Streamline Your Tomcat Installation with FTP and Git
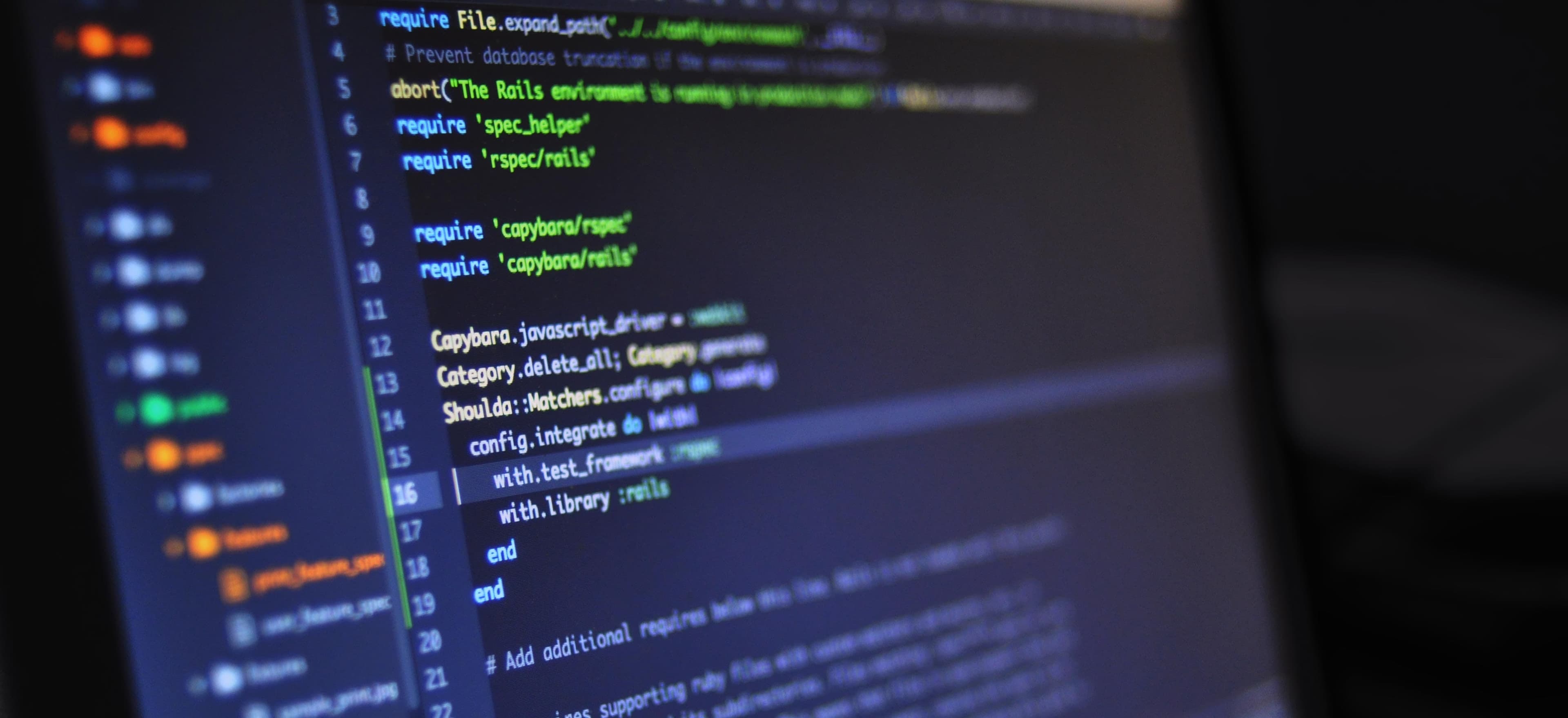
- Published on
Streamline Your Tomcat Installation with FTP and Git
Setting up a web application environment can be a tedious task. However, with the right tools, we can streamline the deployment process significantly. In this post, we will explore how to use FTP and Git to enhance your Tomcat installation. By automating the deployment process, you can focus more on coding and less on configuration.
Table of Contents
- Understanding Tomcat
- Why Use FTP for Tomcat Deployment?
- Why Use Git for Version Control?
- Setting Up Your Environment
- Step-by-Step Deployment Process
- Final Thoughts
Understanding Tomcat
Apache Tomcat is an open-source implementation of the Java Servlet, JavaServer Pages, and Java Expression Language technologies. Essentially, it provides a platform to deploy Java-based web applications, serving millions of users. One of its biggest advantages is its flexibility and ease of integration with other tools.
