Common Dozer Issues: Fixing Bean Mapping Errors
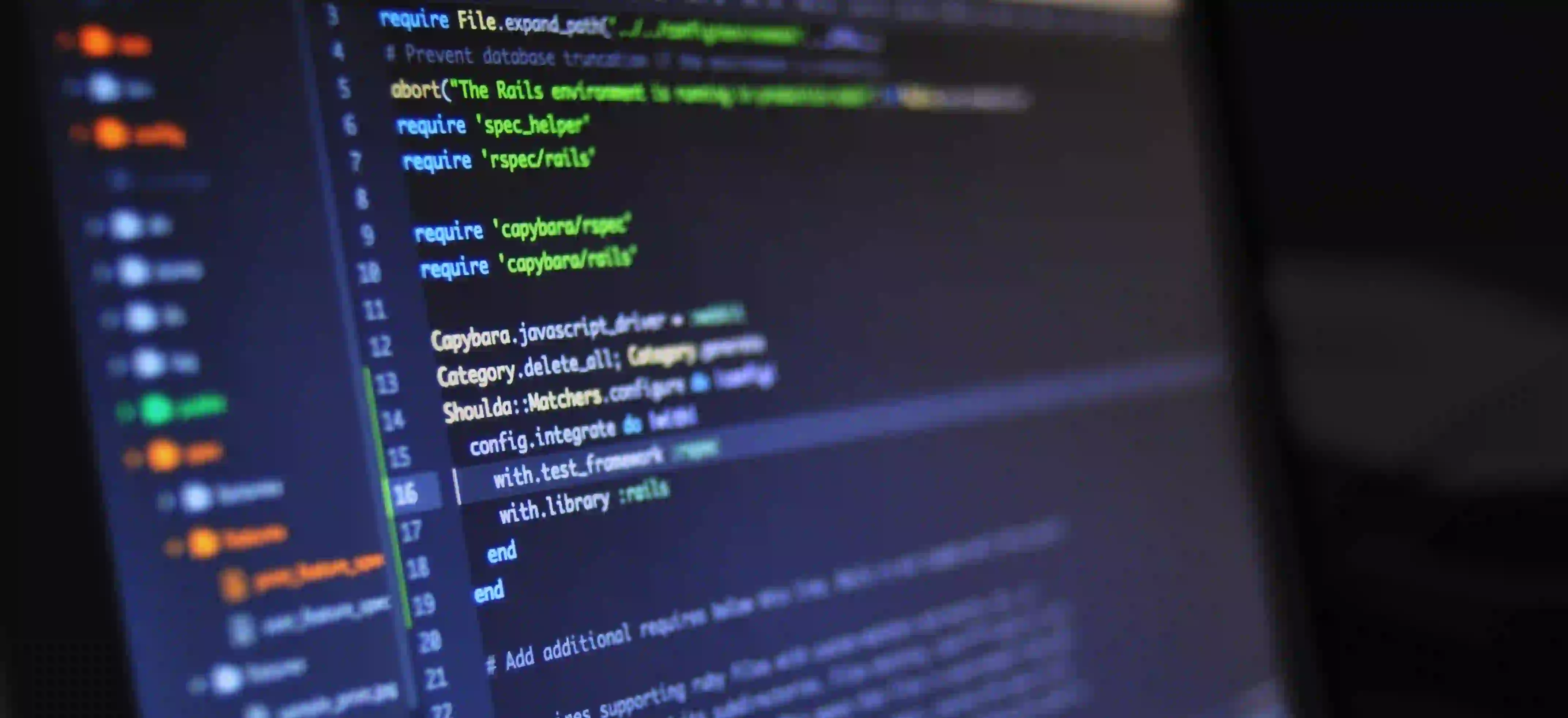
Common Dozer Issues: Fixing Bean Mapping Errors
Apache Dozer is a powerful Java library for mapping between Java beans, allowing developers to easily transform data structures. However, as with any powerful tool, developers can encounter common issues, especially with bean mapping errors. Understanding and resolving these errors is crucial for maintaining clean, efficient, and functional code. In this blog post, we’ll explore some of the most common Dozer mapping issues and provide solutions to help you navigate through them.
What is Dozer?
