Common Java Mistakes Beginners Need to Avoid
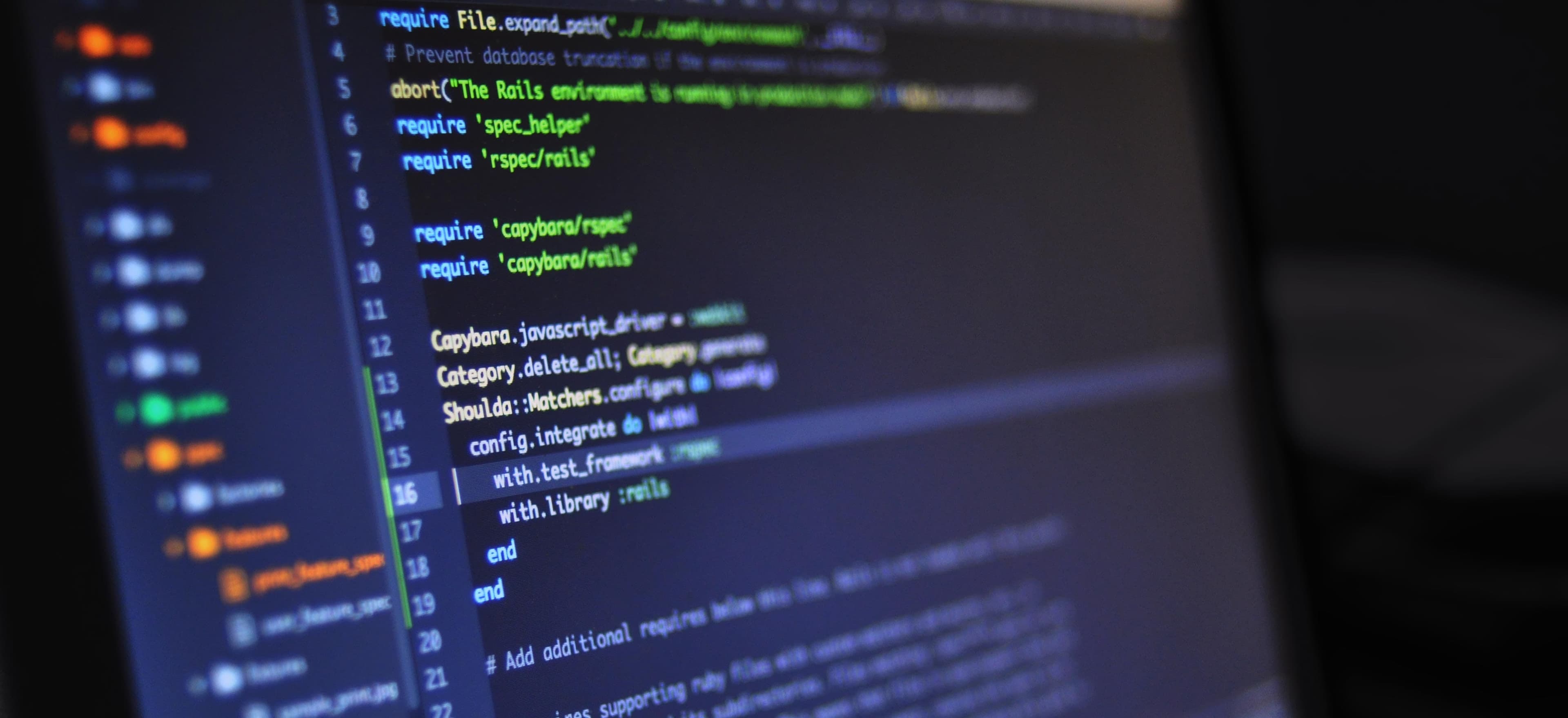
- Published on
Common Java Mistakes Beginners Need to Avoid
Java is one of the most popular programming languages worldwide, appreciated for its portability, performance, and rich feature set. However, when starting out, many beginners encounter pitfalls that can hinder their learning process and lead to ineffective code. In this blog post, we will explore common mistakes that Java newcomers make and provide insights into how to avoid them.
1. Ignoring the Importance of Understanding Object-Oriented Programming (OOP)
Java is inherently an object-oriented language. A common mistake is to write procedural code without leveraging the principles of OOP—encapsulation, inheritance, and polymorphism.
Why It Matters
Understanding OOP is crucial because it helps in managing complex systems by dividing them into manageable classes and objects. Not leveraging OOP can make code less organized and harder to maintain.
