Troubleshooting Common Issues with Apache Camel Wire Tap
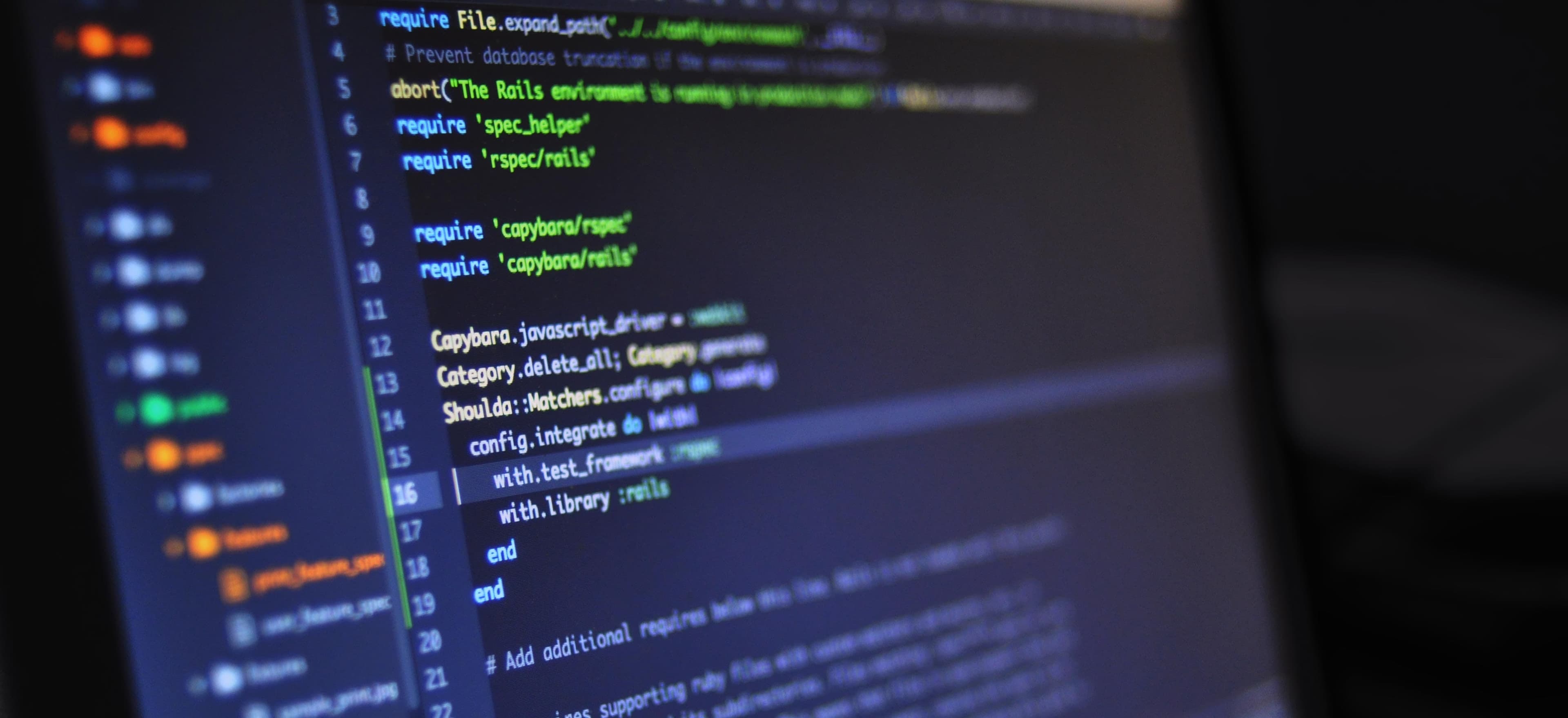
- Published on
Troubleshooting Common Issues with Apache Camel Wire Tap
Apache Camel is a powerful framework designed for integration patterns. One of its handy features, the Wire Tap, allows you to send a copy of an entire message to an endpoint while continuing the original flow seamlessly. However, like any tool, it can come with its own set of issues. In this blog post, we will dive deep into common problems encountered when using Apache Camel Wire Tap, including how to troubleshoot and resolve them effectively.
