Common Pitfalls in JAX-WS with Spring and Maven
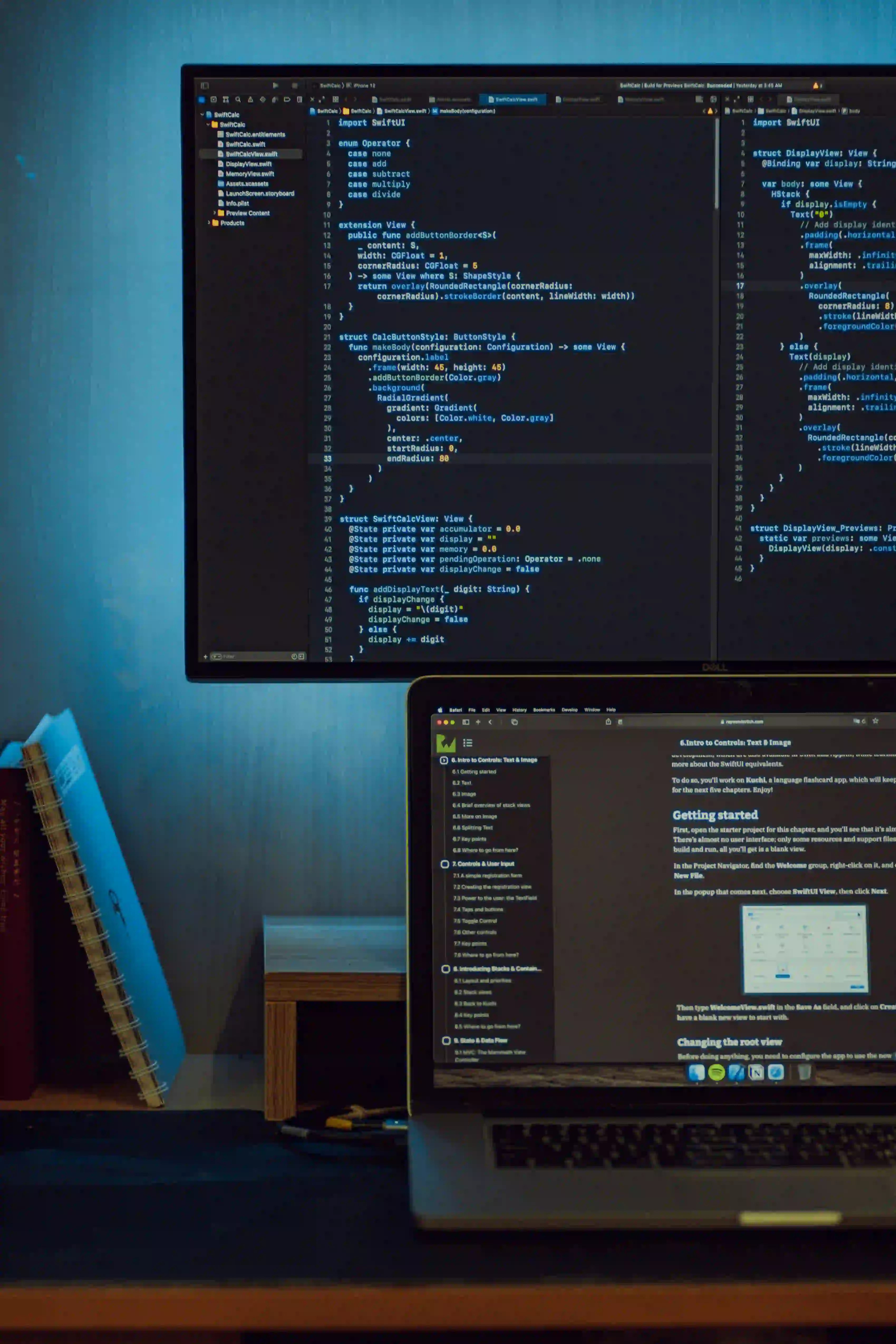
Common Pitfalls in JAX-WS with Spring and Maven
JAX-WS (Java API for XML Web Services) offers a robust framework for creating and consuming web services in Java. When combined with Spring and Maven, it can greatly enhance the efficiency, modularity, and maintainability of your web applications. However, developers can encounter specific pitfalls that might complicate the process. In this article, we'll explore these common pitfalls and provide solutions to ensure you have a smooth development experience.
Understanding the Basics
What is JAX-WS?
JAX-WS is an API for creating web services using XML. It allows developers to generate SOAP (Simple Object Access Protocol) web services from Java objects. These services can then be consumed by web clients, enabling seamless communication over the internet.
Spring Framework
Spring is a widely-used framework that simplifies Java application development. It provides support for various aspects of application building, from dependency injection to aspect-oriented programming.
