Unlocking the Full Potential of Advanced ListenableFutures
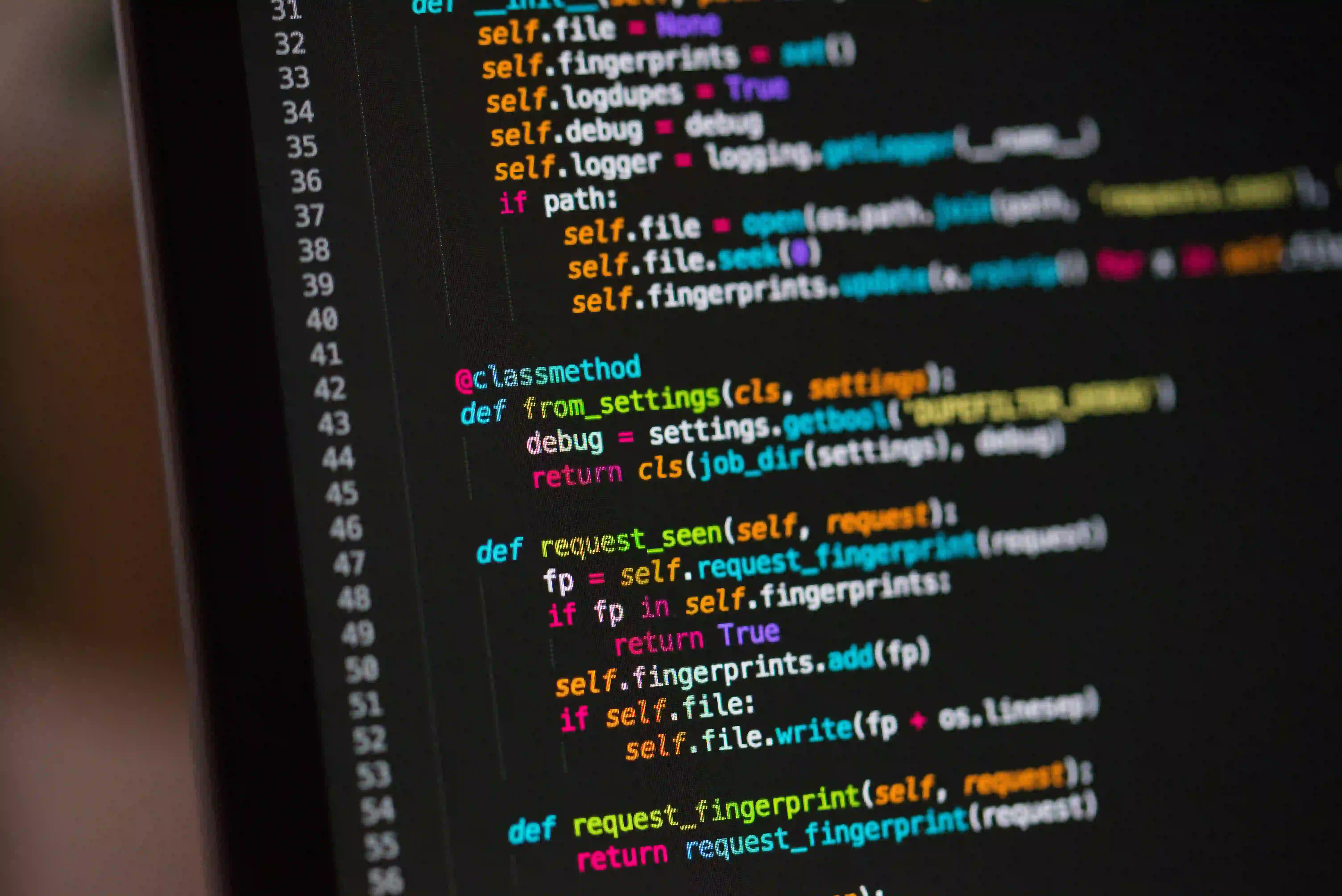
Unlocking the Full Potential of Advanced ListenableFutures in Java
As software development continues to evolve, asynchronous programming has become an essential part of creating responsive and efficient applications. One approach that has gained traction in the Java ecosystem is the use of ListenableFuture
, a powerful abstraction that greatly extends the capabilities of Java's built-in Future
. In this blog post, we will explore how to harness the full potential of ListenableFuture
, delve into its advanced features, and provide practical examples to illustrate its benefits.
What Are ListenableFutures?
ListenableFuture
is a part of the Guava library developed by Google. It is an extension of java.util.concurrent.Future
that provides a more flexible and powerful way to handle asynchronous tasks. Unlike standard Future
, which only provides a way to retrieve the result of an asynchronous computation, ListenableFuture
allows you to register listeners that get notified when the computation is complete. This enables more responsive applications, especially in environments where you need to handle multiple concurrent operations.
Why Use ListenableFutures?
