Maximize Performance: Troubleshooting TTL Issues in Spring
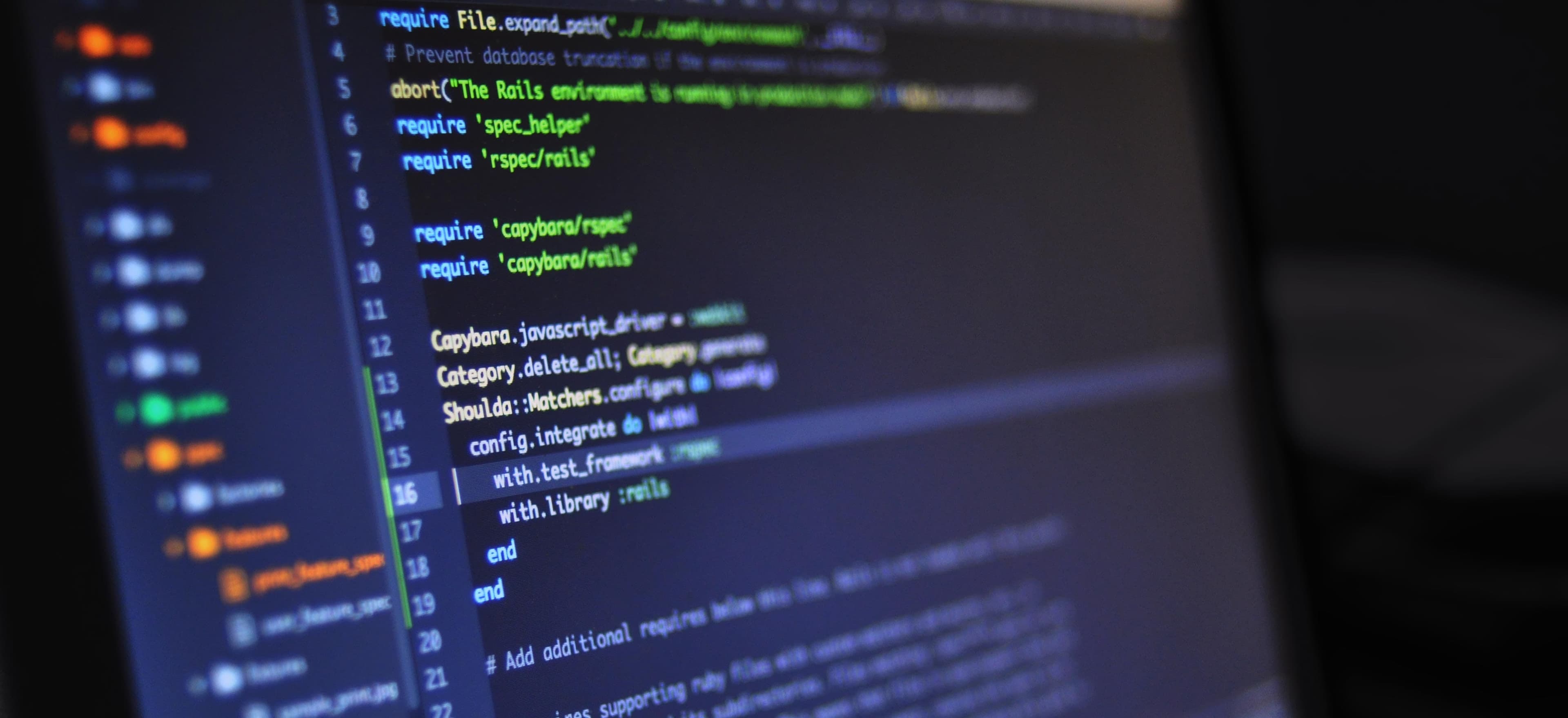
- Published on
Maximize Performance: Troubleshooting TTL Issues in Spring
In the realm of application performance, understanding and optimizing Time-To-Live (TTL) issues in Spring applications is pivotal for ensuring that your applications run smoothly and efficiently. Whether you're dealing with caching systems, database connections, or networking protocols, TTL impacts how long data resides in a particular state before it's either discarded or refreshed.
What is TTL?
Time-To-Live (TTL) is a concept rooted in networking and computing, defining the lifespan or duration of data in a computing environment. Once this period expires, the data becomes stale and is often removed or ignored. In Spring applications, TTL can significantly affect how caches store data, how elements are sent over networks, and how database connections are managed.