Maven
Maven is a build automation tool primarily used for Java projects. It manages project dependencies and simplifies the build process.
The Intersection
When integrating JAX-WS with Spring and Maven, developers can leverage the benefits of these three tools to create comprehensive and efficient web services. However, some common pitfalls can arise.
Common Pitfalls
1. Incorrect Dependency Management
One frequent mistake is not managing Maven dependencies properly. JAX-WS has specific libraries that need to be included in your pom.xml
for proper functioning.
Solution: Ensure that your pom.xml
includes the necessary JAX-WS dependencies. Here’s an example of a minimal configuration:
<dependencies>
<dependency>
<groupId>javax.jws</groupId>
<artifactId>jaxws-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.internal.ws</groupId>
<artifactId>jaxws-rt</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.10</version>
</dependency>
</dependencies>
Why: Including the correct libraries ensures compatibility and prevents runtime errors related to missing classes.
2. Configuration Issues
Mismatches in configuration between Spring and JAX-WS often lead to issues. Spring requires specific configurations to recognize JAX-WS components.
Solution: Use Spring's @Endpoint
annotation to declare your web services. Here’s an example:
import javax.jws.WebMethod;
import javax.jws.WebService;
import org.springframework.stereotype.Service;
@WebService
@Service
public class HelloWorldService {
@WebMethod
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
Why: This structure integrates JAX-WS with Spring, allowing Spring to manage the lifecycle of the JAX-WS endpoint effectively.
3. Missing SOAP Configuration
Another common issue is improperly configuring the SOAP endpoint. If the endpoint isn’t defined correctly, clients won't be able to access the service.
Solution: Ensure your endpoint is specified in the applicationContext.xml
file or through Java Config. Here's how you can register your web service in XML:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<ws:servlet>
<ws:service name="helloWorldService" endpoint="http://localhost:8080/helloWorld" />
</ws:servlet>
<bean id="helloWorldService" class="com.example.HelloWorldService" />
</beans>
Why: This configuration establishes the endpoint that clients use to communicate with your web service.
4. Proper Exception Handling
Developers often overlook exception handling, which can lead to unhelpful error messages being sent back to clients.
Solution: Implement custom fault exceptions to handle errors gracefully.
@WebService
public class HelloWorldService {
@WebMethod
public String sayHello(String name) throws CustomException {
if (name == null) {
throw new CustomException("Name cannot be null");
}
return "Hello, " + name + "!";
}
@WebFault(name = "CustomException")
public class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
}
Why: This method ensures that clients receive meaningful error messages instead of a generic server error, aiding in debugging and improving user experience.
5. Inadequate Testing
Testing web services can be complex. Developers often neglect to test SOAP-based services thoroughly, leading to issues in production.
Solution: Use tools like SoapUI or Postman to test your web services. In addition, consider writing unit tests in your Java application:
import org.junit.Test;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import static org.junit.Assert.assertEquals;
@RunWith(SpringJUnit4ClassRunner.class)
public class HelloWorldServiceTest {
private HelloWorldService helloWorldService = new HelloWorldService();
@Test
public void testSayHello() {
String result = helloWorldService.sayHello("World");
assertEquals("Hello, World!", result);
}
}
Why: Unit tests can catch issues early in the development process, ensuring your services behave as expected.
6. Deployment Complexity
Deploying your application can be complex due to the various configurations and dependencies involved.
Solution: Use a continuous integration tool like Jenkins to automate your deployment process. Ensure all services are properly packaged in your .war
file.
Why: Automation decreases the chance of human error during deployment, leading to a smoother production environment.
7. Ignoring Performance Optimization
Performance can degrade if developers ignore optimal practices when using JAX-WS.
Solution: Implement caching strategies using Spring's caching abstraction. For example:
import org.springframework.cache.annotation.Cacheable;
import javax.jws.WebMethod;
import javax.jws.WebService;
@WebService
public class HelloWorldService {
@WebMethod
@Cacheable("helloCache")
public String sayHello(String name) {
// Simulate a slow service
simulateDelay();
return "Hello, " + name + "!";
}
private void simulateDelay() {
try {
Thread.sleep(2000); // Simulating delay
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Why: Caching minimizes the processing load on your service and improves response times for repeat requests.
Wrapping Up
Integrating JAX-WS with Spring and Maven can significantly enhance your web service capabilities. However, it is essential to be mindful of common pitfalls. By managing dependencies effectively, configuring services correctly, handling exceptions graciously, ensuring thorough testing, automating deployment, and optimizing performance, you can create robust web services that meet user demands.
For further reading, consider checking out the JAX-WS documentation and Spring Web Services project.
With these insights and solutions in hand, you can navigate the complexities of JAX-WS with confidence and ease. Happy coding!