Creating a User-Friendly Android App: Common Design Pitfalls
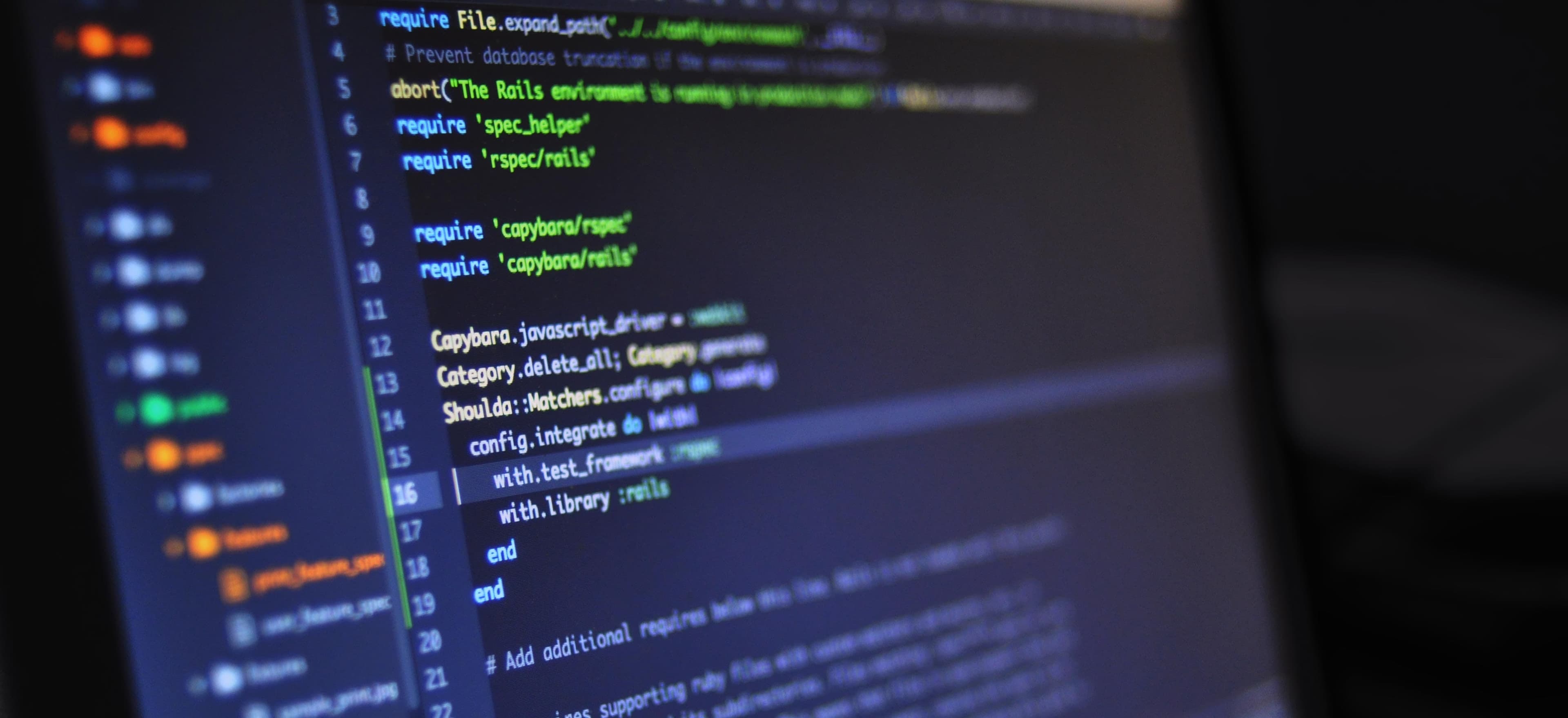
- Published on
Creating a User-Friendly Android App: Common Design Pitfalls
In today's tech-savvy world, developing a user-friendly Android app is not just an option; it is a necessity. The quality of user experience (UX) can determine whether your app becomes a resounding success or fades into obscurity. While Android development offers immense opportunities, developers often fall prey to common design pitfalls. In this blog post, we will explore these pitfalls and provide actionable insights on how to avoid them.
Understanding User-Centric Design
Before diving into the pitfalls, let’s briefly discuss the importance of user-centric design. UX design focuses on creating intuitive, easy-to-navigate interfaces that allow users to complete tasks efficiently. A user-friendly app minimizes frustration and maximizes satisfaction, fostering loyalty among users.
Why User Experience Matters
A study by Forrester Research reveals that a well-designed user experience could yield conversion rates up to 400%. This statistic alone underscores the financial implications of user experience on your app's success.