Dozer is a Java Bean to Java Bean mapping framework that uses reflection to recursively copy data from one object to another while preserving complex data types. It is favored for its simplicity and adaptability.
Common Dozer Issues
1. Unmapped Fields
One prevalent issue developers face is when a field in the source object doesn’t have a corresponding field in the target object, causing a NullPointerException or a missing data error.
Solution:
To solve this, ensure that both objects have matching fields or use Dozer XML Configuration to ignore specific fields. Here’s an example:
<mapping>
<class-a>com.example.SourceClass</class-a>
<class-b>com.example.TargetClass</class-b>
<field>
<a>sourceField</a>
<b>targetField</b>
</field>
<field>
<a>unmappedField</a>
<b>targetFieldToIgnore</b>
<a-hint>true</a-hint> <!-- This will ignore unmapped fields -->
</field>
</mapping>
Using the <a-hint>
field to ignore unmapped fields helps avoid runtime errors.
2. Collection Handling
Dozer sometimes struggles with mapping collection types between objects. For example, mapping a List of objects in the source to an array of objects in the target can lead to issues.
Solution:
To address this, ensure that your configurations correctly define how collections should be handled. Here’s an example of mapping a List to an array:
<mapping>
<class-a>com.example.SourceClass</class-a>
<class-b>com.example.TargetClass</class-b>
<field>
<a>sourceList</a>
<b>targetArray</b>
<a-hint>java.util.List</a-hint>
<b-hint>java.lang.Object[]</b-hint>
</field>
</mapping>
By specifying a-hint
and b-hint
, you provide Dozer with a clearer picture of how to handle the collections.
3. Type Mismatch
Type mismatch occurs when the source and target fields are of different types. For instance, attempting to map a String
type to an Integer
can cause a MappingException
.
Solution:
Always ensure that the types of mapped fields match. If necessary, implement custom converters. Here’s an example of a custom converter:
public class StringToIntegerConverter extends DozerConverter<String, Integer> {
public StringToIntegerConverter() {
super(String.class, Integer.class);
}
@Override
public Integer convertTo(String source, Integer destination) {
if (source == null) {
return null;
}
return Integer.parseInt(source);
}
@Override
public String convertFrom(Integer source, String destination) {
return source == null ? null : String.valueOf(source);
}
}
In your Dozer XML configuration file, you can then register the converter like this:
<mapping>
<class-a>com.example.SourceClass</class-a>
<class-b>com.example.TargetClass</class-b>
<field>
<a>sourceString</a>
<b>targetInteger</b>
<custom-converter>com.example.StringToIntegerConverter</custom-converter>
</field>
</mapping>
This tells Dozer to use the StringToIntegerConverter
when mapping between these types.
4. Nested Mappings
Mapping nested objects can often lead to complex issues. If nested properties are not properly configured, they may be ignored during the mapping process.
Solution:
Explicitly define nested field mappings in the Dozer XML configuration. Here’s an example:
<mapping>
<class-a>com.example.SourceClass</class-a>
<class-b>com.example.TargetClass</class-b>
<field>
<a>sourceNestedObject.sourceField</a>
<b>targetNestedObject.targetField</b>
</field>
</mapping>
In this case, Dozer knows to go deeper into the objects and map the nested fields accordingly.
5. Recursive Mappings
In cases where objects reference each other (like parent-child relationships), this can lead to stack overflow or infinite loops during mapping.
Solution:
To mitigate this, configure Dozer to handle recursion properly using the @DozerMapping
annotation. Here’s an example:
@DozerMapping
public class Parent {
private Child child;
}
@DozerMapping
public class Child {
private Parent parent;
}
Here, it's essential to ensure that appropriate handling is available within the Dozer configuration.
Bringing It All Together
Dozer is undoubtedly a powerful tool for bean mapping, but being aware of these common issues can significantly improve your experience and productivity. By understanding how to configure your mappings properly, utilize custom converters, and manage collections, you can fix bean mapping errors efficiently.
For further reading and resources, consider checking out the official Dozer documentation or looking into related libraries that might suit your specific mapping needs.
Remember, mapping is an intricate process, and encountering issues is part of the learning curve. With the right strategies and solutions, you can overcome these challenges effectively and create robust Java applications with clean data transformations. Happy coding!