Understanding Wire Tap in Apache Camel
Before troubleshooting, let’s clarify what Apache Camel Wire Tap is and how it works. The Wire Tap pattern allows you to "tap into" a message route without interfering with its original processing.
Example of Wire Tap Usage
from("direct:start")
.wireTap("direct:additionalProcessing")
.to("log:mainFlow");
In this example, the message that arrives at the direct:start
endpoint is processed by the additionalProcessing
endpoint without disrupting the main route. This is particularly useful when you want to log messages, store them in a database, or perform analytics in parallel.
Common Issues and Their Troubleshooting Steps
1. Wire Tap Does Not Call the Endpoint
Issue: The Wire Tap might seem ineffective if the endpoint you are trying to tap into does not get invoked.
Solution:
- Check Endpoint URI: Verify that you have provided the correct URI in the
wireTap
method. Misspelled URIs can lead to the Wire Tap failing silently.
.wireTap("direct:validEndpoint") // Ensure this exists
- Validate Route Activation: Ensure that the route you are tapping into is active and correctly configured. Use
camel context.getRoutes()
to list all routes and their statuses.
2. Message is Not Reaching the Wire Tap
Issue: In some cases, messages may not be reaching the wire tap route due to conditions or filters.
Solution:
-
Remove Filters or Conditions: Check if there are filters (
filter
,choice
) that might prevent the message from reaching the wire tap. -
Inspect Log Levels: Enable debug logging within Camel to trace the message flow and identify where the message is being dropped.
log4j.logger.org.apache.camel=DEBUG
3. Performance Issues
Issue: In scenarios where the tapped endpoint is slow or resource-intensive, it can lead to performance bottlenecks.
Solution:
- Asynchronous Processing: Make sure the Wire Tap processes messages asynchronously. Adding
.async()
afterwireTap()
can help.
.wireTap("direct:slowService").async()
- Load Testing: Conduct load testing to identify areas where resources are strained. Apache JMeter or Gatling can assist in simulating and analyzing workload scenarios.
4. Failure on Wire Tap Endpoint
Issue: If your tapped endpoint fails, you may encounter exceptions that could halt the entire route.
Solution:
- Error Handling: Employ Camel’s error handling strategies, such as using Dead Letter Channels (DLC) to handle failures gracefully.
onException(Exception.class)
.to("log:error")
.end();
- Immediate Recovery: Consider adding a
retryDelay
within the endpoint URI:
.wireTap("direct:service?retryDelay=1000")
5. No Response from Wire Tapped Endpoint
Issue: You might notice that the Wire Tap is causing delays due to the endpoint not returning a response timely.
Solution:
- Configure Timeout: Implement timeout configuration on the wire tapped endpoint using Camel’s time-based settings.
.wireTap("direct:service?requestTimeout=2000")
- Monitor Response: Use a callback mechanism to log the responses for monitoring.
.wireTap("direct:service").to("log:tapResponse");
Testing Your Wire Tap Setup
Testing is critical to ensure your Wire Tap configuration works as expected. Create a simple JUnit test to validate your routes and Wire Tap functionality.
Example JUnit Test
@Test
public void testWireTap() throws Exception {
MockEndpoint mock = getMockEndpoint("mock:additionalProcessing");
mock.expectedMessageCount(1);
template.sendBody("direct:start", "Test Message");
mock.assertIsSatisfied();
}
In this test, we're ensuring that the Wire Tapped endpoint receives one message when a message is sent to the direct:start
.
Using Camel Test Kit
Integrate the Camel Test Kit for a more comprehensive testing strategy, which provides a robust way to test routes and behavior.
Wrap Up
The Wire Tap pattern in Apache Camel is an invaluable asset for asynchronously processing messages. By being aware of the common issues and employing effective troubleshooting measures, you can ensure optimal performance of your integration flows.
For comprehensive guidance on Apache Camel and its components, visit the Apache Camel Documentation. Don't forget to explore various other patterns that can enhance your integration strategies.
If you have additional questions or scenarios you’d like to discuss regarding Apache Camel or Wire Tap, feel free to leave comments below!
This blog post demonstrates essential troubleshooting techniques for Apache Camel Wire Tap, ensuring you can quickly get back to focusing on your integration work while effectively managing exceptions and performance issues. Happy coding!