Common Pitfalls in Spring Transactions to Avoid
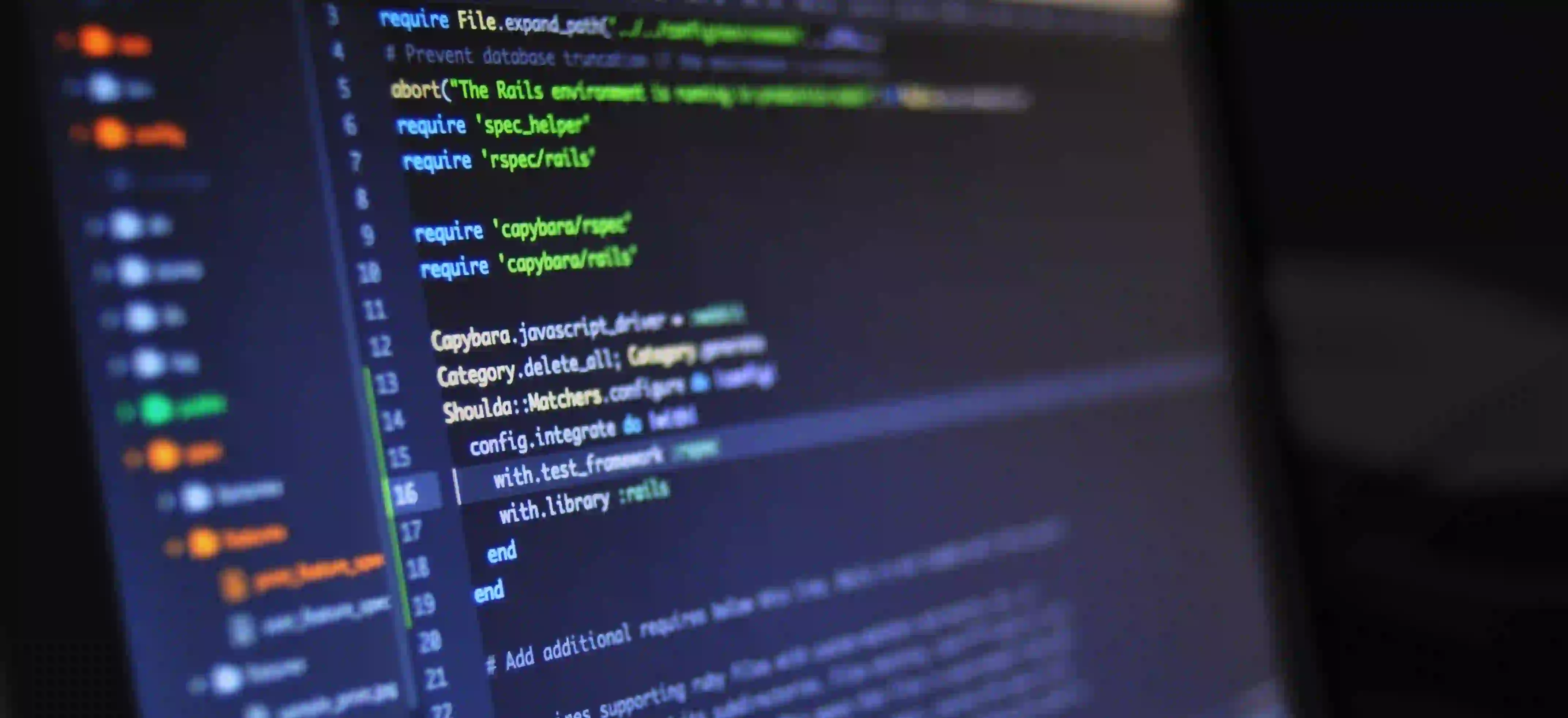
Common Pitfalls in Spring Transactions to Avoid
Spring Framework offers robust support for declarative transaction management, making it easier to manage the complexities of accessing databases in Java applications. However, understanding the transaction management principles can sometimes be challenging. Misusing Spring's transactions can lead to pervasive issues such as data inconsistencies, performance bottlenecks, and obscure bugs. In this blog post, we will discuss common pitfalls in Spring transactions that developers should avoid, providing clear explanations and practical code snippets to guide you.
Understanding Spring Transactions
Before diving into the pitfalls, let's briefly discuss what transactions are in the context of Spring. A transaction is a sequence of operations performed as a single logical unit of work. In the event of a failure, all operations should either complete successfully or none at all. This behavior is governed by the ACID properties (Atomicity, Consistency, Isolation, Durability).
In Spring, two primary approaches are used to manage transactions:
- Programmatic Transaction Management: You manually manage transactions.
- Declarative Transaction Management: You use annotations or XML configurations, allowing Spring to manage transactions for you.
While both methods are powerful, they come with their own sets of pitfalls. Let's explore some of the most common pitfalls and how to avoid them.
