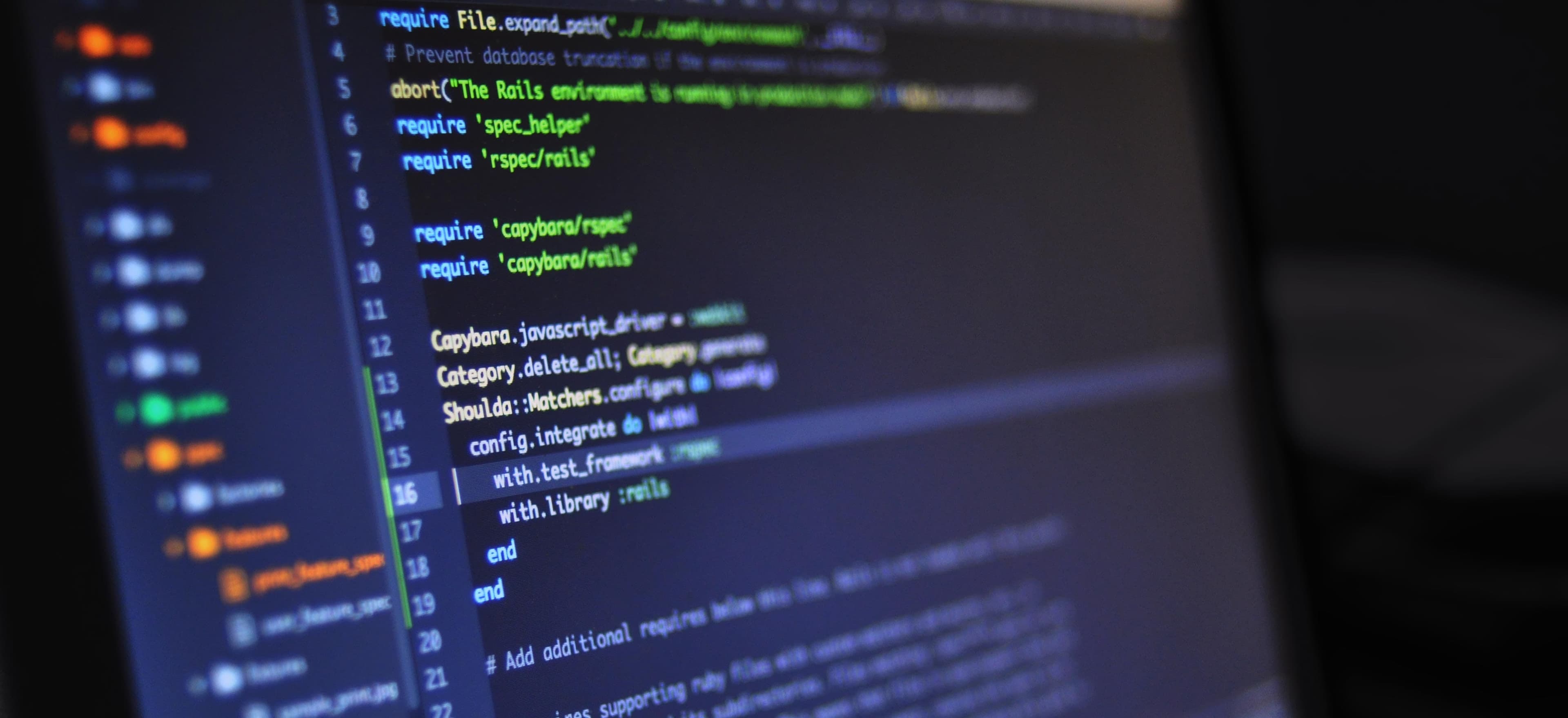
- Published on
Overcoming Common Challenges with Java Management Extensions
Java Management Extensions (JMX) is a powerful feature of the Java programming language that provides a robust way to manage and monitor Java applications. However, like any technology, it comes with its own set of challenges. In this article, we will explore these common challenges and how to effectively overcome them, ensuring your JMX implementations are efficient, secure, and easy to manage.
What are Java Management Extensions?
JMX is part of the Java Standard Edition (Java SE). It facilitates the management and monitoring of Java applications through MBeans (Managed Beans), which represent resources such as applications or subsystems. MBeans are simple Java objects that follow specific naming conventions to expose their attributes and methods for external management.
For more information on JMX and its components, visit the Oracle documentation.
Key Benefits of JMX
- Real-time Monitoring: JMX allows for real-time monitoring of applications, enabling administrators to check the performance and health of their systems.
- Dynamic Management: You can dynamically configure applications without stopping them, which is crucial for high-availability systems.
- Integration: JMX has built-in capabilities for integration with management tools like JConsole and VisualVM, making it a versatile choice.
