Common Log4j 2 Customization Mistakes to Avoid
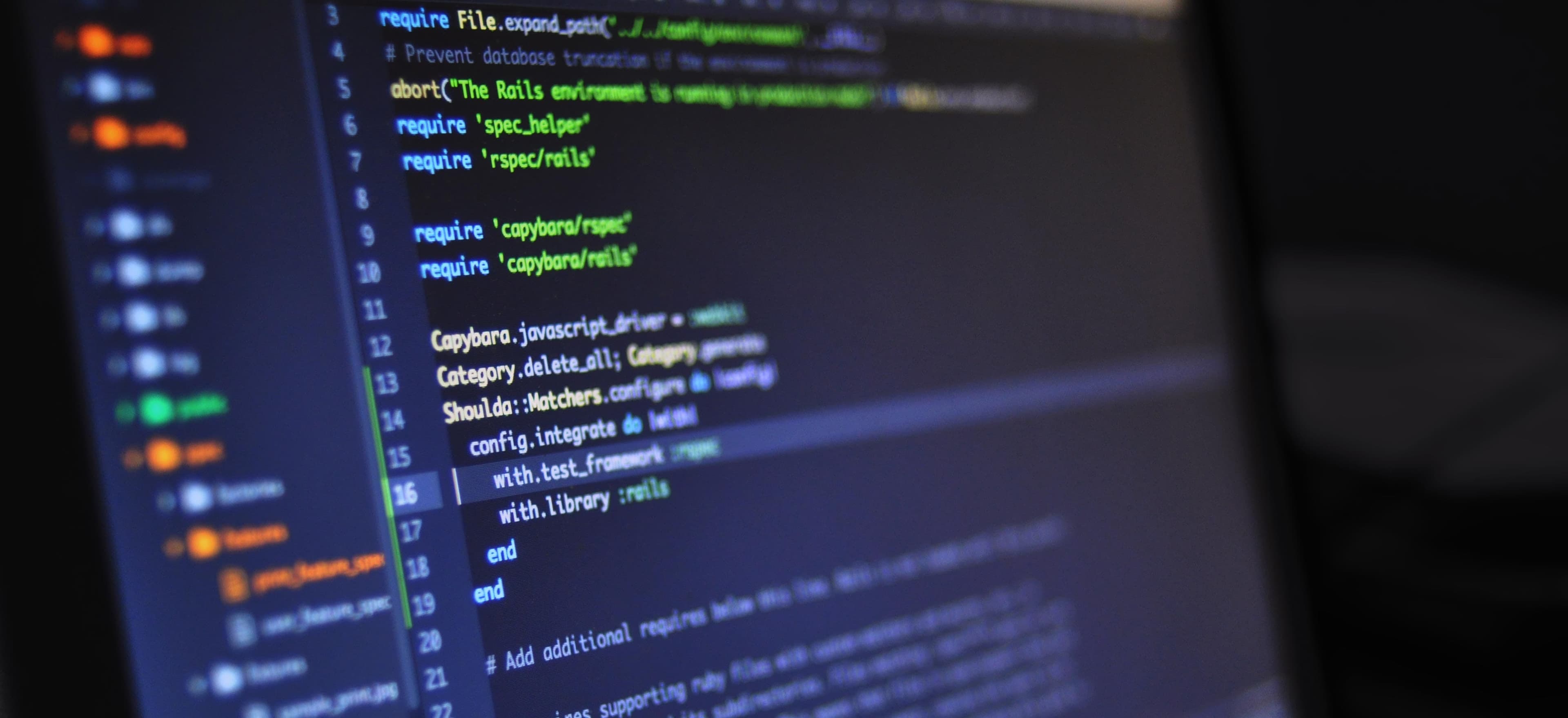
- Published on
Common Log4j 2 Customization Mistakes to Avoid
Logging is an essential aspect of any application, providing crucial insights into its health, performance, and behavior. As many Java developers rely on Log4j 2 for logging, it’s important to understand how to customize it effectively. While Log4j 2 offers great flexibility, improper configurations can lead to significant mistakes. In this post, we will explore some common Log4j 2 customization mistakes to avoid, ensuring that your logging setup is as robust and effective as possible.
Why Use Log4j 2?
Log4j 2 is a popular logging framework for Java applications, offering asynchronous logging, various logging levels, and the ability to log to different outputs such as files, databases, and consoles. By leveraging Log4j 2, developers can capture and manage logs more efficiently, thus improving the monitoring and debugging process.
Benefits of Using Log4j 2
- Performance: With asynchronous logging, your application can process logs without major performance hits.
- Flexibility: Extensive configuration options allow you to tailor your logging setup to meet specific needs.
- Extensibility: Easily create custom appenders, filters, or layouts to enhance logging.