Example Code
// Poor use of OOP: procedural approach
public class UserManager {
public void createUser(String name, String email) {
// creation logic
}
}
// Better OOP approach
public class User {
private String name;
private String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
public void displayUserInfo() {
System.out.println("User: " + name + ", Email: " + email);
}
}
In the second example, we encapsulate the user's attributes and behavior, leading to cleaner and more manageable code.
2. Not Using Proper Naming Conventions
Naming conventions in Java are essential for maintaining readability. Many beginners often overlook this simple rule.
Why It Matters
Good naming conventions improve code clarity and make it easier for others (and your future self) to understand the purpose of a variable or method.
Naming Conventions
- Classes: Use CamelCase (e.g.,
EmployeeDetails
) - Methods/Variables: Use lowerCamelCase (e.g.,
calculateSalary
) - Constants: Use ALL_CAPS with underscores (e.g.,
MAX_VALUE
)
Example Code
public class Employee {
private String firstName; // good
private String lastName; // good
public void calcSalary() { // bad naming
// calculation logic
}
}
Instead of calcSalary
, a more descriptive name like calculateAnnualSalary
would improve clarity.
3. Failing to Handle Exceptions Properly
Java uses a robust exception-handling model. Beginners often overlook proper exception handling, leading to applications that crash unexpectedly.
Why It Matters
Poor error handling can result in application failures that degrade user experience. Proper exception handling ensures that your application can manage runtime anomalies gracefully.
Example Code
// Poor exception handling
public void readFile(String fileName) {
File file = new File(fileName);
Scanner scanner = new Scanner(file); // throws FileNotFoundException
// read data
}
// Good exception handling
public void readFile(String fileName) {
try {
File file = new File(fileName);
Scanner scanner = new Scanner(file);
// read data
} catch (FileNotFoundException e) {
System.out.println("File not found: " + fileName);
}
}
In the second example, the program gracefully informs the user about the specific issue rather than crashing.
4. Misusing the ==
Operator for String Comparison
String comparison can be tricky in Java. Many beginners mistakenly use ==
instead of the .equals()
method.
Why It Matters
Using ==
checks for reference equality, not value equality, which can lead to unexpected results when comparing strings.
Example Code
String str1 = new String("Hello");
String str2 = new String("Hello");
// This will be false
boolean areEqual = (str1 == str2);
// This will be true
boolean areEqualValue = str1.equals(str2);
Always use .equals()
when comparing string values!
5. Forgetting to Use final
Where Appropriate
Another common oversight is the misuse of the final
keyword. Novice programmers often forget to use final
for constants or class variables that should not change.
Why It Matters
Declaring variables as final
prevents unintentional changes, leading to safer and cleaner code.
Example Code
public class Constants {
public static final int MAX_USERS = 100; // good
public int maxUsers; // bad, should be final
}
Using final
makes your intent clear and enforces immutability.
6. Not Utilizing Java Collections Framework Effectively
Java provides a robust Collections Framework. Beginners often misuse arrays where collections would be more appropriate.
Why It Matters
Collections provide versatility and built-in methods for common operations, improving code efficiency and readability.
Example Code
// Using an array
String[] names = new String[5];
names[0] = "Alice";
names[1] = "Bob";
// Using ArrayList from Collections Framework
List<String> namesList = new ArrayList<>();
namesList.add("Alice");
namesList.add("Bob");
Using ArrayList
not only simplifies your code but also provides numerous methods for data manipulation.
7. Neglecting Comments and Documentation
Many beginners write lengthy pieces of code without commenting adequately.
Why It Matters
Without comments, understanding the logic of the code becomes increasingly difficult, especially in complex programs or when working in teams.
Example Code
// Bad commenting
public void process() {
// processing logic
}
// Good commenting
/**
* Processes user data and performs necessary operations.
*/
public void process() {
// processing logic
}
Comments should explain why you did something, not what you did.
The Closing Argument
Avoiding these common Java mistakes will help beginners write cleaner, more efficient, and maintainable code. Remember to invest time in understanding object-oriented programming, properly manage exceptions, utilize good practices in naming conventions, and take full advantage of the Java Collections Framework.
To deepen your learning, consider exploring Java Fundamentals on the Java documentation website or practicing with exercises from Codecademy.
By being aware of these common pitfalls and actively working to avoid them, you can enhance your skills and become a proficient Java developer. Happy coding!