Understanding TTL is essential for both optimization and troubleshooting; it can be the difference between a responsive application and a slow, inefficient one.
Why is TTL Important?
- Resource Management: TTL helps in effective memory management by limiting the lifespan of objects in your application.
- Performance Optimization: A properly configured TTL can enhance application performance significantly by reducing the time to fetch data.
- Consistency and Freshness: TTL ensures that stale data does not get served to users, maintaining the integrity of the application.
Common TTL Issues
When dealing with TTL settings, a few specific issues can arise, complicating performance tuning:
-
Too Short TTL: Setting a TTL that is too short may cause frequent cache misses, leading to unnecessary loads on your database or external service.
-
Too Long TTL: Conversely, an excessively long TTL can deliver outdated information to users, making your application seem unreliable.
-
Inconsistent TTL Across Systems: Across distributed systems, having disparate TTL settings can lead to inconsistencies, which can hinder performance optimization.
-
Application-Specific Policies: Different parts of your application might require different TTL strategies, which could complicate your configuration if not handled properly.
Troubleshooting TTL Issues in Spring
The strategy to troubleshoot TTL-related issues in Spring involves a multifaceted approach, integrating logging, monitoring, and adjustment of TTL settings. Below are key steps to consider when optimizing TTL in Spring applications:
1. Monitor and Analyze Logs
The first step in troubleshooting involves gaining insights into how TTL configurations affect your application's performance.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class CacheService {
private static final Logger logger = LoggerFactory.getLogger(CacheService.class);
public void cacheData(String key, String value, long ttl) {
// Example logging for cache insertion
logger.info("Inserting key: {}, Value: {} with TTL: {} seconds", key, value, ttl);
// Code to insert data into cache
}
}
This example illustrates how to utilize logging effectively. It allows developers to trace the caching operation, including the TTL for each entry, providing insight into expired keys and cache misses.
2. Evaluate Your Caching Strategy
If you’re employing a caching solution like Spring Cache, Redis, or Ehcache, your configurations must suit your application's requirements.
# Sample Spring cache configuration in YAML
spring:
cache:
type: redis
redis:
time-to-live: 600000 # TTL in milliseconds
Setting an appropriate TTL in a caching configuration file is integral for optimizing performance. Ensure you regularly adjust TTL settings based on usage patterns, analyzing data access frequency and patterns to find the sweet spot for your TTL.
3. Configuration Inspection
Inspect configuration files to ensure no hard-coded TTL settings conflict. Spring properties, application.yml, or application.properties files may hold critical TTL values that require periodic review.
4. Use Spring Actuator
Integrating Spring Actuator can simplify monitoring your Spring application. It provides production-ready features to help you better understand how your application is performing.
import org.springframework.boot.actuate.autoconfigure.web.servlet.EndpointAutoConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Utilizing Actuator endpoints can reveal runtime metrics, including the effectiveness of your TTL settings.
5. Performance Testing
It is crucial to perform load testing to observe how changes to TTL impacts application performance under various conditions. Tools such as JMeter or Gatling can simulate usage scenarios that reveal insights into performance bottlenecks.
6. Adjusting TTL Dynamically
You might want to implement dynamic TTL settings based on user interaction patterns and system load. This can be achieved with a caching solution that supports dynamic expiry.
public void cacheDataWithDynamicTTL(String key, String value, long baseTTL) {
long dynamicTTL = calculateDynamicTTL(baseTTL);
cache.put(key, value, dynamicTTL);
logger.info("Caching {} with dynamic TTL: {}", key, dynamicTTL);
}
private long calculateDynamicTTL(long baseTTL) {
// Logic to calculate TTL based on system load or other metrics
return baseTTL; // Placeholder for actual logic
}
This method allows for flexibility based on application demands, meaning your application can adapt to changing conditions, optimizing performance in real-time.
Summary
Optimizing and troubleshooting TTL issues in Spring is not merely a one-time effort but a continuous process. It involves meticulous monitoring, effective logging practices, and a deep understanding of your application's data access patterns.
Remember, the goal of tweaking TTL settings is to strike a balance between keeping data fresh and reducing unnecessary loads on your systems. In addition, leveraging tools like Spring Actuator for monitoring and integrating dynamic TTL strategies can significantly enhance your application's efficiency.
By following these guidelines and maintaining a proactive approach to TTL management, you can maximize the performance of your Spring applications and ensure a smooth operation, ultimately elevating user experience. Happy coding!