- Asynchronous Handling: With
ListenableFuture
, you can execute tasks asynchronously without blocking the main thread. - Callbacks: You can add listeners that respond when tasks complete, giving you more control over task chaining and error handling.
- Flexibility: You can integrate
ListenableFuture
with other asynchronous patterns, such as CompletableFutures, making it easier to adopt modern Java features.
Getting Started with ListenableFutures
To begin using ListenableFuture
, you'll first need to include the Guava library in your project. If you are using Maven, add the following dependency:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>32.0.0-jre</version>
</dependency>
Creating a ListenableFuture
To demonstrate how to create a ListenableFuture
, let's consider a simple asynchronous task that simulates a long-running computation. Here’s how you can do it:
import com.google.common.util.concurrent.*;
import java.util.concurrent.Executors;
public class ListenableFutureExample {
private static final ListeningExecutorService executorService =
MoreExecutors.listeningDecorator(Executors.newFixedThreadPool(4));
public static void main(String[] args) {
ListenableFuture<String> future = executorService.submit(() -> {
Thread.sleep(2000); // Simulating long computation
return "Task Completed";
});
// Registering a callback using addListener
Futures.addCallback(future, new FutureCallback<String>() {
@Override
public void onSuccess(String result) {
System.out.println(result);
}
@Override
public void onFailure(Throwable t) {
System.err.println("Error: " + t.getMessage());
}
}, executorService);
}
}
Explanation
- ListeningExecutorService: We use
MoreExecutors.listeningDecorator()
to create aListeningExecutorService
, which allows us to submit tasks that returnListenableFuture
. - submit(): This method submits a Callable that contains the long-running operation.
- addCallback(): We register a callback to be executed upon the Future's completion. If it completes successfully,
onSuccess()
is called; if there is an error,onFailure()
is executed.
Benefits
This method of handling asynchronous tasks enhances code readability and maintainability as you can separate the logic of completing tasks from the work done once they finish.
Advanced Features of ListenableFutures
Chaining ListenableFutures
One of the standout features of ListenableFuture
is the ability to chain multiple asynchronous operations together. By using methods like Futures.transform()
, you can execute subsequent tasks based on the result of a previous task.
Here's an example:
ListenableFuture<Integer> firstFuture = executorService.submit(() -> {
Thread.sleep(1000); // Simulating delay
return 5;
});
ListenableFuture<String> transformedFuture = Futures.transform(firstFuture, input -> {
return "Transformed Result: " + (input * 2);
}, executorService);
Futures.addCallback(transformedFuture, new FutureCallback<String>() {
@Override
public void onSuccess(String result) {
System.out.println(result);
}
@Override
public void onFailure(Throwable t) {
System.err.println("Error: " + t.getMessage());
}
}, executorService);
Explanation
- Transforming the Result: The
Futures.transform()
function allows you to take the result fromfirstFuture
and apply a transformation to generate a newListenableFuture
. - Chaining Operations: You can keep chaining operations in a clean and understandable manner without nesting callback logic.
Handling Multiple Futures
If you have multiple ListenableFutures
that need to be executed in parallel, you can combine them using Futures.whenAllComplete()
. This is useful when you want to perform an action once all futures are complete.
Here’s how:
ListenableFuture<Integer> future1 = executorService.submit(() -> {
Thread.sleep(1000);
return 1;
});
ListenableFuture<Integer> future2 = executorService.submit(() -> {
Thread.sleep(1500);
return 2;
});
ListenableFuture<List<Integer>> allDone = Futures.successfulAsList(future1, future2);
Futures.addCallback(allDone, new FutureCallback<List<Integer>>() {
@Override
public void onSuccess(List<Integer> result) {
int sum = result.stream().mapToInt(Integer::intValue).sum();
System.out.println("Sum: " + sum);
}
@Override
public void onFailure(Throwable t) {
System.err.println("Error: " + t.getMessage());
}
}, executorService);
Explanation
- successfulAsList(): This method creates a new future that completes successfully with a list of results when all of the provided futures complete.
- Calculating the Sum: In the callback, we can aggregate results from multiple futures.
Best Practices for Using ListenableFutures
- Use Proper Exception Handling: Always ensure that you handle exceptions in your callbacks to prevent unhandled exceptions from crashing your application.
- Avoid Blocking: Aim to handle all tasks asynchronously. If a task blocks, it'll defeat the purpose of using
ListenableFuture
. - Monitor Executor Services: Always shut down your
ExecutorService
when it’s no longer needed to prevent memory leaks.
Lessons Learned
ListenableFuture
is a powerful tool in the Java programmer's toolkit, enabling more responsive, scalable applications. By allowing you to register callbacks, chain operations, and manage multiple futures, it streamlines asynchronous programming while also keeping the codebase clean and manageable.
To dive deeper into asynchronous programming in Java, consider exploring the Java Concurrency in Practice book, which provides comprehensive insights and best practices for managing concurrency in Java applications.
With ListenableFuture
at your disposal, you can unlock the full potential of asynchronous programming in Java. Happy coding!