Mastering Prepared Statements for JSON in PostgreSQL
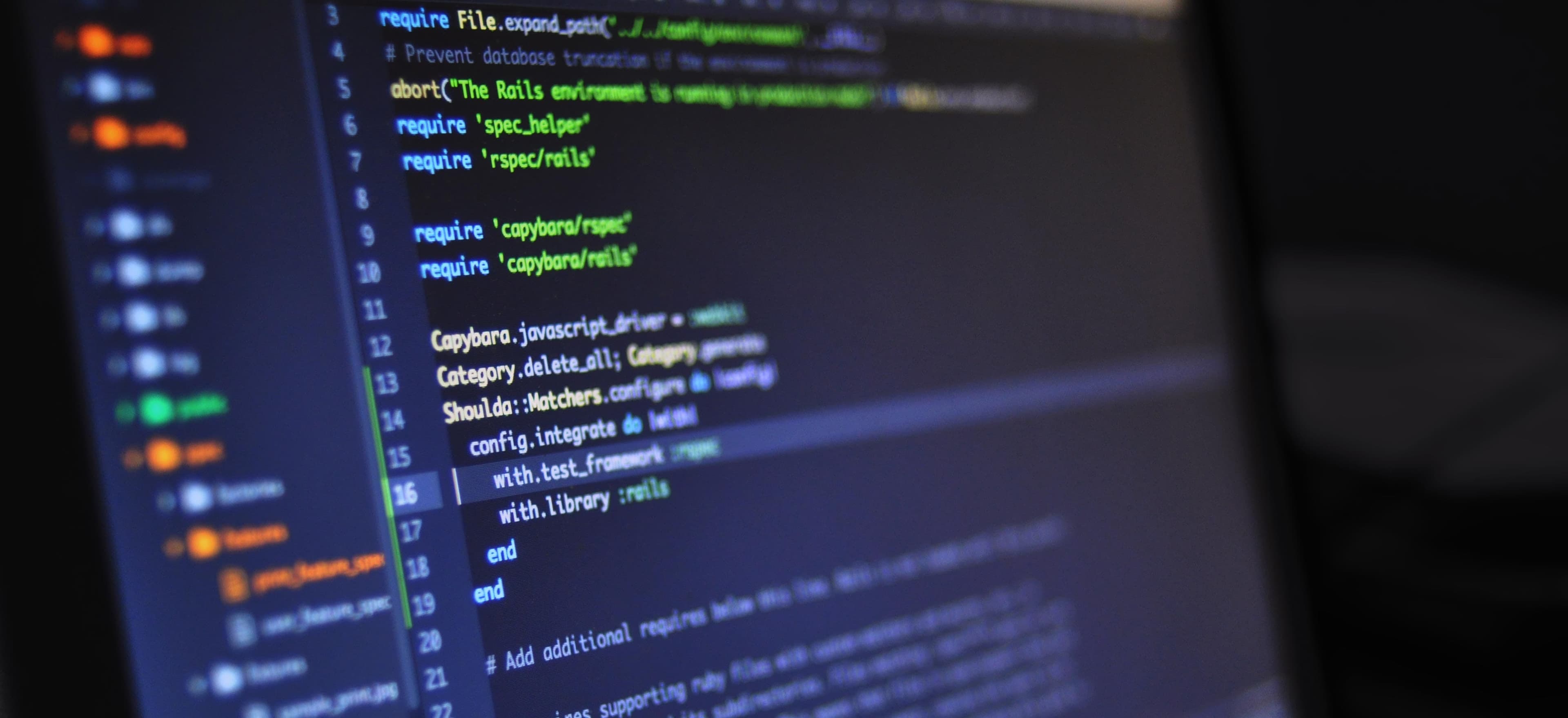
- Published on
Mastering Prepared Statements for JSON in PostgreSQL
When working with databases, performance and security are essential considerations. PostgreSQL, with its robust support for JSON data types, allows for powerful and flexible data manipulation. However, it’s crucial to handle JSON data securely to prevent SQL injection attacks. This is where prepared statements come into play. This blog post will guide you through the process of mastering prepared statements for JSON in PostgreSQL, including why and how to use them effectively.
What Are Prepared Statements?
Prepared statements are a feature provided by many SQL databases, including PostgreSQL, that allow you to execute the same query multiple times efficiently and securely. They work by separating the query structure from the data being inserted into the query. This not only enhances performance but also minimizes the risk of SQL injection attacks.
Why Use Prepared Statements?