Common Mistakes to Avoid
1. Not Using the Correct Logging Level
One of the first mistakes developers make is not using the appropriate logging level. Log4j 2 provides several logging levels: TRACE, DEBUG, INFO, WARN, ERROR, and FATAL. Each serves a different purpose.
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class Example {
private static final Logger logger = LogManager.getLogger(Example.class);
public void run() {
logger.debug("Debugging information, useful for development.");
logger.info("Informational message, something to note.");
logger.warn("Warning: this might be an issue.");
logger.error("Error occurred, something went wrong!");
}
}
Using the wrong logging level can lead to excessive noise or missed important information. Always think about the intention behind the logs. For example, debug-level logs should primarily aid development, and less critical information should not clutter production-level logs.
2. Ignoring Asynchronous Logging
Many developers overlook the performance benefits of asynchronous logging. When synchronous logging occurs, every log request blocks the thread until the logging operation completes. This can result in performance bottlenecks.
By configuring asynchronous logging, you can decouple log writing from your application’s primary flow, enhancing performance. Here’s a simple configuration in a log4j2.xml
file:
<Appenders>
<Async name="AsyncAppender">
<AppenderRef ref="FileAppender"/>
</Async>
<File name="FileAppender" fileName="app.log">
<PatternLayout pattern="%d{YYYY-MM-dd HH:mm:ss} %-5p %c{1} - %m%n"/>
</File>
</Appenders>
This XML configuration snippet sets up an asynchronous appender that sends logs to a file. It ensures that the application can continue processing requests while log entries are handled in the background.
3. Hardcoding Log File Paths
Another common mistake is hardcoding the paths of log files. This can cause issues when deploying applications across different environments. Instead, leverage properties files or environment variables to define your log paths.
For instance, instead of hardcoding:
<File name="FileAppender" fileName="/var/log/myapp/app.log">
You can use a variable:
<File name="FileAppender" fileName="${sys:log.file.path}">
Before running your application, you can set log.file.path
through system properties. This approach enhances the flexibility of your logging setup and allows for different paths in different environments (development, testing, and production).
4. Overloading the Logging Configuration
While it can be tempting to have an extensive logging configuration, overdoing it can lead to confusion and maintenance difficulties. Keep configurations clean and straightforward.
Ensure that each appender has a specific purpose, and avoid creating too many appenders or filters that complicate the understanding of your logging behavior. Here’s a minimalist approach:
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %p %c{1} - %m%n"/>
</Console>
<File name="File" fileName="logs/app.log">
<PatternLayout pattern="%d{YYYY-MM-dd} [%t] %-5level %logger{36} - %msg%n"/>
</File>
</Appenders>
In this example, two appenders (console and file) are created with clear objectives and efficient patterns.
5. Forgetting to Rotate Log Files
Log files can grow quickly in size, especially in applications with high logging volume. Forgetting to implement log rotation can lead to disk space issues. Log4j 2 supports rolling file appenders that can help manage log file sizes effectively.
By using RollingFileAppender
, specify policies for when to roll over files:
<RollingFile name="RollingFile" fileName="logs/app.log"
filePattern="logs/app-%d{yyyy-MM-dd}-%i.log.gz">
<PatternLayout>
<pattern>%d{yyyy-MM-dd HH:mm:ss} %p %c{1} - %m%n</pattern>
</PatternLayout>
<Policies>
<SizeBasedTriggeringPolicy size="10MB"/>
<TimeBasedTriggeringPolicy interval="1" modulate="true"/>
</Policies>
</RollingFile>
In this example, the log file rolls over once it reaches 10 MB or every day at midnight, creating compressed backup files.
6. Not Testing Your Logging Configuration
A mistake often seen is the lack of testing. After customizing your Log4j configuration, failure to verify that it operates as intended can lead to missed logs or non-performance. Always load test your logging setups under realistic conditions.
You might want to incorporate tests in your CI/CD pipeline. Ensure your logging works well during various application states. This proactive approach helps identify logging issues before they reach production environments.
7. Failing to Monitor Log Files
It's not enough to just configure and forget your logging system; it also needs to be monitored. Overlooking log monitoring means missing out on valuable insights and not catching issues early.
Utilize tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk to aggregate and visualize logs. By monitoring logs, you can better understand application behavior and swiftly react to anomalies.
Final Considerations
Customizing Log4j 2 can significantly enhance the logging capabilities of your Java application, but it's critical to avoid common mistakes that could undermine these benefits. By selecting appropriate logging levels, leveraging asynchronous logging, preventing hardcoded file paths, maintaining a clean configuration, implementing log rotation, testing effectively, and monitoring logs, you can create a robust logging environment that fits your application’s needs.
For more detailed information on Log4j configuration, consider visiting the official Log4j 2 documentation and the helpful Log4j 2 GitHub repository.
By implementing these practices, you’ll not only optimize logging in your application but also enhance your troubleshooting capabilities and overall system performance. Happy logging!