Avoiding Common Antipatterns in Software Architecture
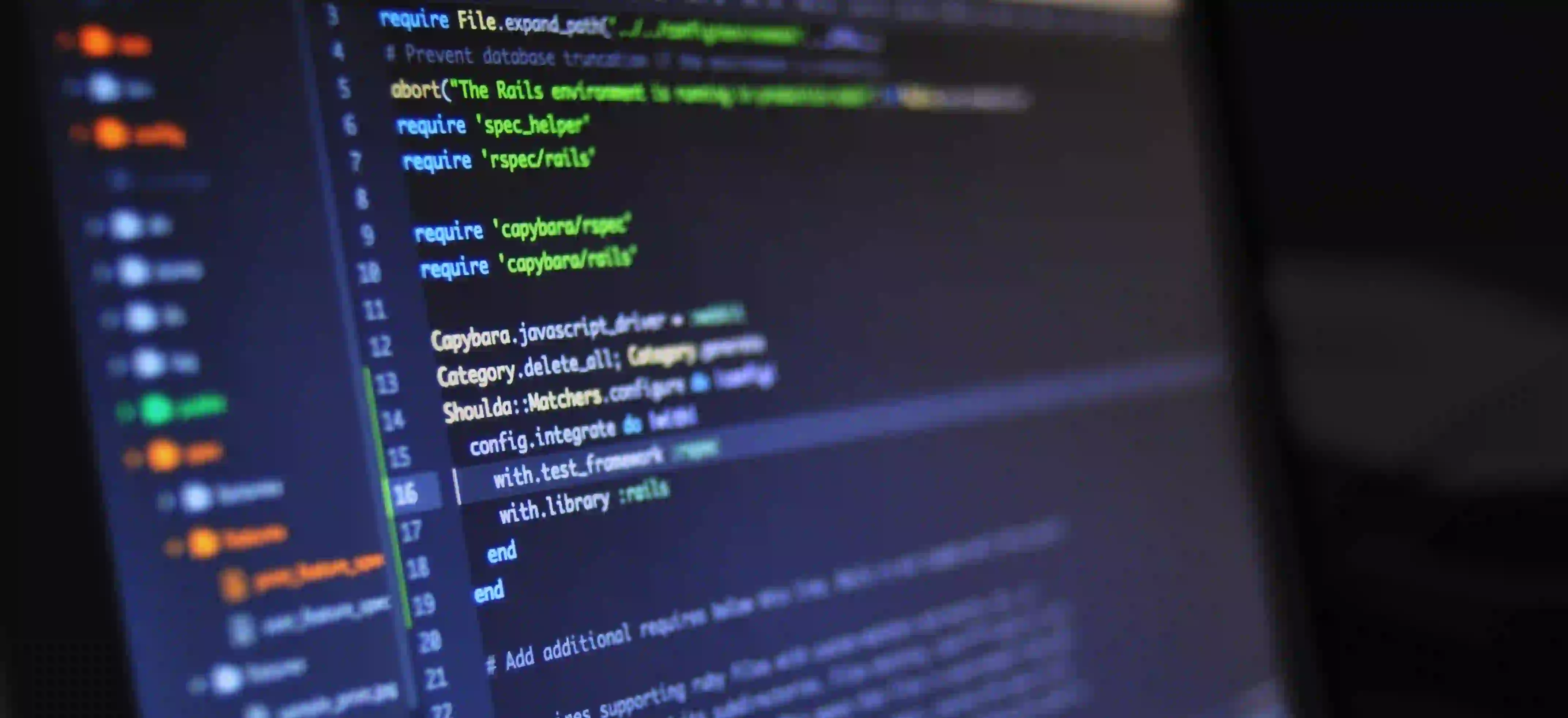
Avoiding Common Antipatterns in Software Architecture
Software architecture serves as the blueprint for a system, determining how components interact and ensuring that specific quality attributes are achieved. However, the path to a robust architecture is often littered with pitfalls. These pitfalls, known as antipatterns, can lead to inefficient systems, increased maintenance overhead, and a waste of valuable resources. In this blog post, we will explore some common software architecture antipatterns and provide strategies for avoiding them.
What is an Antipattern?
An antipattern is essentially a common response to a recurring problem that ends up being counterproductive. While it may seem like a reasonable approach at first glance, antipatterns tend to aggravate the issues they aim to resolve. Instead of providing effective solutions, they introduce complexity and hinder the system's performance and maintainability.
Common Antipatterns
1. God Object
The God Object antipattern occurs when a single class or module handles an overwhelming amount of responsibilities. This violates the Single Responsibility Principle (SRP) from SOLID principles, which states that a class should have only one reason to change.
Why is it a Problem?
A God Object can lead to tight coupling between components, making the code difficult to manage and test. Long-term, it leads to high maintenance costs and low reusability.