Pitfall 1: Not Understanding Transaction Propagation
One of the most common mistakes is misunderstanding transaction propagation levels. Spring's transaction propagation settings determine how transactions interact with each other.
For example, the @Transactional
annotation can be configured with various propagation behaviors such as:
REQUIRED
: Joins an existing transaction or creates a new one if none exists (default).REQUIRES_NEW
: Always creates a new transaction, suspending any existing one.NESTED
: Executes within a nested transaction (if supported).
Code Example
@Service
public class UserService {
@Transactional(propagation = Propagation.REQUIRED)
public void registerUser(User user) {
// Existing transaction is used or a new one is created.
userRepository.save(user);
notifyUser(user);
}
@Transactional(propagation = Propagation.REQUIRES_NEW)
public void notifyUser(User user) {
// Always executes in a new transaction.
emailService.sendRegistrationEmail(user);
}
}
Why It Matters
Understanding transaction propagation can prevent lost updates and ensure the integrity of your operations. For example, if you make a call to a method annotated with REQUIRES_NEW
within a REQUIRED
transaction, the new transaction will not roll back if the outer transaction fails.
Pitfall 2: Not Handling Rollbacks Correctly
Another frequent error involves incorrect rollback behavior. By default, Spring only rolls back transactions for unchecked exceptions (subclasses of RuntimeException
) and Error
. To roll back for checked exceptions, you must explicitly specify that using the rollbackFor
attribute.
Code Example
@Transactional(rollbackFor = { Exception.class })
public void updateUser(User user) throws Exception {
userRepository.update(user);
if (!isValid(user)) {
throw new Exception("User data is invalid");
}
}
Why It Matters
Failing to configure rollback behavior can leave your database in an inconsistent state. Always check whether your application needs to roll back for specific exceptions, especially during data validation or business logic checks.
Pitfall 3: Ignoring Transaction Isolation Levels
Transaction isolation levels dictate how transaction integrity is visible to other transactions. Spring supports various isolation levels, such as READ_COMMITTED
, READ_UNCOMMITTED
, and SERIALIZABLE
.
Ignoring isolation levels can lead to issues like dirty reads, non-repeatable reads, and phantom reads.
Code Example
@Transactional(isolation = Isolation.SERIALIZABLE)
public void performCriticalSection() {
// All operations within this method will be executed with the highest isolation level.
accountService.debitAccount();
accountService.creditAccount();
}
Why It Matters
Choosing the appropriate isolation level is vital for data integrity and performance. Higher isolation levels can lead to performance bottlenecks due to locking, while lower levels may expose the application to data inconsistency.
Pitfall 4: Avoiding Transaction Boundaries
Another common pitfall is failing to correctly define transaction boundaries. A transaction must encompass all operations that should be committed or rolled back atomically.
@Service
public class OrderService {
@Transactional
public void processOrder(Order order) {
// Place order
orderRepository.save(order);
// Update inventory
inventoryService.updateInventory(order);
// May throw checked exceptions
try {
paymentService.processPayment(order);
} catch (PaymentException e) {
// Handle payment failure without interrupting transaction
}
}
}
Why It Matters
Failure to establish clear transaction boundaries can lead to unexpected behaviors. If an operation outside of the transactional context fails, it may cause inconsistent data states when other operations were already committed.
Pitfall 5: Mixing Multiple Data Sources
When using multiple databases, mixing transaction management across them can lead to issues. Spring's transaction management may not work seamlessly across different databases, resulting in inconsistencies.
For example, if you're using a relational database alongside a NoSQL database, managing transactions between them requires distinct strategies.
Code Example
@Transactional
public void transferFunds(Long fromAccount, Long toAccount, Double amount) {
// Update in the SQL database
accountRepository.debitAccount(fromAccount, amount);
// A NoSQL operation that does not participate in the same transaction
noSqlOrderService.logTransfer(fromAccount, toAccount, amount);
}
Why It Matters
Always assess your transaction model when working with multiple data sources. Leveraging distributed transactions with tools like Spring Cloud or compensatory transactions may be necessary to manage operations across diverse types of databases.
Key Takeaways
Spring's transaction management features provide powerful tools for ensuring data integrity in your Java applications. However, being aware of common pitfalls is essential for harnessing the full power of transactions.
- Understand transaction propagation to prevent unintended data states.
- Configure rollback behavior correctly to maintain integrity.
- Select the appropriate transaction isolation level for your operations.
- Define clear transaction boundaries for atomic operations.
- Be cautious when dealing with multiple data sources.
By following these best practices, you can optimize your Spring applications for better performance and reliability. For further reading, refer to the official Spring Documentation and explore community-contributed Spring Boot Transaction Management resources.
Thus armed with knowledge, may your Spring applications thrive, free from the common pitfalls that can hamper their success. Happy coding!