- Security: They prevent SQL injection by treating user input as parameters rather than executable SQL code.
- Performance: Prepared statements can be compiled and optimized once and executed multiple times, reducing the overhead of parsing the SQL statement each time.
- Maintainability: Prepared statements make your code cleaner and easier to read, especially when dealing with complex queries.
Understanding JSON in PostgreSQL
PostgreSQL offers powerful support for JSON data types, allowing you to store and manipulate JSON data directly in your tables. There are two JSON types in PostgreSQL:
- json: Stores the JSON data as text.
- jsonb: Stores the JSON data in a binary format for faster processing.
Using jsonb
is generally recommended due to its improved performance, especially for query processing.
How to Use Prepared Statements with JSON
Here, we will demonstrate how to create and execute prepared statements in PostgreSQL that utilize JSON data. We'll focus on insertion and retrieval of JSON data.
Step 1: Creating a Sample Table
First, let’s create a sample table that stores JSON data. This will be our working environment.
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name TEXT NOT NULL,
details JSONB NOT NULL
);
Step 2: Inserting Data Using Prepared Statements
Now, let’s insert data into the users
table using a prepared statement. We will use a JSON object that represents user details.
Example Code: Inserting with Prepared Statements
-- Prepare the statement
PREPARE insert_user AS
INSERT INTO users (name, details) VALUES ($1, $2);
-- Execute the prepared statement
EXECUTE insert_user('John Doe', '{"age": 30, "email": "john.doe@example.com", "active": true}');
EXECUTE insert_user('Jane Smith', '{"age": 25, "email": "jane.smith@example.com", "active": false}');
Why This Works:
- The
PREPARE
command creates a template for the SQL statement, allowing you to specify placeholder parameters ($1
,$2
) instead of hardcoding values. - The
EXECUTE
command runs the prepared statement with actual values. This keeps the data separate from the query structure, enhancing security against SQL injections.
Step 3: Retrieving Data from JSON Columns
Once you have your data inserted, you’ll likely want to retrieve it using JSON operators. You can query JSON data directly with appropriate SQL commands.
Example Code: Querying JSON Data
-- Query all users with active status
PREPARE get_active_users AS
SELECT * FROM users WHERE details->>'active' = 'true';
-- Execute the query
EXECUTE get_active_users;
Why This Matters:
- The
->>
operator retrieves the value of the specified key as text. In this case, we’re checking if theactive
key in thedetails
JSONB column is set totrue
. - Prepared statements here enhance security as we separate the SQL structure from the values.
Step 4: Updating JSON Data
Preparing statements are not limited to retrieval and insertion; they can also be used for updating records. In PostgreSQL, you can update JSON fields with new values using the jsonb_set
function.
Example Code: Updating JSON Data
-- Prepare the statement for updating user details
PREPARE update_user_email AS
UPDATE users SET details = jsonb_set(details, '{email}', '"new.email@example.com"', false) WHERE name = $1;
-- Execute the prepared statement
EXECUTE update_user_email('John Doe');
Why This Is Important:
- The
jsonb_set
function allows you to update specific keys within JSONB objects while maintaining the rest of the structure intact. - Using prepared statements for updates ensures that your SQL is secure while also allowing for efficient changes to be made.
Step 5: Deleting Data
Lastly, let’s see how to delete records based on JSON criteria, again using prepared statements.
Example Code: Deleting Data
-- Prepare a statement to delete inactive users
PREPARE delete_inactive_users AS
DELETE FROM users WHERE details->>'active' = 'false';
-- Execute the prepared statement
EXECUTE delete_inactive_users;
Why This Is Effective:
- Just like before, this keeps the SQL query clean and reduces the risk associated with user input, eliminating SQL injection risks considerably.
- Deleting inactive users from the database ensures your data remains current and relevant without manual checks.
Key Takeaways
Mastering prepared statements for JSON in PostgreSQL is a valuable skill that not only optimizes performance but also enhances the security of your applications. By utilizing these techniques in your code, you can better handle JSON data types, keeping your database interactions efficient and safe.
Additional Resources
- PostgreSQL Documentation: Prepared Statements
- PostgreSQL Documentation: JSON Functions and Operators
- PostgreSQL JSONB Data Type
In conclusion, take the step toward secure and efficient database management with prepared statements in your PostgreSQL-driven applications. Whether you're developing web applications, microservices, or data processing pipelines, this knowledge will significantly benefit your projects. Happy coding!
Checkout our other articles