Common Challenges
- Complexity of Implementation
- Security Concerns
- Performance Overhead
- CLI Integration
- Versioning Issues
1. Complexity of Implementation
Implementing JMX can be complex due to its architecture and the need to understand MBeans, ObjectName, and the JMX Connector.
Solution
To simplify implementation, follow a structured approach. You can create a clear plan of your MBeans, keeping them lightweight and focused on the attributes and operations critical to your application.
Here’s an example of a simple MBean:
public interface SystemConfigMBean {
String getLogLevel();
void setLogLevel(String level);
}
In this example, SystemConfigMBean
allows for the management of a logging level in real time. The interface defines getter and setter methods, which are essential as they provide the necessary functionality for monitoring and management.
To implement this MBean, you would create a class that implements the interface:
public class SystemConfig implements SystemConfigMBean {
private String logLevel = "INFO";
@Override
public String getLogLevel() {
return logLevel;
}
@Override
public void setLogLevel(String level) {
this.logLevel = level;
// Assume we have a logging framework that uses this level
System.out.println("Log level set to: " + level);
}
}
In this class, we encapsulate the log level variable and provide the implementation for our MBean methods. Such designs reduce complexity by promoting modular and maintainable code.
2. Security Concerns
JMX exposes management and monitoring features, which may pose security risks if not handled properly.
Solution
To tackle security issues, always enable JMX authentication and encryption. You can achieve this by configuring the Java application to demand user credentials for JMX access and to utilize SSL for encrypted communication.
To enable authentication, add the following system properties when starting your Java application:
-Dcom.sun.management.jmxremote
-Dcom.sun.management.jmxremote.port=9999
-Dcom.sun.management.jmxremote.authenticate=true
-Dcom.sun.management.jmxremote.ssl=true
-Dcom.sun.management.jmxremote.password.file=/path/to/jmx.password
-Dcom.sun.management.jmxremote.access.file=/path/to/jmx.access
In these properties:
jmxremote
enables the JMX agent.jmxremote.port
defines which port JMX listens on.authenticate
ensures that authentication is required.ssl
uses secure socket layer for encrypted data transfer.
Utilizing these features can significantly reduce your application's risk of unauthorized access.
3. Performance Overhead
Using JMX adds some overhead, especially if you're frequently accessing attributes and invoking operations on MBeans.
Solution
One effective method for minimizing performance impact is to use JMX selectively. Only expose MBeans that are necessary for monitoring or management. Furthermore, avoid polling MBeans too frequently. Here’s how you can reduce overhead:
private static final long POLLING_INTERVAL = 5000; // 5 seconds
Timer timer = new Timer();
timer.scheduleAtFixedRate(new TimerTask() {
@Override
public void run() {
// Example function to poll MBean value
String logLevel = systemConfigMBean.getLogLevel();
System.out.println("Current log level: " + logLevel);
}
}, 0, POLLING_INTERVAL);
In this code, we utilize a timer to poll the log level every five seconds. Be cautious with your polling interval based on your application's performance needs.
4. CLI Integration
Integrating JMX into Command-Line Interfaces (CLI) can be tricky, especially when dealing with Java applications running in different environments.
Solution
One practical approach is to use existing tools that leverage JMX, such as jconsole
and VisualVM
. These tools provide a user-friendly interface for connecting to JMX agents and can be launched with the following command:
jconsole
To connect to a remotely running JMX-enabled application, specify the hostname and port. This method allows for a seamless connection without worrying about the intricacies of CLI integration.
5. Versioning Issues
As applications evolve, maintaining version compatibility can become a hassle, particularly if the signatures of MBeans change.
Solution
Adopt a versioning strategy for your MBeans. Include version numbers in the MBean interface and ensure backward compatibility where possible.
Here’s an example of a versioned MBean interface:
public interface SystemConfigV2MBean {
String getLogLevel();
void setLogLevel(String level);
int getVersion(); // Added method for versioning
}
Here, the getVersion
method provides insight into which version of your MBean is in use, allowing better management of changes over time.
The Bottom Line
Java Management Extensions provide valuable capabilities for monitoring and managing Java applications. While common challenges like complexity, security, performance, CLI integration, and versioning may arise, utilizing best practices can effectively address these challenges.
By keeping your MBeans simple, securing connections, managing performance proactively, leveraging existing tools, and implementing versioning strategies, you can unlock the full potential of JMX in your development environment.
For further reading on performance optimization in Java applications, visit Baeldung’s guide on Java profiling.
By following the practices outlined here, your journey with Java Management Extensions will be smoother and more efficient, resulting in applications that are not only manageable but also robust and secure. If you have additional tips or challenges you've faced with JMX, feel free to share your thoughts in the comments!
Checkout our other articles