Mastering Java Switch Expressions: Common Pitfalls Explained
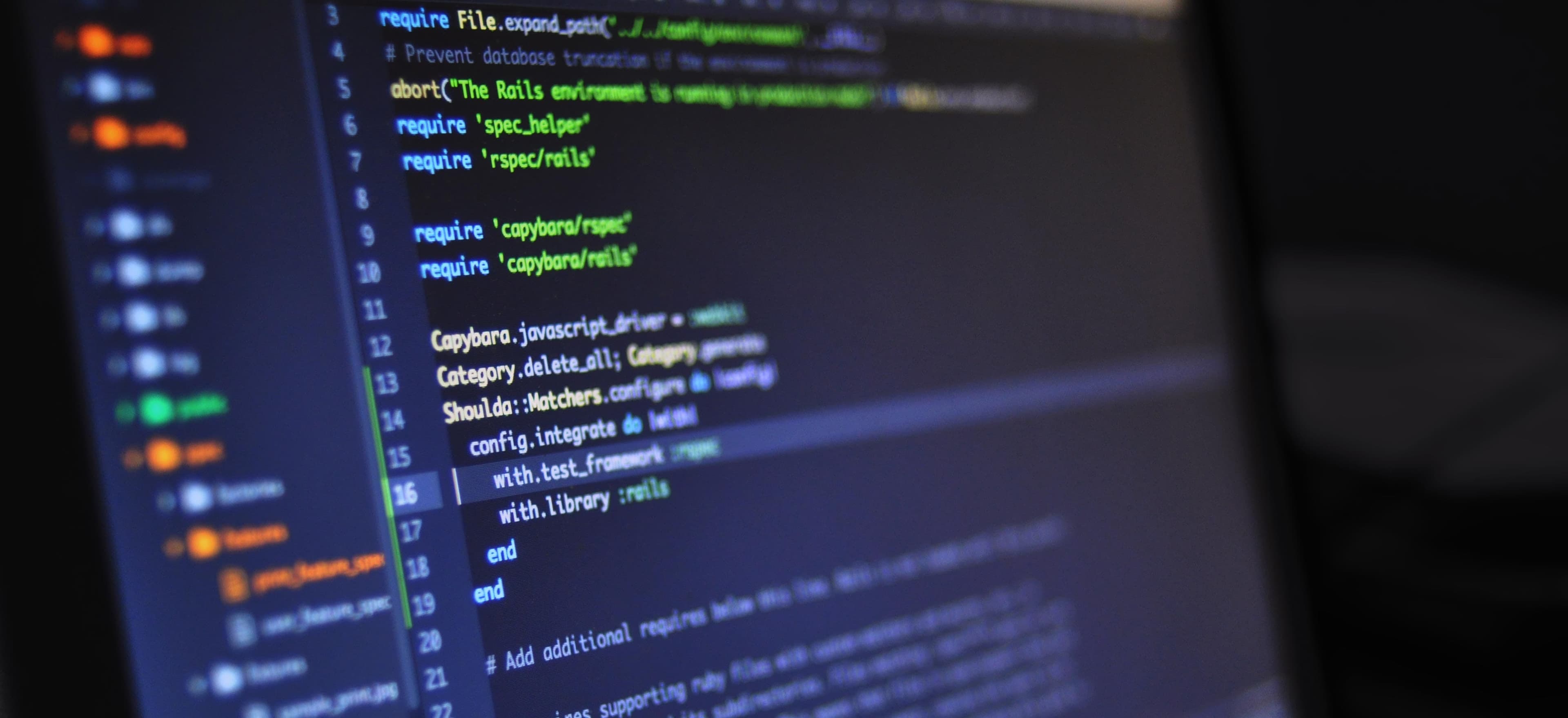
- Published on
Mastering Java Switch Expressions: Common Pitfalls Explained
In recent years, Java has evolved significantly, introducing new features that enhance productivity and code readability. One of these features is the switch expression, introduced in Java 12 as a preview feature and finalized in Java 14. While switch statements have been a staple in Java since the early days, switch expressions bring many enhancements to the table. However, with great power comes great responsibility. In this post, we will delve into mastering Java switch expressions and point out some common pitfalls to watch for.
