Mastering Command Line Options in Java: Common Pitfalls
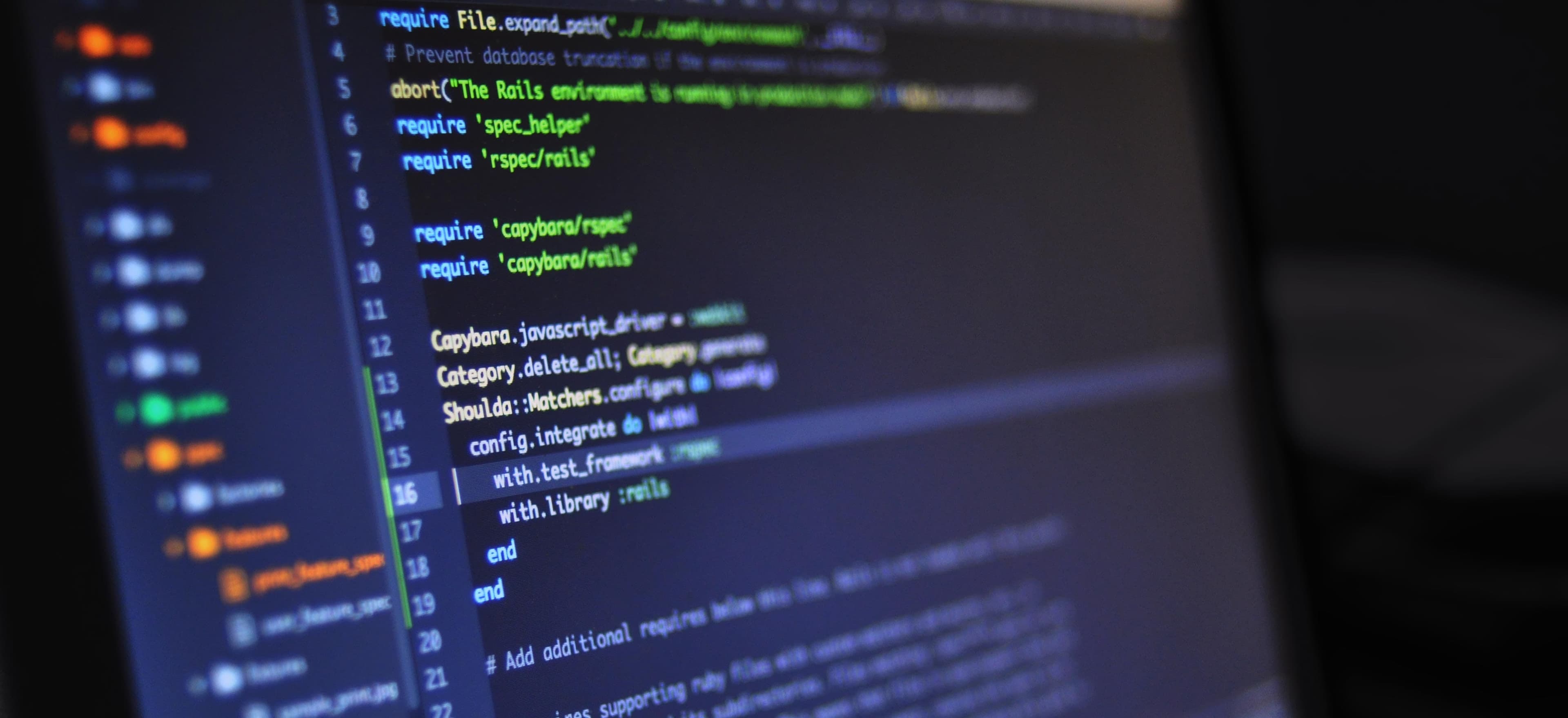
- Published on
Mastering Command Line Options in Java: Common Pitfalls
Java is a versatile and powerful programming language that enables developers to create robust applications. One of the often-underestimated features of Java is its ability to handle command line options. Command line arguments allow you to pass parameters to your Java application, enabling dynamic behavior without recompiling code. However, while utilizing command line options, developers frequently encounter common pitfalls. In this blog post, we'll explore these pitfalls and discuss best practices to avoid them, complemented by code examples to highlight their significance.
Understanding Command Line Options in Java
When launching a Java application, you can provide additional parameters using the command line. These parameters can modify the application’s behavior or pass data necessary for execution. In Java, these parameters are accessible via the args
array of the main
method.
