Mastering Gson: Exclude Fields Without Breaking Your Code
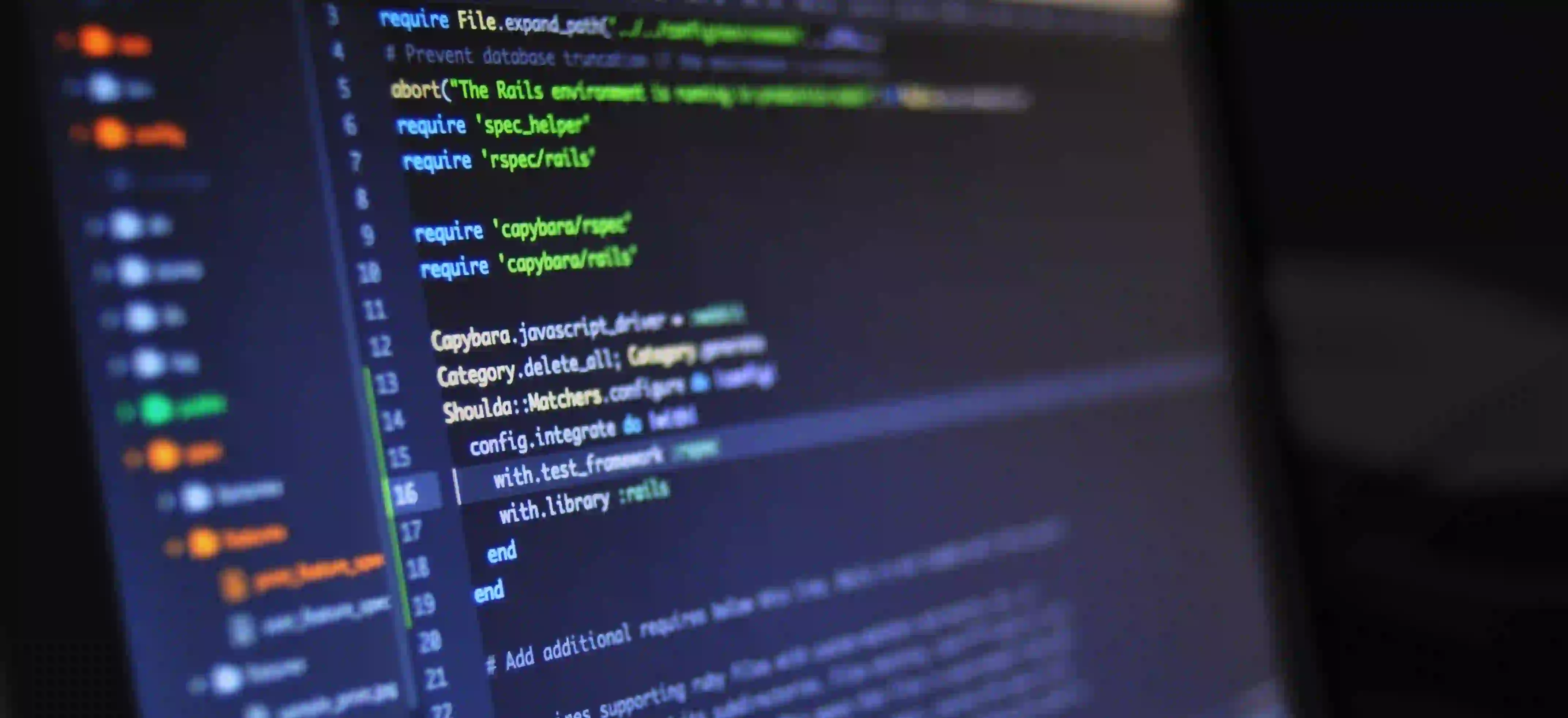
Mastering Gson: Exclude Fields Without Breaking Your Code
In today's world of Java development, data interchange between applications is a critical aspect. JSON, being lightweight and easy to understand, is the preferred format for this exchange. When dealing with JSON in Java, Gson, a popular library from Google, stands out for its simplicity and ease of use. However, as we design our data models, we often encounter the need to exclude certain fields from the JSON output without causing disruptions in our code. In this guide, we'll explore how to achieve this effectively.
What is Gson?
Gson is a Java library developed by Google that allows developers to convert Java objects into their JSON representation and vice versa. It provides flexible ways to control the serialization and deserialization process, empowering developers to handle their data models efficiently.
You can find the Gson library on GitHub for more information.
Why Exclude Fields?
Excluding fields can be essential for various reasons:
- Privacy: Sensitive data, such as passwords or personal identification numbers, should never be exposed in JSON responses.