Understanding Java Switch Expressions
Before diving into pitfalls, let's clarify what switch expressions are and how they differ from traditional switch statements.
In a traditional switch statement, you must use the break
keyword to exit each case, which can lead to fall-through bugs if forgotten. Switch expressions, on the other hand, allow you to return a value directly and suppress fall-through behavior.
Basic Syntax
Here is the basic syntax of a switch expression:
String day = "Monday";
String typeOfDay = switch (day) {
case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday" -> "Weekday";
case "Saturday", "Sunday" -> "Weekend";
default -> "Invalid day";
};
System.out.println(typeOfDay); // Output: Weekday
Why Use Switch Expressions?
- Cleaner Code: By eliminating the need for break statements, switch expressions reduce boilerplate code.
- Enhanced Readability: The arrow (
->
) operator provides a clear syntax that can be read more easily. - Returning values: You can directly return values from each case, making it easier to work with.
Common Pitfalls
Despite the improvements with switch expressions, several common pitfalls can catch even seasoned developers off guard. Let's explore these pitfalls and how to avoid them.
1. Forgetting the Default Case
One of the fundamental best practices in any switch structure is to include a default case. Omitting it can result in unexpected behavior, especially when dealing with user input.
Avoiding the Pitfall:
Always ensure you account for any unexpected input.
String season = "Autumn";
String seasonType = switch (season) {
case "Spring" -> "Flowers bloom";
case "Summer" -> "It's hot!";
case "Winter" -> "It's cold!";
// Be sure to include a default option
default -> "Unknown season";
};
System.out.println(seasonType); // Output: Unknown season
2. Using Non-Exhaustive Patterns
With the introduction of switch expressions, one might assume that all possible inputs have been handled. However, if any case or the default is left out, it can lead to incomplete logic.
Avoiding the Pitfall:
Consider the potential values and ensure they are handled properly.
String fruit = "Mango";
String color = switch (fruit) {
case "Apple" -> "Red";
case "Banana" -> "Yellow";
// Missed case for Mango
default -> "Unknown color";
};
System.out.println(color); // Output: Unknown color
3. Case Duplication
Another common mistake is repeating case labels within a switch expression. This will result in a compilation error.
Avoiding the Pitfall:
You can group multiple cases using commas, streamlining your code.
String vehicleType = "Car";
String category = switch (vehicleType) {
case "Car", "Truck" -> "Land Vehicle";
case "Boat" -> "Water Vehicle";
case "Airplane" -> "Air Vehicle";
default -> "Unknown Vehicle";
};
System.out.println(category); // Output: Land Vehicle
4. Incorrect Use of Variable Scope
Variables declared inside a switch expression are scoped only to that expression. If you reference a variable declared outside, ensure it is appropriately handled within the cases.
Avoiding the Pitfall:
Be mindful of where you declare your variables.
int x = 10;
String result = switch (x) {
case 1 -> "One";
case 2 -> "Two";
case 10 -> {
// Correctly referenced the variable
yield "Ten";
}
default -> "Unknown";
};
System.out.println(result); // Output: Ten
5. Switch Expressions with Null Values
Handling null
can be tricky. A null value will throw a NullPointerException
if not appropriately managed.
Avoiding the Pitfall:
Include a check for null values, thus ensuring robustness.
String input = null;
String response = switch (input) {
case "yes" -> "Confirmed";
case "no" -> "Denied";
default -> {
if (input == null) {
yield "Input cannot be null";
}
yield "Unexpected input";
}
};
System.out.println(response); // Output: Input cannot be null
Performance Considerations
While the switch expression has many benefits, it's essential to use it wisely. Although performance isn't typically an issue since Java compiles switch cases efficiently, poorly constructed switch logic can lead to maintainability problems over time.
In Conclusion, Here is What Matters
Mastering Java switch expressions can significantly enhance your coding accuracy and efficiency. By avoiding common pitfalls such as forgetting default cases, using exhaustive patterns, or mismanaging variable scopes, you can make your code cleaner and more readable.
For more detailed information on Java's switch expressions, you can refer to the Official Java Documentation.
By honing your skills with switch expressions, you'll write code that is not just functional but elegant. As always, practice is key. Experiment with these structures in real projects, and watch as your proficiency burgeons! Happy coding!