Example of Command Line Options
Here’s a simple example that demonstrates how command line options work in Java:
public class CommandLineExample {
public static void main(String[] args) {
for (String arg : args) {
System.out.println("Argument: " + arg);
}
}
}
Why Use Command Line Options? Command line options help make your applications flexible and reusable. You can pass various data sets without modifying the application code, making it extremely useful for different environments and scenarios.
Usage
To run this application, you would execute:
java CommandLineExample arg1 arg2 arg3
The output would be:
Argument: arg1
Argument: arg2
Argument: arg3
Common Pitfalls
1. Not Checking for Argument Length
One of the most common mistakes is neglecting to check if the expected number of arguments has been provided before using them. Accessing an index that does not exist will lead to ArrayIndexOutOfBoundsException
.
Example of Pitfall
public class ArgumentCheck {
public static void main(String[] args) {
// This line can throw an exception if no arguments are provided
System.out.println("First argument: " + args[0]);
}
}
Fix
Always check the length of the args
array before accessing its elements:
public class SafeArgumentCheck {
public static void main(String[] args) {
if (args.length > 0) {
System.out.println("First argument: " + args[0]);
} else {
System.out.println("No arguments provided!");
}
}
}
Why Check for Length? This practice prevents runtime exceptions and enhances user experience by providing useful feedback when inputs are missing.
2. Improper Argument Parsing
Misunderstanding the structure of command line arguments can lead to incorrect parsing, resulting in unexpected behavior. For example, separating multiple values improperly can cause logical errors.
Example of Pitfall
Consider the following scenario where we want to pass multiple values for -name
option incorrectly.
public class ImproperParsing {
public static void main(String[] args) {
String name = args[1]; // Assuming -name is always at index 1
System.out.println("Name: " + name);
}
}
Fix
A better approach is to use a command line parser library, which can gracefully handle options and arguments. One such library is Apache Commons CLI.
import org.apache.commons.cli.*;
public class ProperParsing {
public static void main(String[] args) {
Options options = new Options();
options.addOption("n", "name", true, "User's name");
CommandLineParser parser = new DefaultParser();
try {
CommandLine cmd = parser.parse(options, args);
if (cmd.hasOption("n")) {
String name = cmd.getOptionValue("n");
System.out.println("Name: " + name);
}
} catch (ParseException e) {
System.out.println("Failed to parse command line arguments: " + e.getMessage());
HelpFormatter formatter = new HelpFormatter();
formatter.printHelp("utility-name", options);
}
}
}
Why Use a Library? Libraries like Apache Commons CLI provide robust error handling, help display usage instructions, and facilitate easier code management compared to manual parsing.
3. Ignoring Data Types
Another pitfall is assuming that all command line arguments are strings. When expecting numbers, failing to convert them appropriately can lead to runtime exceptions.
Example of Pitfall
public class TypeAssumption {
public static void main(String[] args) {
int number = Integer.parseInt(args[0]); // Will throw NumberFormatException if not an integer
System.out.println("Number: " + number);
}
}
Fix
Use proper error handling to manage incorrect types:
public class SafeTypeAssumption {
public static void main(String[] args) {
if (args.length > 0) {
try {
int number = Integer.parseInt(args[0]);
System.out.println("Number: " + number);
} catch (NumberFormatException e) {
System.out.println("Please provide a valid integer.");
}
} else {
System.out.println("No arguments provided!");
}
}
}
Why Handle Types Properly? Validating and converting data types ensures your application does not crash unexpectedly and provides clear error messages for users.
4. Not Documenting Command Line Options
Failing to document your command line options can lead to confusion for users interacting with your application. It's essential to provide adequate help or instructions.
Example of Pitfall
public class NoDocumentation {
public static void main(String[] args) {
// No user guidance or help functionality
System.out.println("Usage: java NoDocumentation -n <name>");
}
}
Fix
Implement a help option or external documentation:
public class DocumentedOptions {
public static void main(String[] args) {
if (args.length == 0 || args[0].equals("-help")) {
System.out.println("Usage: java DocumentedOptions -n <name>");
System.out.println("-n: specify the user's name");
return;
}
// Handle other options...
}
}
Why Document? Providing clear usage instructions promotes good user experience and reduces frustration, potentially saving time for both users and developers.
Final Thoughts
Mastering command line options in Java is essential for building robust and flexible applications. By understanding common pitfalls and employing best practices, you can improve the reliability and usability of your applications. This involves checking argument lengths, proper parsing and type handling, and providing documentation.
For additional resources, consider exploring the Java Documentation and using libraries like Apache Commons CLI for handling command line arguments seamlessly.
Embrace these tips, and you will make your Java applications more user-friendly and resilient to errors that can arise from the command line.
Happy coding!