- Performance: Sending unnecessary data over the network can slow down your application, especially if you're working with large data sets.
- Backward Compatibility: You might want to avoid breaking changes when updating your API or data structures.
- Simplification: For certain API endpoints, a simplified JSON schema may make sense, omitting complex data that is unnecessary for that particular context.
Now that we understand why excluding fields is important, let’s dive into how to use Gson to perform this task seamlessly.
Setting Up Your Project
First, ensure that you have Gson added to your project. If you are using Maven, include the following dependency in your pom.xml
:
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.10.1</version>
</dependency>
For Gradle users, add this line to your build.gradle
:
implementation 'com.google.code.gson:gson:2.10.1'
Basic Serialization With Gson
Before excluding fields, let's recap basic serialization with Gson:
import com.google.gson.Gson;
public class User {
private String username;
private String email;
private String password; // This will be excluded
public User(String username, String email, String password) {
this.username = username;
this.email = email;
this.password = password;
}
}
Here's how we convert a User
object into a JSON string:
public static void main(String[] args) {
Gson gson = new Gson();
User user = new User("john_doe", "john@example.com", "secret_password");
String json = gson.toJson(user);
System.out.println(json);
}
Output:
{"username":"john_doe","email":"john@example.com","password":"secret_password"}
As demonstrated above, every field—including sensitive information like password
—is serialized.
Excluding Fields with @Expose Annotation
Gson allows you to control which fields are serialized or deserialized using the @Expose
annotation. By default, Gson will serialize all fields unless specified otherwise.
- Mark Fields with @Expose: Only fields marked with
@Expose
will be included in the JSON output.
Let's modify the User
class:
import com.google.gson.annotations.Expose;
public class User {
@Expose
private String username;
@Expose
private String email;
@Expose(serialize = false, deserialize = false) // Exclude password
private String password;
public User(String username, String email, String password) {
this.username = username;
this.email = email;
this.password = password;
}
}
Now, when we serialize the User
object:
public static void main(String[] args) {
Gson gson = new GsonBuilder().excludeFieldsWithoutExposeAnnotation().create();
User user = new User("john_doe", "john@example.com", "secret_password");
String json = gson.toJson(user);
System.out.println(json);
}
Output:
{"username":"john_doe","email":"john@example.com"}
Why Use @Expose?
Using @Expose
gives you fine-grained control over your object's serialization and deserialization. You eliminate the risk of unintentionally exposing sensitive information while also retaining the flexibility needed for various data representations.
Excluding Fields Conditionally
Sometimes, you might want to exclude fields based on certain conditions. One way to achieve this is via custom serialization by implementing the JsonSerializer
interface.
Implementing Custom Serialization
Here's how you can implement it:
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonSerializationContext;
import com.google.gson.JsonSerializer;
import java.lang.reflect.Type;
public class UserSerializer implements JsonSerializer<User> {
@Override
public JsonElement serialize(User user, Type typeOfSrc, JsonSerializationContext context) {
JsonObject jsonObject = new JsonObject();
jsonObject.addProperty("username", user.getUsername());
jsonObject.addProperty("email", user.getEmail());
// Password will be excluded based on your condition.
return jsonObject;
}
}
You can then register this serializer:
public static void main(String[] args) {
Gson gson = new GsonBuilder()
.registerTypeAdapter(User.class, new UserSerializer())
.create();
User user = new User("john_doe", "john@example.com", "secret_password");
String json = gson.toJson(user);
System.out.println(json);
}
Why Use Custom Serialization?
Custom serialization provides maximum flexibility. When different use cases require various fields to be included or excluded dynamically, custom logic can facilitate this process without cluttering your model with annotations.
Excluding Fields with Transient Modifier
Another approach to exclude fields without using Gson annotations is to use the transient
modifier on your class fields. Fields marked as transient
are skipped during serialization.
public class User {
private String username;
private String email;
private transient String password; // Automatically excluded
// Constructor and getters
}
Using transient
is a straightforward method; however, it may not always be ideal, especially if you still need those fields for other purposes.
Summary
In conclusion, Gson provides multiple ways to exclude fields from JSON serialization effectively. We covered several methods, including:
- Using the
@Expose
annotation - Implementing custom serialization
- Utilizing the
transient
modifier
Each method has its strengths, allowing developers to choose the most appropriate approach for their requirements.
For further reading on Gson and advanced configurations, check out the Gson User Guide.
By mastering these techniques, you can manage your data interchange with precision and control while maintaining the integrity and performance of your Java applications.