Debugging Common Issues in Spring Boot with Micrometer
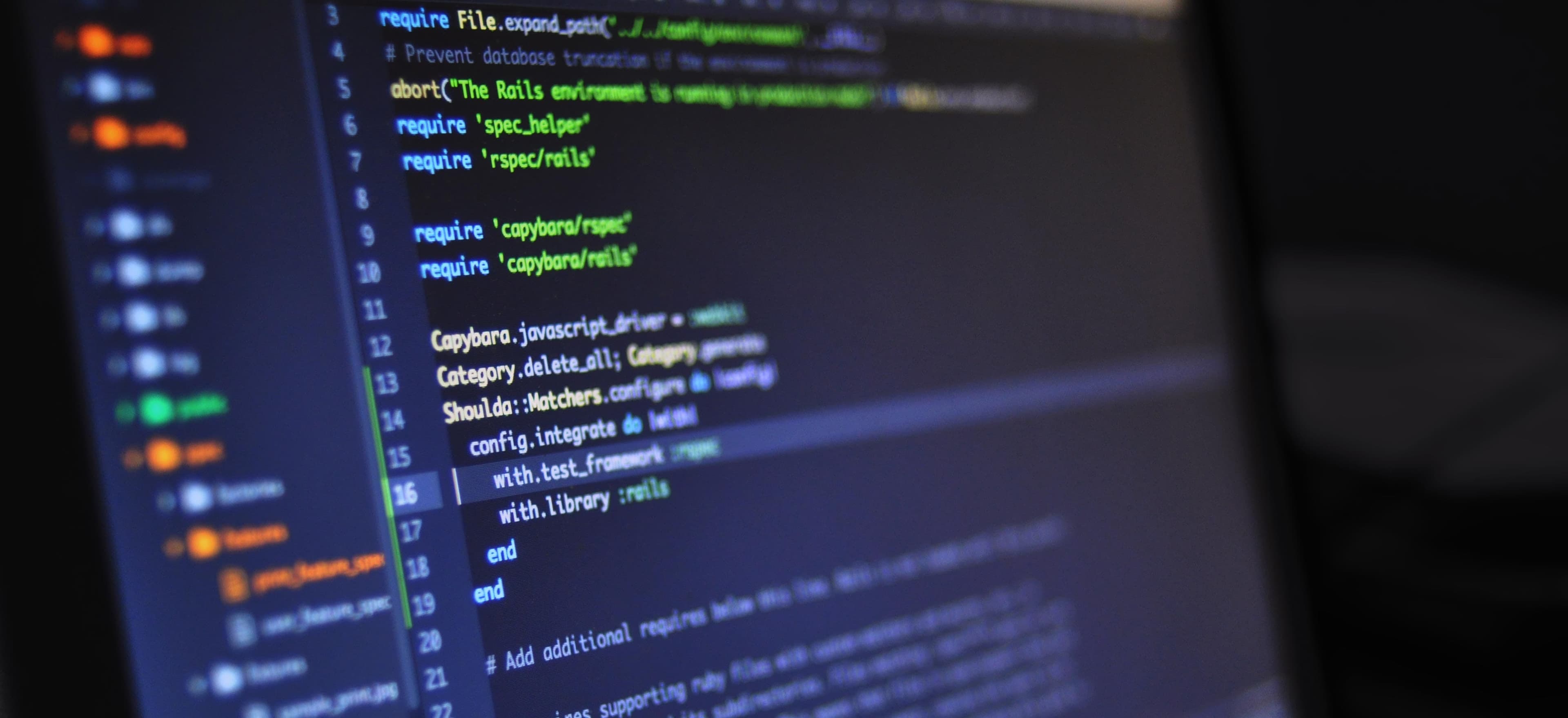
- Published on
Debugging Common Issues in Spring Boot with Micrometer
As modern applications grow in complexity, performance monitoring and debugging become crucial for maintaining service reliability. Spring Boot, one of the most popular frameworks for building Java applications, integrates seamlessly with Micrometer, a metrics collection library. This integration allows developers to track application performance and health metrics effectively. However, even with these tools at your disposal, issues still arise. This blog post will walk you through common problems you may encounter while using Spring Boot with Micrometer, along with strategies for debugging and resolving them.
Understanding Micrometer
Micrometer provides a facade for monitoring systems like Prometheus, CloudWatch, and others. It allows developers to capture metric data in their applications, enabling observability into performance metrics, error rates, and other key indicators.
In a Spring Boot application, you typically configure Micrometer by adding the necessary dependencies:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-core</artifactId>
</dependency>
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
With these dependencies included, you're ready to create and manage metrics.
