Common Pitfalls When Deploying Spring Boot on Elastic Beanstalk
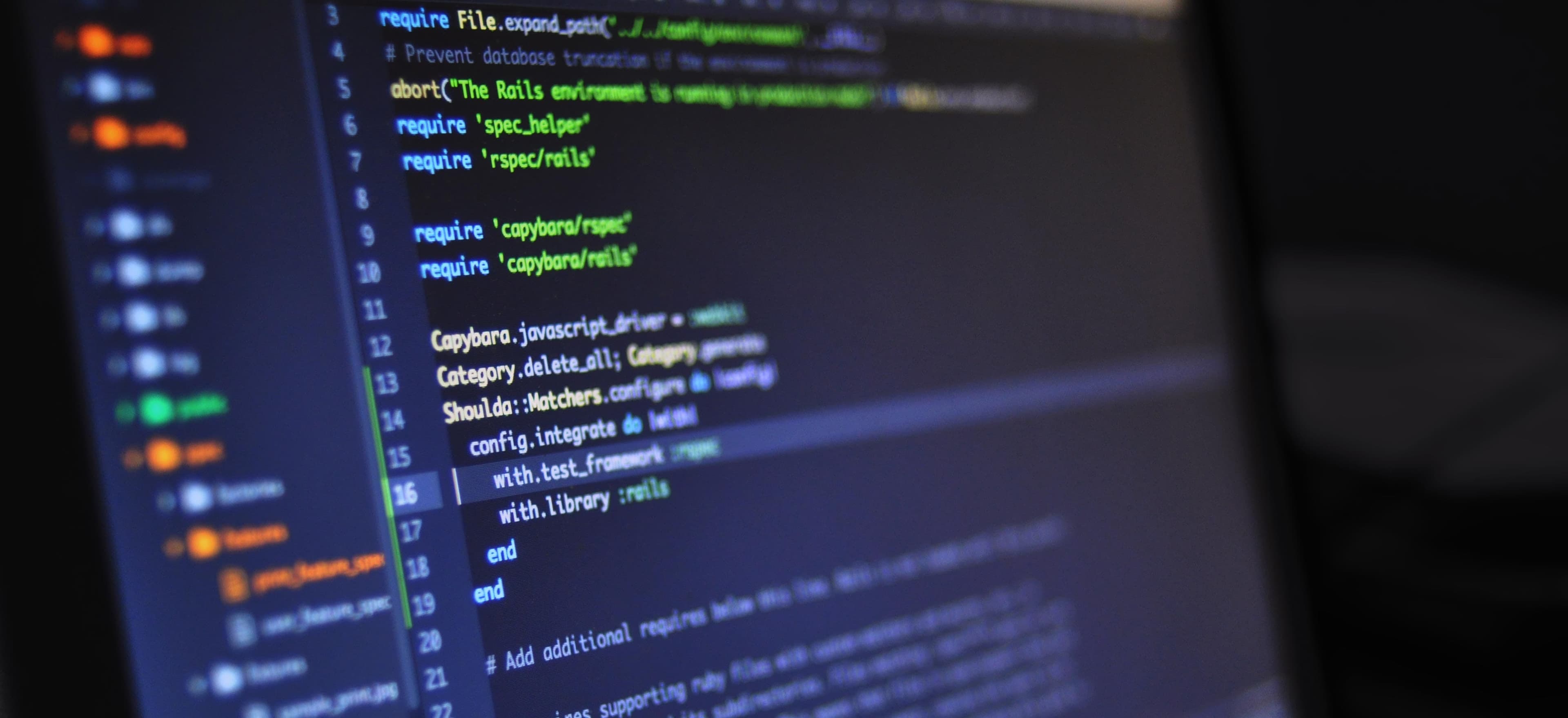
- Published on
Common Pitfalls When Deploying Spring Boot on Elastic Beanstalk
Deploying Spring Boot applications on AWS Elastic Beanstalk can be an exciting journey for any developer. While Elastic Beanstalk provides a streamlined way to manage applications in the cloud, there are several common pitfalls that can hinder a smooth deployment. In this blog post, we will dissect these pitfalls, share code snippets, and highlight their impacts on your application's performance, maintainability, and availability.