How to Avoid It
- Break down large classes into smaller, self-contained units.
- Identify distinct responsibilities and abstract them into different classes.
// Bad Example: God Object
public class UserManager {
public void createUser(String username) {
// Logic for user creation
}
public void deleteUser(String username) {
// Logic for user deletion
}
public void sendEmail(String email) {
// Logic to send email
}
public void logActivity(String username) {
// Logic to log user activity
}
// Other user-related functionalities...
}
In the example above, UserManager
handles too many responsibilities, which can complicate the class.
To avoid such issues, focus on smaller classes with clear responsibilities:
// Good Example: Single Responsibility
public class UserService {
public void createUser(String username) {
// Logic for user creation
}
public void deleteUser(String username) {
// Logic for user deletion
}
}
public class EmailService {
public void sendEmail(String email) {
// Logic to send email
}
}
public class LoggingService {
public void logActivity(String username) {
// Logic to log user activity
}
}
2. Spaghetti Code
Spaghetti code refers to code with a tangled, unorganized structure. It lacks clear logical flow and is hard to read and maintain.
Why is it a Problem?
As projects grow, spaghetti code becomes increasingly difficult to follow. Debugging, maintaining, or extending such code becomes a highly complex task.
How to Avoid It
- Adopt a clear coding standard and style guide.
- Encourage modular programming principles.
- Use libraries and frameworks that promote a structuring pattern, such as MVC (Model-View-Controller) for Java applications.
// Bad Example: Spaghetti Code
public void processUserInput(String input) {
if ("CREATE".equals(input)) {
// Logic to create user
} else if ("DELETE".equals(input)) {
// Logic to delete user
} else if ("UPDATE".equals(input)) {
// Logic to update user
}
// More and more conditions...
}
In this example, the process user input method is overwhelmed with conditions, making it hard to manage.
In contrast, you could use a command pattern to handle various user inputs cleanly:
// Good Example: Command Pattern
public interface Command {
void execute();
}
public class CreateUserCommand implements Command {
public void execute() {
// Logic to create user
}
}
public class DeleteUserCommand implements Command {
public void execute() {
// Logic to delete user
}
}
// Invoker
public class UserInputProcessor {
private Map<String, Command> commandMap;
public UserInputProcessor() {
commandMap = new HashMap<>();
commandMap.put("CREATE", new CreateUserCommand());
commandMap.put("DELETE", new DeleteUserCommand());
}
public void processUserInput(String input) {
Command command = commandMap.get(input);
if (command != null) {
command.execute();
}
}
}
3. Golden Hammer
The Golden Hammer antipattern occurs when a specific technology or solution is used for every problem, regardless of its appropriateness.
Why is it a Problem?
This can lead to inefficient solutions, as the chosen tool may not fit the problem at hand.
How to Avoid It
- Continuously evaluate the requirements of your system.
- Stay open to various tools and technologies.
- Invest time in understanding the strengths and weaknesses of available options.
For more comprehensive discussions about various software architecture strategies, refer to Martin Fowler's article on Architecture.
4. Shotgun Surgery
Shotgun Surgery happens when a single change in a system requires making multiple changes across various classes or modules.
Why is it a Problem?
This leads to inconsistency and increases the chances of bugs. Managing such scattered changes can also be cumbersome.
How to Avoid It
- Aim for high cohesion within classes and modules.
- Use Domain-Driven Design (DDD) to ensure behaviors are encapsulated where they belong.
// Bad Example: Shotgun Surgery
public void updateUserRole(String username, String role) {
userRepository.updateRole(username, role);
notificationService.notifyRoleChange(username, role);
auditService.logRoleChange(username, role);
}
In this example, updating a user’s role requires interacting with multiple services, thereby spreading changes across various classes.
Here's how you could encapsulate the behaviour:
// Good Example: Encapsulated Behaviour
public class User {
private String username;
private String role;
public void updateRole(String newRole) {
this.role = newRole;
notifyRoleChange(); // notify within the User class
logRoleChange(); // log within User class
}
private void notifyRoleChange() {
notificationService.notifyRoleChange(username, role);
}
private void logRoleChange() {
auditService.logRoleChange(username, role);
}
}
The Last Word
By remaining diligent and aware of these antipatterns, developers can create cleaner, more maintainable, and efficient software architectures. Instead of conforming to common pitfalls, aim for best practices that foster modular design, respect the principles of SOLID, and embrace continuous refactoring.
Remember, the goal is not merely to avoid antipatterns but to cultivate a thoughtful architecture that enables your application to grow, evolve, and meet user needs effectively. For further reading on good coding practices and architecture, consider checking out the online Software Engineering Institute resources.
By focusing on these guidelines, you can build a software architecture that anticipates change and is robust enough to endure the test of time. Happy coding!