Common Issues and Debugging Techniques
Let's explore some common issues developers face when integrating Micrometer with Spring Boot and how to debug them.
1. No Metrics Exported
Problem: Sometimes, you may notice that your metrics are not being exported correctly or at all.
Solution: Check your configuration files. Ensure that you're properly configuring Micrometer to publish metrics. Here’s how you can set it up in your application.properties
or application.yml
:
management:
endpoints:
web:
exposure:
include: '*'
metrics:
export:
prometheus:
enabled: true
This configuration allows all endpoints to be exposed and specifically enables Prometheus metrics export.
Also, verify your dependency management. You may be missing a required dependency for your Micrometer implementation.
2. Metrics Not Captured
Problem: You might set up a metric but notice that it isn’t capturing the desired data.
Solution: First, ensure that you are using the correct metric type. Micrometer supports different metric types such as Timer, Gauge, Counter, and Histogram.
Here is an example of how to use a Counter
to track user logins:
import io.micrometer.core.instrument.Counter;
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final Counter loginCounter;
public UserService(MeterRegistry meterRegistry) {
this.loginCounter = meterRegistry.counter("user.logins");
}
public void login(String username) {
// Your login logic here...
// Increment the login counter
loginCounter.increment();
}
}
In this example, every time a user logs in, the loginCounter
increments by 1. If you find that logins are not being counted, check to ensure that this method is being invoked correctly.
3. High Latency on Timer Metrics
Problem: Timer metrics may show unexpected high latency values.
Solution: This can happen if your method's execution is being influenced by external factors. To diagnose, consider wrapping your method within a Timer:
import io.micrometer.core.instrument.Timer;
import org.springframework.stereotype.Service;
@Service
public class OrderService {
private final Timer orderProcessingTimer;
public OrderService(MeterRegistry meterRegistry) {
this.orderProcessingTimer = meterRegistry.timer("order.processing");
}
public void processOrder(Order order) {
orderProcessingTimer.record(() -> {
// Simulate order processing logic
// Implement your logic here...
});
}
}
You can log the result and check if any external calls or database operations are causing delays.
4. Aggregated Metrics Not Reporting
Problem: You may find that your aggregated metrics (like sum or average) aren't reflecting the expected results.
Solution: Ensure your aggregation logic or the custom metrics setup is correct. For instance, if you’re creating a Gauge
, make sure it’s updated correctly:
import io.micrometer.core.instrument.Gauge;
import io.micrometer.core.instrument.MeterRegistry;
public class InventoryService {
private int itemsInStock;
public InventoryService(MeterRegistry meterRegistry) {
// Registering the Gauge with the current stock value
Gauge.builder("inventory.items_in_stock", this, InventoryService::getItemsInStock)
.register(meterRegistry);
}
public int getItemsInStock() {
return itemsInStock;
}
public void addItem() {
itemsInStock++;
}
public void removeItem() {
itemsInStock--;
}
}
In this snippet, the Gauges
dynamically update their value based on method callbacks. By registering the method getItemsInStock
, you can ensure that any changes to itemsInStock
get reflected in the metrics.
5. Prometheus Not Scraping Metrics
Problem: Even with everything set up correctly, Prometheus may not scrape the metrics from your Spring Boot app.
Solution: Check your Prometheus configuration file (prometheus.yml
). Make sure the scrape_config
section includes your Spring Boot app.
scrape_configs:
- job_name: 'spring-boot-app'
metrics_path: '/actuator/prometheus'
static_configs:
- targets: ['localhost:8080']
Verify that the metrics_path
points to the correct actuator endpoint. You can test this manually by visiting http://localhost:8080/actuator/prometheus
in your web browser. If you see the metrics, then Prometheus configuration is your next area to focus on.
6. Documentation and Support
When facing complex issues, don't hesitate to refer to the documentation. The Micrometer documentation is a valuable resource for understanding metrics types, nuances of metric collection, and APIs provided. For specific Spring Boot issues, the Spring Boot documentation is also essential.
Final Considerations
Monitoring your Spring Boot applications using Micrometer can vastly improve your performance insights and help you debug issues effectively. Understanding how to capture, register, and expose metrics can help you diagnose potential problems proactively.
By employing robust debugging techniques, from checking configurations, exploring metric implementations, to investigating Prometheus, you can ensure that your application maintains optimal performance. Remember that observability is a continuous process, and staying vigilant and informed boosts your application's reliability.
For more insights and Java best practices, consider checking out other resources like Java Brains or Baeldung. Happy coding!
Checkout our other articles