Why Use FTP for Tomcat Deployment?
File Transfer Protocol (FTP) simplifies the process of uploading files to your Tomcat server. While there are various methods for deployment (like manual uploads or scripts), FTP is a direct and user-friendly way to transfer files.
Benefits of Using FTP:
- Easy Management: Uploading and managing files can be done quickly through an FTP client.
- No Additional Commands: FTP eliminates the need to run additional command-line instructions to push updates.
- Supporting Remote Teams: FTP clients are widely understood, making it easier for teams to collaborate on file uploads.
Why Use Git for Version Control?
Git is essential for tracking changes in your codebase. With Git, you maintain a history of your development, making it easy to rollback or branch out new features without losing work.
Benefits of Using Git:
- Version History: Keep a comprehensive log of all code changes made.
- Collaboration: Team members can work on parallel features without clashes, using branches effectively.
- Easier Rollbacks: If something goes wrong, reverting back to previous versions is straightforward.
Setting Up Your Environment
Before we dive into the deployment specifics, let's set up our environment. You will need:
- A Tomcat server installed (look at the Tomcat Installation guide).
- An FTP client like FileZilla or WinSCP installed.
- A Git client installed on your machine. You can use the command line or GUI clients like GitHub Desktop.
Once these tools are set up, you're ready to streamline your deployment process.
Step-by-Step Deployment Process
Step 1: Create Your Java Web Application
First, ensure you have a Java web application ready to deploy. For the sake of this example, let's create a simple "Hello World" application.
package com.example;
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
public class HelloWorldServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
response.getWriter().println("<h2>Hello, World!</h2>");
}
}
Step 2: Package as a WAR File
You'll need to package your application into a war (Web Application Archive) file. This is how Tomcat deploys applications. Here's a simple command in Maven that you can use from the terminal:
mvn clean package
This command packages your application into a target/yourapp.war
file.
Step 3: Initialize a Git Repository
Create a new Git repository for your project. Navigate to your project's root directory in the terminal:
git init
git add .
git commit -m "Initial commit: Creating a Hello World application."
Step 4: Set Up FTP for Deployment
In your FTP client, connect to your Tomcat server. You will need the following information:
- Host: IP address of your server
- Username: Your FTP username
- Password: Your FTP password
Once connected, navigate to the webapps
folder in your Tomcat installation.
Step 5: Upload the WAR File
Using your FTP client, upload the WAR file that you just generated to the webapps
folder on your Tomcat server. Tomcat will automatically recognize the newly uploaded WAR file and deploy it.
Step 6: Verify the Deployment
To verify that your application is up and running, open your web browser and enter:
http://<your-ip-address>:8080/yourapp
You should see the message "Hello, World!" displayed.
Step 7: Automate with Scripts (Optional)
You can write a shell script to automate the FTP upload process and make it more efficient:
#!/bin/bash
# Variables
HOST='your.host.com'
USER='your_username'
PASS='your_password'
WAR_FILE='target/yourapp.war'
REMOTE_DIR='/path/to/tomcat/webapps/'
# Uploading WAR file using FTP
ftp -inv $HOST <<EOF
user $USER $PASS
put $WAR_FILE $REMOTE_DIR
bye
EOF
echo "Deployment complete!"
Step 8: Use Git for Continuous Deployment
To take it a step further, you can automate this process using Git hooks. After a successful commit, a post-commit hook can be set up to run your script and deploy the latest changes to Tomcat automatically.
You can create a post-commit hook like this:
#!/bin/sh
# Navigate to your project directory
cd /path/to/your/project
# Run your deployment script
sh deploy.sh
Make sure to give the hook file executable permissions:
chmod +x .git/hooks/post-commit
Final Thoughts
Streamlining your Tomcat installation using FTP and Git can save you countless hours and simplify the deployment process. Automating your deployments allows you to dedicate your energy to developing new features rather than configuring servers.
Remember, practice good coding conventions and make use of Git features like branching and pull requests to elegantly manage your development cycle.
If you're looking to dive deeper into other deployment methods or advanced Git usage, check out Git Documentation and Tomcat Deployment Documentation.
By following these steps, you will greatly enhance your productivity and maintain a smoother workflow for your Java applications. Happy coding and deploying!
Checkout our other articles