Common Design Pitfalls
-
Neglecting User Research
Many developers overlook the importance of user research. Understanding your target audience is essential to create an app that resonates with them. Skipping this step can lead to a disjointed design that does not meet user needs.
Solution: Conduct interviews, surveys, and usability tests to gather insights about user preferences. Utilize tools like Google Forms or Typeform for streamlined survey creation.
-
Overcomplicating Navigation
Excessive layers of navigation can confuse users. The principle of simplicity is dominant in UI/UX design. When users can't find what they need within a reasonable timeframe, they're likely to abandon the app.
Solution: Aim for an intuitive navigation structure. A good practice is to limit the number of taps needed to reach the main features of your app. Consider using a tab bar or a navigation drawer for easy access.
Here’s a simple example of how you can implement a navigation drawer in your Android app:
// In your MainActivity.java DrawerLayout drawerLayout = findViewById(R.id.drawer_layout); NavigationView navigationView = findViewById(R.id.nav_view); ActionBarDrawerToggle toggle = new ActionBarDrawerToggle(this, drawerLayout, R.string.navigation_drawer_open, R.string.navigation_drawer_close); drawerLayout.addDrawerListener(toggle); toggle.syncState(); navigationView.setNavigationItemSelectedListener(new NavigationView.OnNavigationItemSelectedListener() { @Override public boolean onNavigationItemSelected(@NonNull MenuItem item) { switch (item.getItemId()) { case R.id.nav_home: // Navigate to Home break; // Handle other menu items } drawerLayout.closeDrawer(GravityCompat.START); return true; } });
- Why: This code snippet creates a navigation drawer that allows users to navigate seamlessly throughout the app, enhancing user experience.
-
Ignoring Platform Guidelines
Each platform—Android, iOS, web—has its own design guidelines. Ignoring these can lead to an app that feels foreign or out-of-place. Android follows the Material Design principles that focus on usability and aesthetics.
Solution: Familiarize yourself with Material Design Guidelines and integrate these principles into your app to ensure a consistent look and feel across the platform.
-
Inconsistent Design Elements
Aesthetics play a significant role in user experience. Inconsistent design elements—like buttons, fonts, and colors—can create confusion and mistrust.
Solution: Establish a design system that includes a color palette, typography, and button styles to create uniformity across your app.
Here’s how to define a color palette in your
colors.xml
:<?xml version="1.0" encoding="utf-8"?> <resources> <color name="primaryColor">#FF5722</color> <color name="secondaryColor">#3F51B5</color> <color name="backgroundColor">#FFFFFF</color> </resources>
- Why: This centralized way of defining colors allows you to maintain consistency throughout the app, making it visually appealing and coherent.
-
Failure to Optimize for Performance
A sluggish app can ruin the user experience instantly. Users expect apps to be fast and responsive. Bloated code and heavy graphics can lead to lag and crashes.
Solution: Optimize your images, minimize the use of heavy libraries, and adopt best practices when coding.
Here’s how you might use Glide for efficient image loading:
// In your activity or fragment ImageView imageView = findViewById(R.id.image_view); Glide.with(this) .load("https://example.com/path/to/image.jpg") .into(imageView);
- Why: Glide is optimized for image loading and caching, improving the performance of your app without sacrificing user experience.
-
Overloading Push Notifications
While notifications can engage users, too many can annoy them. If users feel overwhelmed, they may disable notifications altogether or uninstall the app.
Solution: Use notifications judiciously and ensure that they provide real value. Segment your audience, and personalize notifications to enhance relevance.
-
Not Considering Accessibility
Accessibility is often overlooked in app design. Neglecting accessibility features prevents a significant portion of users from enjoying your app fully.
Solution: Incorporate accessibility features, such as screen reader compatibility and alternative text for images. Follow the Web Content Accessibility Guidelines to make sure your app caters to users of all abilities.
For example, you can enhance accessibility in your XML layout using the following properties:
<ImageView android:id="@+id/image_view" android:contentDescription="@string/image_description" android:src="@drawable/sample_image" />
- Why: By providing a content description, screen readers can convey the purpose of the image, allowing visually impaired users to navigate your app more effectively.
To Wrap Things Up
Creating a user-friendly Android app involves numerous considerations, with design being at the forefront. By understanding and avoiding common design pitfalls, developers can enhance user experience and engagement. Remember, the goal is to create an app that not only serves its purpose but does so in a manner that is enjoyable and easy for users.
By implementing the best practices outlined in this post, you're not just building an app; you're crafting an experience that speaks to your audience.
For additional resources and detailed guidance on Android development, check out the Android Developer Documentation or consider community-driven platforms like Stack Overflow for real-world insights and solutions. Happy coding!