Table of Contents
- Understanding AWS Elastic Beanstalk
- Pitfall 1: Ignoring the Environment Configuration
- Pitfall 2: Not Configuring the Database Properly
- Pitfall 3: Overlooking Logging and Monitoring
- Pitfall 4: Handling Application Secrets Poorly
- Pitfall 5: Neglecting Custom Domain Configuration
- Conclusion
Understanding AWS Elastic Beanstalk
AWS Elastic Beanstalk is a Platform as a Service (PaaS) that simplifies the deployment and scaling of web applications. It abstracts the infrastructure and provisioning tasks while allowing you to manage your application environment. However, understanding how Elastic Beanstalk works is vital to make the most of it. You can find more on its functionality in the AWS Elastic Beanstalk documentation.
Pitfall 1: Ignoring the Environment Configuration
One of the most common mistakes is ignoring the environment configuration options. Elastic Beanstalk provides options for environment variables that can drastically alter the behavior of your application.
Example
Here is an example of how to set environment variables in your application.properties
:
server.port=${PORT:8080}
spring.datasource.url=${DB_URL}
spring.datasource.username=${DB_USER}
spring.datasource.password=${DB_PASSWORD}
Why?
The ${PORT:8080}
syntax allows your app to read the port assigned by Elastic Beanstalk, ensuring that your application runs correctly in the environment. Skipping this can lead to your application not binding to the correct port or database parameters not being recognized.
Solution
Ensure that your environment settings are carefully configured in the Elastic Beanstalk console under "Configuration" > "Software".
Pitfall 2: Not Configuring the Database Properly
Another frequent oversight is in configuring the database connection. Many developers hard-code database credentials or skip setting up RDS (Amazon Relational Database Service) altogether.
Example
To configure in an Amazon RDS instance, your application.properties
might look like this:
spring.datasource.url=jdbc:mysql://${DB_HOST}:${DB_PORT}/${DB_NAME}
spring.datasource.username=${DB_USER}
spring.datasource.password=${DB_PASSWORD}
Why?
Hardcoding credentials poses a security risk and can lead to errors in targeting a production database. Moreover, improper configuration can lead to connection issues.
Solution
Utilize Elastic Beanstalk’s RDS integration. When creating an environment, click on "Add RDS" and fill out the necessary fields. This configuration will ensure the correct parameters are used in your application.
Pitfall 3: Overlooking Logging and Monitoring
Logging and monitoring are essential for diagnosing issues with your Spring Boot application. Unfortunately, many developers fail to configure these aspects appropriately.
Example
You might configure logging in your application.properties
like so:
logging.file.name=logs/spring-boot-application.log
logging.level.org.springframework=DEBUG
logging.level.root=INFO
Why?
Without proper logging, identifying the root cause of issues post-deployment becomes extremely difficult. Furthermore, monitoring the application can help catch performance bottlenecks before they affect users.
Solution
Consider integrating with AWS CloudWatch for logging and monitoring. This can be done by adding the AWS SDK dependencies and configuring your logging settings accordingly.
Pitfall 4: Handling Application Secrets Poorly
In the age of data breaches, improperly handling application secrets is one of the most critical issues. Hardcoding API keys, secrets, or any sensitive information in the codebase can lead to significant vulnerabilities.
Example
Instead of hardcoding secrets, use AWS Secrets Manager to manage sensitive information:
String secret = getSecret("my-secret-key"); // Fetch secret from AWS Secrets Manager
Why?
Hardcoding sensitive information violates security best practices. Additionally, treating these secrets responsibly ensures your application remains secure.
Solution
Use AWS's native services to manage secrets. AWS Secrets Manager or AWS Systems Manager Parameter Store are both good options. Learn more about them here.
Pitfall 5: Neglecting Custom Domain Configuration
Finally, many developers forget to configure a custom domain for their deployed Spring Boot application. While Elastic Beanstalk environments come with a default URL, customizing it enhances branding and user trust.
Example
To set a custom domain, you'll typically modify your Route 53 DNS settings:
- Create a CNAME record pointing to your Elastic Beanstalk environment URL.
- Add an alias for your domain.
Why?
Using a custom domain not only elevates your brand but also helps with SEO, which is crucial for visibility.
Solution
Consider utilizing AWS Route 53 for domain registration and DNS management. Refer to the AWS Route 53 documentation for more detailed instructions.
A Final Look
Deploying a Spring Boot application on AWS Elastic Beanstalk can significantly improve your development and deployment process. However, avoiding common pitfalls is crucial for ensuring a seamless experience. Always remember to configure your environments correctly, handle databases wisely, focus on logging and monitoring, manage secrets effectively, and set up a custom domain for a professional appearance.
By following the recommendations above, you can shortcut many of the headaches associated with deployments and maintain a healthy, efficient web application. For a deeper dive into deploying with AWS, check out resources like the Deployment Guide for Spring Boot on AWS.
Happy coding!
Checkout our other articles